Often, Python is a preferred language of programmers due to its simplicity and effectiveness. A very common application of it is to verify the existence of an item in the list. Knowing “how to check if something is in a list Python” can drastically change the way we go about efficient coding. In this article, we walk you through eight effective steps which include various methods and tips on how to code this task in Python.
Understanding Python Lists
To better understand how to check if something is in a list in Python, you must get a good understanding of Python lists. A list in Python is the most solid data structure that enables storing and conducting operations on data in the most efficient way. Here’s a detailed breakdown of Python lists:
Definition
A Python list is a collection that is:
- Ordered: Items in a list are stored in a specific sequence, and this sequence is maintained until the list is modified;
- Changeable: Lists are mutable, meaning you can modify, add, or remove elements after the list is created.
Example of a Python List
my_list = [1, 2, 3, 'apple', 'banana']
In the given case, the my_list list has both item types, integers (1, 2, 3) and strings (‘apple’, ‘banana’). While it is worth mentioning that Python lists can take up many data types like integers, strings, floats and even list objects, a Python dictionary is more preferable.
Properties of Python Lists
- Ordered Collection: Lists assure an inserting order for elements being listed. When you work on retrieving elements from a list it always will be in the same order they were added and it is possible to access them as in sequential order;
- Mutable: Lists are dynamic meaning that they can be modified after creation. By making use of these methods like adding elements, removing them, and perhaps changing the values associated with those elements, you can easily operate a list;
- Dynamic Size: In Python, lists cannot only grow or diminish in size, but also can perform this function dynamically. You concentrate on the actual contents of the list when not concerned with its size, and you can change the elements there at any time;
- Heterogeneous Elements: In python, elements of different data types can appear on lists. You can combine numbers (integers), strings, decimals, even other complicated objects within a single list.
Common Operations on Python Lists
Here are some common operations you can perform on Python lists:
Operation | Description |
---|---|
Accessing Elements | Retrieve individual elements or slices of elements |
Modifying Elements | Change the value of existing elements in the list |
Adding Elements | Append new elements to the end of the list |
Removing Elements | Remove elements from the list by value or index |
List Concatenation | Combine multiple lists into a single list |
List Slicing | Extract a subset of elements from a list |
List Iteration | Traverse through each element in the list using loops |
List Comprehension | Create new lists by applying expressions to existing lists |
Checking if Something is in a Python List
Now that you have a good understanding of Python lists, let’s discuss how to check if something is in a list in Python. The in keyword is used to determine whether a value exists within a list. Here’s how you can use it:
if 'apple' in my_list:
print("Yes, 'apple' is in the list.")
else:
print("No, 'apple' is not in the list.")
In this example, we check if the string ‘apple’ exists in the list my_list. If it does, the program prints a message confirming its presence; otherwise, it prints a message indicating that ‘apple’ is not in the list.
The ‘in’ Operator: Your First Tool
The ‘in’ operator is a powerful tool for checking the existence of an item within a list. It provides a simple and efficient way to perform membership tests. Let’s delve into the details of how the ‘in’ operator works and how you can leverage it effectively in your Python code.
Basic Syntax
The basic syntax of using the ‘in’ operator in Python is as follows:
if item in list:
# Do something
Here, item is the element you want to check for existence, and list is the list in which you want to perform the check. If item is found in list, the condition evaluates to True; otherwise, it evaluates to False.
Example Usage
Consider the following example:
my_list = ['apple', 'banana', 'orange']
if 'apple' in my_list:
print("Apple is in the list!")
In this code snippet, the ‘in’ operator checks if the string ‘apple’ exists in the list my_list. Since ‘apple’ is indeed present in my_list, the condition evaluates to True, and the message “Apple is in the list!” is printed.
Key Features
Here are some key features and considerations regarding the ‘in’ operator:
- Case Sensitivity: The ‘in’ operator is case-sensitive. For example, ‘Apple’ and ‘apple’ are treated as different items;
- Membership Test: The ‘in’ operator performs a membership test and returns a Boolean value (True or False) based on whether the item exists in the list or not;
- Efficiency: The ‘in’ operator is highly efficient, especially when working with large lists. It utilizes optimized algorithms for fast membership testing;
- Iterable Compatibility: Apart from lists, the ‘in’ operator can be used with other iterable data structures like tuples, sets, and dictionaries.
Advanced Usage
You can also use the ‘in’ operator in conjunction with conditional statements and loops for more advanced functionality. For example:
fruits = ['apple', 'banana', 'orange']
for fruit in fruits:
if 'a' in fruit:
print(fruit)
In this example, the ‘in’ operator is used within a loop to filter and print only those fruits that contain the letter ‘a’.
Using Loops for Checking
While the ‘in’ operator provides a straightforward way to check for the existence of an item in a list, there are scenarios where you might need to employ loops for more complex checks or additional operations. Let’s explore how you can use loops, specifically for loops, to achieve this in Python.
When to Use Loops
While the ‘in’ operator efficiently checks for the existence of an item in a list, using loops becomes necessary when:
- You need to perform additional operations or checks alongside the membership test;
- You want to iterate through the entire list to gather more information about the elements;
- The condition for checking membership is more complex than a simple equality comparison.
Using a for Loop
A common approach to check if something is in a list using loops is by iterating through each element of the list using a for loop. Here’s how you can accomplish this:
my_list = ['apple', 'banana', 'orange']
for item in my_list:
if item == 'banana':
print("Found banana!")
In this code snippet, the for loop iterates through each element (item) in the my_list. Within the loop, an if statement checks if the current item is equal to ‘banana’. If it is, a message “Found banana!” is printed.
Key Considerations
When using loops for checking membership in Python, keep the following considerations in mind:
- Iteration Overhead: Using loops for membership tests might introduce additional iteration overhead, especially for large lists, compared to the direct use of the ‘in’ operator;
- Complexity: If the membership check involves complex conditions or operations, using loops can offer more flexibility and control over the process;
- Performance: While loops provide flexibility, they may not always be the most efficient solution for simple membership tests. Consider the trade-offs between performance and flexibility based on your specific requirements.
Alternative Looping Techniques
Besides loops, Python offers other looping techniques such as while loops and list comprehensions, which can also be used for checking membership in lists. The choice of looping technique depends on the specific requirements and complexity of the task.
The Power of List Comprehension
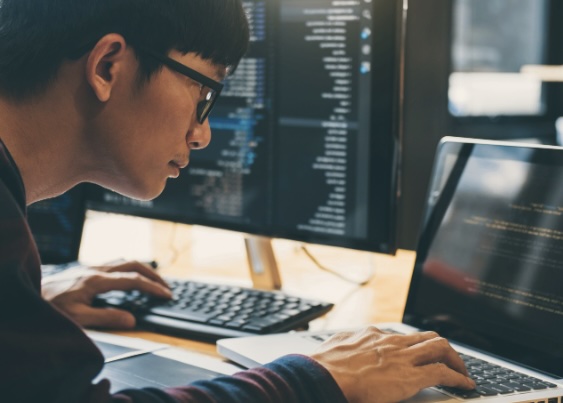
List comprehension in Python is a powerful and concise technique for creating lists. It allows you to construct lists in a more compact and elegant manner, often combining a loop and a conditional test into a single line of code. Let’s delve into how list comprehension can be used to check if something is in a list and explore its syntax and benefits.
Introduction to List Comprehension
List comprehension provides a succinct way to generate lists by applying an expression to each item in an iterable while also allowing for the inclusion of a conditional statement. This makes it particularly useful for tasks like filtering elements or performing transformations on a list.
Example of List Comprehension
Consider the following example of using list comprehension to check if something is in a list:
my_list = ['apple', 'banana', 'orange']
found_items = [item for item in my_list if item == 'banana']
In this example, the list comprehension iterates over each element (item) in my_list. For each element, it checks if the item is equal to ‘banana’ using the conditional statement if item == ‘banana’. If the condition is met, the item is included in the found_items list.
Key Features of List Comprehension
List comprehension offers several advantages and features:
- Compact Syntax: List comprehension allows you to achieve the same result with fewer lines of code compared to traditional approaches using loops;
- Readability: The syntax of list comprehension is concise and expressive, making it easier to understand and maintain code;
- Efficiency: List comprehension often results in more efficient code execution compared to equivalent loop-based approaches due to its optimized implementation in Python;
- Versatility: List comprehension can be used for a variety of tasks, including filtering, mapping, and transforming lists, providing a versatile tool for data manipulation.
Comparison with Other Techniques
While list comprehension offers many benefits, it’s important to note that it might not always be the most appropriate solution for every scenario. Here’s a comparison with other techniques:
- ‘in’ Operator: List comprehension offers a more expressive and concise syntax compared to the ‘in’ operator, especially when additional filtering or transformation is required;
- Loops: List comprehension can replace traditional loops for simple tasks, offering a more elegant and Pythonic solution.
Employing Functions and Methods
In addition to operators, loops, and list comprehensions, Python offers built-in functions and methods that can be utilized to check if something is in a list. These functions and methods provide alternative approaches to accomplish the task efficiently and effectively. Let’s explore how you can employ functions and methods, particularly the count() method, to check for the existence of an item in a list.
Introduction to Functions and Methods
Functions and methods are essential components of Python programming that allow for code organization, reuse, and abstraction. They encapsulate specific functionalities and can be invoked to perform tasks or operations.
Using the count() Method
The count() method is a built-in method in Python lists that returns the number of occurrences of a specified element in the list. It can be leveraged to check if something is in a list by examining the count of occurrences of the desired item. Here’s an example:
if my_list.count('apple') > 0:
print("Apple exists in the list!")
In this example, the count() method is called on the my_list object with the argument ‘apple’. If the count of occurrences of ‘apple’ in my_list is greater than zero, it indicates that ‘apple’ exists in the list, and the corresponding message is printed.
Key Features of the count() Method
The count() method offers several features and advantages:
- Simplicity: The count() method provides a simple and straightforward way to determine the occurrence of an item in a list;
- Directness: By directly returning the count of occurrences, the count() method eliminates the need for explicit iteration or conditional checks;
- Efficiency: The count() method is optimized for efficiency, making it suitable for large lists and frequent usage.
Comparison with Other Techniques
Let’s compare the count() method with other techniques for checking membership in a list:
- ‘in’ Operator: While the ‘in’ operator checks for membership based on existence, the count() method provides additional information about the frequency of occurrence;
- List Comprehension: List comprehension can be used to filter elements based on specific criteria, whereas the count() method focuses solely on counting occurrences.
Performance Considerations
Selecting the most efficient method for checking the existence of an item in a list is essential to ensure optimal performance. While the ‘in’ operator is often efficient for most scenarios, it’s crucial to assess performance implications based on the specific use case.
Importance of Performance
Efficient code execution is paramount, especially when dealing with large datasets or performance-sensitive applications. Slow or inefficient code can lead to increased processing time, higher resource consumption, and degraded overall system performance.
The Efficiency of the ‘in’ Operator
The ‘in’ operator is a built-in Python feature designed for fast membership testing. It leverages optimized algorithms to efficiently check for the existence of an item in a list. In many cases, the ‘in’ operator provides satisfactory performance for most use cases involving list membership checks.
Factors Affecting Performance
Several factors can influence the performance of list membership checks:
- Size of the List: The size of the list being examined directly impacts the performance of membership checks. Larger lists generally require more time for processing;
- Frequency of Checks: If membership checks are performed frequently within a loop or iterative process, the overall execution time can be significantly affected;
- Data Distribution: The distribution of data within the list, including factors such as duplicates or patterns, can affect the efficiency of membership checks.
Alternative Methods
While the ‘in’ operator is efficient for many scenarios, alternative methods such as list comprehension, the count() method, or custom functions may offer better performance under specific circumstances. It’s essential to benchmark and profile different approaches to determine the most efficient solution for your use case.
Benchmarking and Optimization
Benchmarking involves measuring the performance of different implementations to identify the most efficient approach. Techniques such as profiling can help pinpoint bottlenecks and optimize critical sections of code to improve overall performance.
Advanced Tips and Tricks
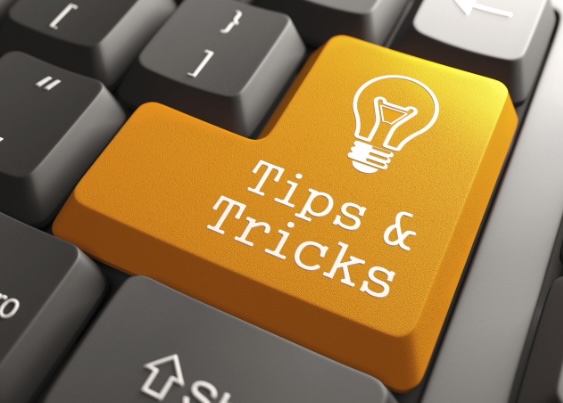
As you gain proficiency in Python programming and become more familiar with checking if something is in a list, you can explore advanced techniques to further enhance your skills and efficiency. Advanced methods such as lambda functions, filter functions, and specialized list methods like index() provide additional flexibility and power in handling list operations.
Lambda Functions
Lambda functions, also known as anonymous functions, are compact functions that can be defined inline without the need for a formal function definition. They are particularly useful when a simple function is required for a short-lived purpose.
- Syntax: Lambda functions are defined using the lambda keyword, followed by parameters and an expression. For example: lambda x: x * 2;
- Application: Lambda functions can be employed with functions like filter() and map() to perform operations on lists more succinctly.
Filter Functions
The filter() function in Python is used to filter elements from an iterable based on a specified condition. It takes a function and an iterable as arguments, returning an iterator containing the elements for which the function returns True.
- Syntax: The syntax of the filter() function is: filter(function, iterable);
- Application: By combining filter() with lambda functions or other conditional functions, you can efficiently filter elements from a list based on specific criteria.
List Methods
Python lists provide various methods that can be utilized for advanced list manipulation. One such method is index(), which returns the index of the first occurrence of a specified value in the list.
- Syntax: The syntax of the index() method is: list.index(value, start, end);
- Application: The index() method can be employed to locate the position of an item in a list, facilitating advanced list processing and manipulation.
Example Usage
my_list = [1, 2, 3, 4, 5]
# Using lambda function with filter to filter even numbers
filtered_list = list(filter(lambda x: x % 2 == 0, my_list))
# Using index() method to find the index of a specific value
index_of_3 = my_list.index(3)
In this example, a lambda function is used with the filter() function to filter even numbers from the list my_list, and the index() method is employed to find the index of the value ‘3’ in the list.
Conclusion
Knowing how to check if something is in a list in Python is a fundamental skill for any Python programmer. Whether you’re a beginner or an experienced coder, these methods and tips will enhance your coding toolkit. Always consider the context of your task to choose the most efficient and suitable method.
With these insights, you’re now equipped to efficiently determine if an item is part of your Python list.
FAQ
You can use a combination of the ‘in’ operator in a loop or list comprehension to check for multiple items.
Yes, using the index() method. For example, my_list.index(‘apple’) returns the position of ‘apple’.
The methods described work irrespective of the data types in the list.
Yes, but you might need to use nested loops or recursion for deeper levels.