Appending multiple items to a list in Python is like learning a new magic trick. It’s simple, elegant, and incredibly useful. Whether you’re a seasoned coder or a newbie, understanding how to append multiple items to a list in Python can be a game-changer in your coding journey.
Understanding Python Lists
A Python list is a fundamental data structure that serves as an ordered collection of items, each of which can be of any data type. Lists in Python are mutable, meaning they can be altered or modified after their creation. This inherent mutability makes lists highly flexible and adaptable for various programming tasks and scenarios. To better grasp the essence of Python lists, let’s explore their key characteristics:
- Ordered Collection: Lists maintain the order of elements as they are added. This implies that the position of each item within the list is preserved, allowing for sequential access and manipulation;
- Mutable Nature: One of the most significant features of Python lists is their mutability. Unlike immutable data structures such as tuples, lists can be modified after they are created. This includes adding, removing, or modifying elements within the list;
- Heterogeneous Elements: Lists in Python can accommodate elements of varying data types. This means that a single list can contain integers, floats, strings, booleans, or even other lists as its elements, providing versatility in data representation.
Creating and Manipulating Lists
In Python, lists serve as a fundamental and versatile data structure, enabling you to store and manipulate collections of items efficiently. Let’s delve deeper into the process of creating and manipulating lists in Python, exploring various operations and techniques.
Creating a List
Lists in Python are typically instantiated using square brackets []. You can initialize a list with or without elements. Here’s a basic example:
# Creating a list
my_list = [1, 2, 3]
In this example, my_list is initialized as a list containing three integer elements: 1, 2, and 3. Lists in Python can hold a variety of data types including integers, floats, strings, and even other lists.
Operations on Lists
Once a list is created, a myriad of operations can be performed on it, enhancing its flexibility and utility. Let’s explore some of the key operations:
Accessing Elements
Elements within a list can be accessed using indexing and slicing techniques. Indexing starts from 0 for the first element and negative indexing starts from -1 for the last element.
# Accessing elements
print(my_list[0]) # Output: 1
print(my_list[-1]) # Output: 3
You can also use slicing to extract a subset of elements from the list:
# Slicing
print(my_list[1:]) # Output: [2, 3]
Modifying Elements
Lists are mutable, meaning you can modify their elements after creation. This can be done by assigning new values to specific elements or utilizing built-in methods such as append(), extend(), insert(), remove(), and pop().
# Modifying elements
my_list[0] = 4 # Change the first element to 4
my_list.append(5) # Append 5 to the end of the list
Iterating Over Lists
Lists can be iterated over using loops such as for loops or comprehensions, allowing you to perform operations on each element of the list iteratively.
# Iterating over a list
for item in my_list:
print(item)
Concatenating Lists
Lists can be concatenated using the + operator or the extend() method, enabling you to combine multiple lists into a single list.
# Concatenating lists
new_list = my_list + [6, 7, 8]
The Basics of Appending Items
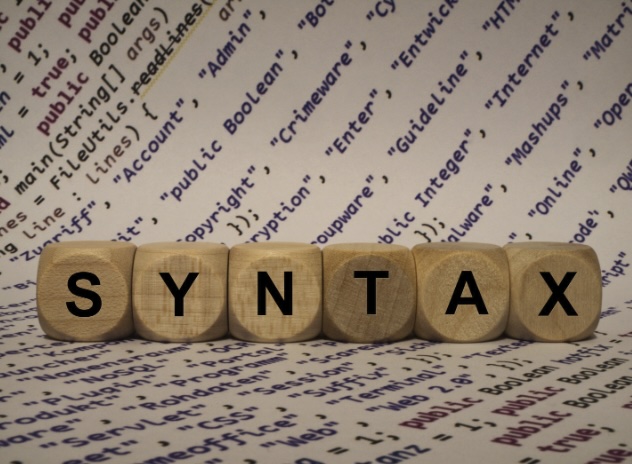
Appending an item to a list in Python is a fundamental operation that involves adding an element to the end of an existing list. This process is widely used in programming for various data manipulation tasks. Python provides a simple and efficient method called append() to accomplish this task.
Syntax
The syntax for the append() method is straightforward:
list_name.append(item)
Here, list_name refers to the name of the list to which the item will be appended, and item represents the element that you want to add to the list.
Example
Let’s consider an example to demonstrate the usage of the append() method:
my_list = [1, 2, 3]
my_list.append(4)
After executing the append() operation, the value of my_list becomes [1, 2, 3, 4]. This example illustrates how the append() method adds the item 4 to the end of the list my_list.
Advantages of Append Method
The append() method offers several advantages:
- Simplicity: The syntax is straightforward, making it easy to understand and use;
- Efficiency: Appending an item to the end of a list using append() is an efficient operation, particularly for large lists;
- In-place Modification: The append() method modifies the original list in-place, avoiding the need for creating a new list or copying elements.
Use Cases
The append() method is commonly used in scenarios where new elements need to be added to an existing list. Some typical use cases include:
- Building Lists Dynamically: When you’re constructing a list dynamically and need to add elements as you go;
- Processing Data Streams: In scenarios where data is arriving incrementally, appending allows for processing data as it becomes available;
- Stack Implementation: Appending items to a list is often used in implementing a stack data structure.
Comparison with Other Methods
While the append() method is convenient for adding elements to the end of a list, Python offers other methods for list manipulation, each with its own use cases:
- extend(): This method is used to append elements from an iterable to the end of the list, effectively extending the list;
- List Concatenation: Using the + operator to concatenate lists can also achieve similar results, but it creates a new list rather than modifying the original one;
- List Insertion: The insert() method allows inserting an element at a specific position in the list, providing more flexibility than append().
How to Append Multiple Items to a List in Python
Appending multiple items to a list in Python can be achieved through various methods, each offering its own advantages. Below, we explore three commonly used techniques:
Using extend() Method
The extend() method in Python is specifically designed to add elements from an iterable (such as a list, set, or tuple) to the end of an existing list.
Syntax:
list_name.extend(iterable)
Example:
my_list = [1, 2, 3]
my_list.extend([4, 5])
# Now, my_list is [1, 2, 3, 4, 5]
This method offers a straightforward way to add multiple items to a list without the need for additional concatenation or list manipulation.
Using + Operator
The + operator in Python can be used to concatenate two lists, effectively appending one list to another.
my_list = [1, 2, 3]
my_list = my_list + [4, 5]
# Now, my_list is [1, 2, 3, 4, 5]
While this method achieves the desired result, it involves creating a new list by combining the original list with the additional elements, which may not be as efficient as the extend() method for large lists.
Using List Comprehension
List comprehension provides a more flexible approach for appending multiple items to a list, particularly in scenarios where additional processing or filtering is required.
my_list = [1, 2, 3]
my_list = [x for x in my_list] + [4, 5]
# Now, my_list is [1, 2, 3, 4, 5]
By using list comprehension, you can apply transformations or conditions to the elements of the original list before appending the additional items. However, this method may be less concise and less efficient compared to the extend() method, especially for simple appending operations.
When to Use Each Method
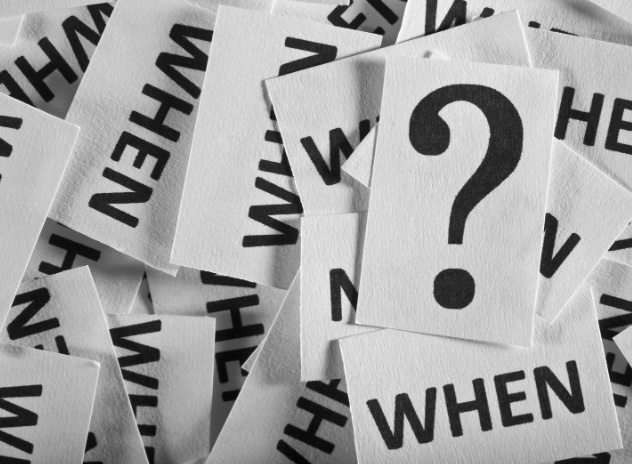
Depending on the specific requirements and context of your code, you may choose different methods for achieving this task efficiently. In Python, some commonly used methods for appending multiple items to a list include extend(), the + operator, and list comprehension. Each of these methods has its own specific use case, and understanding when to use each one is essential for writing clean, efficient, and readable code.
Use extend() Method
The extend() method is used when you have an iterable and want to add its elements to an existing list. This method is particularly useful when you have another list or any iterable object from which you want to append elements to your list. Here’s how you can use the extend() method:
# Example of using extend() method
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
Use + Operator
The + operator in Python is not only used for arithmetic addition but also for concatenating sequences like lists. When you want to join two lists and assign the result to a new list, the + operator can be a convenient choice. However, it’s important to note that using the + operator creates a new list rather than modifying any of the original lists. Here’s an example:
# Example of using + operator
list1 = [1, 2, 3]
list2 = [4, 5, 6]
new_list = list1 + list2
print(new_list) # Output: [1, 2, 3, 4, 5, 6]
Use List Comprehension
List comprehension is a concise and elegant way to create lists in Python. It can also be used for appending elements conditionally or through a transformation. When you need to add elements to a list based on certain conditions or after applying some transformation to the elements, list comprehension provides a readable and efficient solution. Here’s how you can use list comprehension for appending elements conditionally:
# Example of using list comprehension
numbers = [1, 2, 3, 4, 5]
# Append only even numbers to a new list
even_numbers = [x for x in numbers if x % 2 == 0]
print(even_numbers) # Output: [2, 4]
In this example, only the even numbers from the original list are appended to the new list using list comprehension.
Append Multiple Items in a Loop
Appending items to a list within a loop is a common requirement in Python programming, especially when you need to dynamically generate or collect data. This process involves iterating through a sequence or performing some calculations and then adding the resulting elements to a list. Python provides several approaches to accomplish this task efficiently.
Using a Loop with append() Method
One straightforward method to append items to a list in a loop is by iterating through the loop and using the append() method to add each item to the list. Here’s an example demonstrating this approach:
# Example of appending items to a list in a loop
my_list = []
for i in range(3):
my_list.append(i)
print(my_list) # Output: [0, 1, 2]
In this example, a list my_list is initialized as an empty list. Then, a for loop iterates over the range of numbers from 0 to 2 (exclusive). Within each iteration, the value of i is appended to the my_list using the append() method. Finally, the resulting list is printed, showing [0, 1, 2].
Efficiency Considerations
When appending items to a list within a loop, it’s essential to consider the efficiency of your code, especially for large datasets or performance-critical applications. Here are some considerations:
- Preallocating Memory: If you know the approximate size of the final list beforehand, preallocating memory can improve efficiency by reducing the number of memory allocations. You can achieve this by initializing the list with a predefined size, although this might not always be practical;
- List Comprehension: In some cases, using list comprehension can offer a more concise and efficient alternative to appending items in a loop, particularly when applying transformations or filtering elements;
- Time Complexity: The time complexity of appending items to a list within a loop is O(1) for each append operation. However, if the size of the list grows significantly, the overall time complexity can approach O(n), where n is the number of elements in the list.
Best Practices and Performance
When appending multiple items to a list in Python, it’s essential to consider various factors to ensure optimal performance and resource usage. Here are some best practices to follow along with performance considerations:
Use extend() for Better Performance
The extend() method is generally preferred over the + operator when appending multiple items to a list, especially when dealing with large datasets. This is because extend() directly modifies the original list in place, while the + operator creates a new list by concatenating the existing lists. The extend() method is optimized for adding elements from an iterable, resulting in better performance for appending multiple items. Here’s a comparison:
# Using extend() method
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
# Using + operator
list3 = [1, 2, 3]
list4 = [4, 5, 6]
new_list = list3 + list4
In this example, list1 is modified in place using extend(), while new_list is created as a new list using the + operator.
Be Mindful of Memory Usage
Appending multiple items to a list, especially in a loop or when dealing with large datasets, can lead to significant memory consumption. It’s essential to be mindful of memory usage to avoid running into memory-related issues, such as out-of-memory errors or excessive memory allocation. Consider the following:
- Preallocating Memory: If you know the approximate size of the final list beforehand, preallocating memory can help optimize memory usage by reducing the number of memory allocations. However, this approach might not always be practical or feasible;
- Memory Profiling: Use memory profiling tools to analyze memory usage patterns and identify potential areas for optimization. This can help you pinpoint memory-intensive operations and optimize your code accordingly.
Immutable vs. Mutable
It’s crucial to understand the distinction between immutable and mutable objects when appending items to a list in Python. Both extend() and append() methods modify the list in place, while the + operator creates a new list. Consider the following:
- Mutable Operations: extend() and append() are mutable operations, meaning they directly modify the original list without creating a new list;
- Immutable Operation: The + operator creates a new list by concatenating the existing lists, resulting in an immutable operation.
Conclusion
Understanding how to append multiple items to a list in Python is a fundamental skill that can enhance your coding efficiency. Whether you’re manipulating data, building complex structures, or just organizing your ideas, these techniques provide the flexibility and power needed to work with lists effectively. Remember, practice is key, so try out these methods and see how they can fit into your next Python project!
FAQ
Yes, Python lists are heterogeneous, meaning you can mix data types in a list.
Using append() will add the entire iterable as a single element in the list.
Yes, use slicing and concatenation. For example, my_list[1:1] = [4, 5] inserts at index 1.
No, extend() only works with iterables. For single items, use append().
You can chain extend() calls or concatenate lists using +.