Python, a versatile programming language, offers various methods for embedding variables within strings. This article explores the key techniques of how to put a variable in a string in Python, ensuring that even beginners can grasp these concepts with ease.
Basic Concatenation: The Plus (+) Operator
At the foundation of string manipulation in Python lies the venerable plus (+) operator. It is one of the simplest ways to integrate a variable into a string. This method involves directly combining a string and a variable:
name = “Alice” greeting = “Hello, ” + name + “!” print(greeting) # Output: Hello, Alice! |
Points to Note:
- Always ensure that the variable is a string; otherwise, Python will raise an error;
- For non-string variables, use the str() function to convert them to strings.
The plus operator is a straightforward approach that serves well for basic string composition.
String Formatting with the Percent (%) Operator
Before the era of F-strings, Python relied heavily on the percent (%) operator, also known as the string formatting or interpolation operator, to incorporate variables into strings. This operator offers a concise and convenient syntax:
name = “Bob” greeting = “Hello, %s!” % name print(greeting) # Output: Hello, Bob! |
Usage Guide:
- %s is used to insert strings;
- %d for integers;
- %f for floating-point numbers.
While this method may be considered somewhat dated, it remains a viable option for many string formatting tasks, especially in legacy code.
Advanced String Formatting with str.format()
For developers seeking enhanced control over formatting, the str.format() method is a versatile choice. This method enables you to insert variables into strings while providing greater flexibility:
name = “Charlie” age = 30 greeting = “Hello, {}. You are {} years old.”.format(name, age) print(greeting) # Output: Hello, Charlie. You are 30 years old. |
Advantages:
- Facilitates easy insertion of multiple variables;
- Offers fine-grained control over formatting, making it suitable for complex string compositions.
str.format() shines when you require precise control over how variables are incorporated into your strings.
F-Strings: A Modern Approach
Introduced in Python 3.6, F-strings have revolutionized the way variables are inserted into strings. These formatted string literals offer a concise and readable approach:
name = “Diana” greeting = f”Hello, {name}!” print(greeting) # Output: Hello, Diana! |
Key Features:
- Preface the string with f or F to indicate an F-string;
- Place variables directly within curly braces {}.
F-strings are not only concise but also versatile, allowing you to embed expressions and even call functions directly within the braces.
Expression Embedding in F-Strings
One of the standout features of F-strings is their ability to seamlessly embed expressions within the string:
a = 5 b = 10 result = f”The sum of {a} and {b} is {a + b}.” print(result) # Output: The sum of 5 and 10 is 15. |
F-strings, with their simplicity and power, have become the preferred choice for many Python developers, especially in modern codebases.
Handling Special Characters
In the realm of string manipulation, you may encounter special characters such as quotes or backslashes. To correctly include variables containing these characters in a string, Python provides escape sequences:
quote = “Python’s syntax is \”easy\” to learn.” print(quote) # Output: Python’s syntax is “easy” to learn. |
Understanding and utilizing escape sequences is essential when dealing with strings that contain special characters.
Handling Complex Data Structures
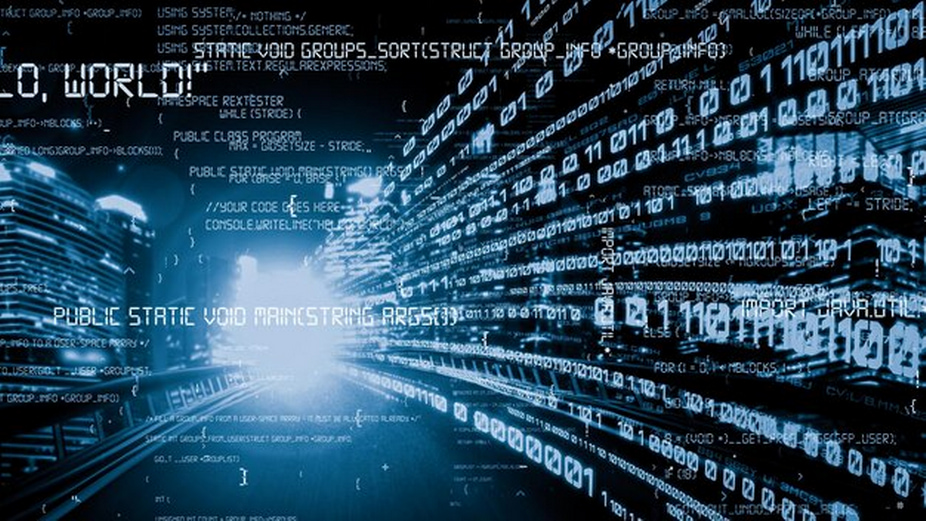
Python’s flexibility extends beyond simple variables. You can seamlessly incorporate variables from complex data structures like lists or dictionaries into your strings:
person = {“name”: “Eve”, “age”: 28} greeting = f”{person[‘name’]} is {person[‘age’]} years old.” print(greeting) # Output: Eve is 28 years old. |
Python’s ability to work with complex data structures within strings adds a layer of sophistication to your text output.
Combining Multiple Methods
As you progress in your Python journey, you’ll often encounter the need to insert variables into strings to create dynamic and customized text output. Python offers a versatile toolkit of methods to achieve this, allowing you to tailor your approach to your specific project requirements. Let’s explore these methods in detail:
String Concatenation
String concatenation involves using the + operator to combine strings and variables. For example:
name = “Alice” age = 30 message = “My name is ” + name + ” and I am ” + str(age) + ” years old.” |
This method is straightforward, but it requires explicit type conversion for non-string variables.
String Formatting with % Operator
The % operator allows you to create placeholders within a string and then provide values to replace those placeholders using the % operator:
name = “Bob” age = 25 message = “My name is %s and I am %d years old.” % (name, age) |
The %s and %d placeholders indicate the types of values to expect.
String Formatting with .format()
Python’s .format() method offers a more flexible and readable way to insert variables into strings. You use curly braces {} as placeholders and then call the .format() method to replace them:
name = “Charlie” age = 35 message = “My name is {} and I am {} years old.”.format(name, age) |
This method is more versatile and doesn’t require specifying value types explicitly.
F-Strings (Formatted String Literals)
Starting from Python 3.6, f-strings have gained popularity for their simplicity and readability. You can create them by prefixing a string with ‘f’ or ‘F’ and then embed variables directly within curly braces {}:
name = “David” age = 40 message = f”My name is {name} and I am {age} years old.” |
F-strings offer a concise and intuitive way to create formatted strings with variables.
String Templates
Python’s string.Template class provides a template-based approach for inserting variables into strings. You define placeholders using $ followed by the variable name and then substitute values using the .substitute() method:
from string import Template name = “Eve” age = 45 template = Template(“My name is $name and I am $age years old.”) message = template.substitute(name=name, age=age) |
This method is useful when you want to create reusable templates.
f-Strings with Expressions
F-strings allow you to include expressions within the curly braces, enabling more dynamic content:
x = 10 y = 5 result = f”The sum of {x} and {y} is {x + y}.” Here, the sum of x and y is calculated within the f-string and included in the string. |
Conclusion
Learning how to put a variable in a string in Python is a fundamental skill, essential for both simple and complex tasks. Whether you choose basic concatenation, the percent operator, str.format(), or the modern F-strings, each method offers unique advantages. By mastering these techniques, you enhance your Python programming efficiency and readability, unlocking the door to more advanced programming feats.
FAQ
Can I use F-strings with older versions of Python?
No, F-strings were introduced in Python 3.6. Use str.format() or the percent (%) operator for older versions.
How do I format a floating-point number in a string?
Use the str.format() method or F-strings with format specifiers like {:.2f} for two decimal places.
What is the most efficient method to put a variable in a string in Python?
F-strings are generally considered the most efficient and readable method.
Can I nest variables within variables in a string?
Yes, especially using F-strings or str.format(), you can nest variables for dynamic string composition.
How do I handle special characters in Python strings?
Use backslash (\) as an escape character for special characters like quotes or newlines.