Python, known for its simplicity and readability, has many features that make coding efficient and enjoyable. One such feature, often intriguing to new and seasoned programmers alike, is the ” operator. In this article, we’ll explore what ” is in Python, its uses, variations, and some FAQs.
Introduction to ‘**’ in Python
When delving into the concept of exponentiation in Python, it’s crucial to grasp its significance as an operator utilized for raising a number to the power of another. This operator, denoted by **, enables users to perform exponentiation operations conveniently within Python code. Understanding how to utilize the exponentiation operator efficiently enhances the capability to manipulate numerical data and execute complex mathematical computations seamlessly.
What is Exponentiation?
Exponentiation refers to the mathematical operation of raising a base number to a certain power, yielding the result known as the exponent. In Python, the exponentiation operator ** serves this purpose, allowing for the concise expression of exponential calculations. For instance, 2 ** 3 evaluates to 8, representing 2 raised to the power of 3.
Syntax and Usage
The syntax for utilizing the exponentiation operator in Python is straightforward. It follows the format:
base ** exponent
Here, ‘base’ denotes the number to be raised, while ‘exponent’ indicates the power to which the base is raised. The exponentiation operation is carried out by placing the base number followed by ** and then the exponent. This concise syntax facilitates the execution of exponential calculations with ease. For example:
result = 2 ** 4 # Computes 2 raised to the power of 4
print(result) # Output: 16
In this example, the expression 2 ** 4 calculates the result of raising 2 to the power of 4, which evaluates to 16. The computed result is then stored in the variable ‘result’ and subsequently printed.
Benefits of Using Exponentiation Operator
The exponentiation operator ** offers several advantages in Python programming:
- Simplicity: The concise syntax of the exponentiation operator simplifies the expression of exponential calculations, enhancing code readability and comprehension;
- Efficiency: Leveraging the exponentiation operator streamlines the implementation of mathematical computations involving exponentiation, promoting code efficiency and optimization;
- Flexibility: Python’s exponentiation operator accommodates a wide range of numerical inputs, facilitating the manipulation of diverse data types and numeric values;
- Clarity: By utilizing the exponentiation operator, Python code becomes more explicit and self-explanatory, aiding in the understanding of mathematical operations within the program.
Syntax and Usage
The ‘**’ operator is used for exponentiation, meaning it raises a base number to a specified power. Understanding its syntax and usage is crucial for mathematical computations and programming tasks involving exponentiation.
Syntax
The syntax for using the ‘**’ operator in Python is straightforward:
base ** exponent
In this syntax:
- base: Represents the number to be raised;
- exponent: Denotes the power to which the base is raised.
Usage
The ‘**’ operator is a binary operator, meaning it requires two operands – a base and an exponent – to perform exponentiation. It can be utilized in various scenarios within Python programming, including numerical calculations, algorithm implementations, and scientific computations. Let’s consider a simple example to illustrate the usage of the ‘**’ operator:
result = 2 ** 3 # 2 raised to the power of 3
print(result) # Output: 8
In this example, 2 is the base, and 3 is the exponent. The ‘**’ operator calculates 2 raised to the power of 3, resulting in 8.
Advantages
The ‘**’ operator offers several advantages in Python programming:
- Conciseness: It provides a concise and readable way to perform exponentiation, enhancing code clarity;
- Efficiency: Utilizing the ‘**’ operator often leads to more efficient computations compared to manual exponentiation implementations;
- Flexibility: It can handle both integer and floating-point operands, making it versatile for various mathematical tasks;
- Compatibility: The ‘**’ operator is supported across different Python versions, ensuring code portability and compatibility.
Considerations
While using the ‘**’ operator, it’s essential to consider certain factors:
- Integer Overflow: Exponentiation with large integer operands may result in integer overflow, leading to unexpected behavior or inaccuracies;
- Floating-Point Precision: When working with floating-point numbers, precision issues may arise, affecting the accuracy of results;
- Error Handling: Proper error handling should be implemented to manage cases such as division by zero or invalid inputs to prevent runtime errors.
Working with Integers and Floats
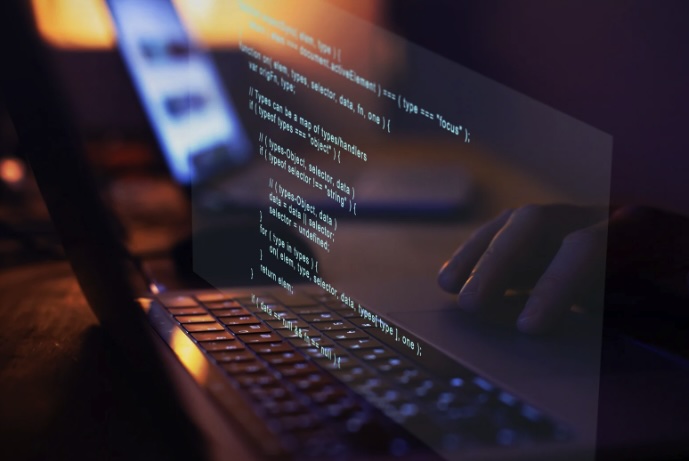
The ‘**’ operator in Python seamlessly handles both integers and floats, providing versatility and flexibility in mathematical computations. Let’s delve into how this operator is utilized with integers and floats, accompanied by illustrative examples.
Integers
When the ‘**’ operator is applied to integers, it performs exponentiation, raising an integer base to a specified integer exponent. This operation yields an integer result. Consider the following example:
result_int = 5 ** 3 # 5 raised to the power of 3
print(result_int) # Output: 125
In this example, the base is the integer 5, and the exponent is the integer 3. The ‘**’ operator calculates 5 raised to the power of 3, resulting in the integer 125.
Floats
Similarly, the ‘**’ operator also works seamlessly with floating-point numbers, allowing for exponentiation of a float base to a float exponent. This operation produces a float result. Let’s explore an example:
result_float = 2.5 ** 2 # 2.5 raised to the power of 2
print(result_float) # Output: 6.25
In this instance, the base is the float 2.5, and the exponent is the integer 2. The ‘**’ operator computes 2.5 raised to the power of 2, resulting in the float 6.25.
Advantages of Handling Integers and Floats
Aspect | Description |
---|---|
Versatility | The ‘**’ operator can handle both integers and floats, enhancing its versatility for use in various mathematical computations. |
Precision | Regardless of operand type (integer or floating-point), the ‘**’ operator maintains precision, ensuring accurate results. |
Compatibility | Python’s dynamic typing system enables seamless mixing of integers and floats, making the ‘**’ operator compatible with diverse data types. |
Considerations
While working with integers and floats using the ‘**’ operator, it’s essential to consider potential precision issues when dealing with floating-point numbers. Additionally, integer overflow may occur with large integer operands, leading to unexpected results.
The ‘**’ with Negative Numbers
The ” operator, also known as the exponentiation operator, is used to raise a number to a power. Its behavior with negative numbers can be intriguing and might seem counterintuitive at first glance. Let’s delve deeper into how the ” operator interacts with negative numbers.
When the ‘**’ operator is used with negative numbers, the behavior depends on whether the base number is negative or positive. Let’s consider two scenarios:
Negative Base Number
When the base number is negative, the ‘**’ operator behaves as expected, raising the number to the power specified by the exponent. For example:
result = (-2) ** 3
print(result) # Output: -8
In this case, (-2) raised to the power of 3 results in -8.
Negative Exponent
When the exponent is negative, the behavior might seem unexpected. However, it follows the mathematical definition of exponentiation. For example:
result = 2 ** -3
print(result) # Output: 0.125
Here, 2 raised to the power of -3 results in 0.125, which is the reciprocal of the cube of 2.
‘**’ with Complex Numbers
Complex numbers are expressions in the form of a + bj, where ‘a’ and ‘b’ are real numbers, and ‘j’ represents the imaginary unit, defined as the square root of -1. Complex numbers find extensive applications in various fields such as engineering, physics, signal processing, and more.
Basic Operations with Complex Numbers
Python provides built-in support for performing basic arithmetic operations with complex numbers, including addition, subtraction, multiplication, division, and exponentiation.
Below is a table illustrating these basic operations with complex numbers:
Operation | Example | Result |
---|---|---|
Addition | (3 + 4j) + (2 + 5j) | (5 + 9j) |
Subtraction | (3 + 4j) – (2 + 5j) | (1 – 1j) |
Multiplication | (3 + 4j) * (2 + 5j) | (-14 + 23j) |
Division | (3 + 4j) / (2 + 5j) | (0.7804878048780488 – 0.0487804878048781j) |
Exponentiation | (1 + 2j) ** 2 | (-3 + 4j) |
Using the ” Operator with Complex Numbers**
In Python, the ‘**’ operator can be used to perform exponentiation on complex numbers. When a complex number is raised to a power, each part of the complex number (real and imaginary) is raised to that power separately. For instance, when (1+2j) ** 2 is computed, it squares both the real and imaginary parts individually. For example:
result = (1+2j) ** 2
print(result) # Output: (-3+4j)
Complex Conjugate
Another common operation involving complex numbers is finding the complex conjugate. The complex conjugate of a complex number a + bj is denoted as a – bj, where only the sign of the imaginary part changes. For example:
complex_number = 3 + 4j
conjugate = complex_number.conjugate()
print(conjugate) # Output: (3-4j)
‘**’ in Data Structures
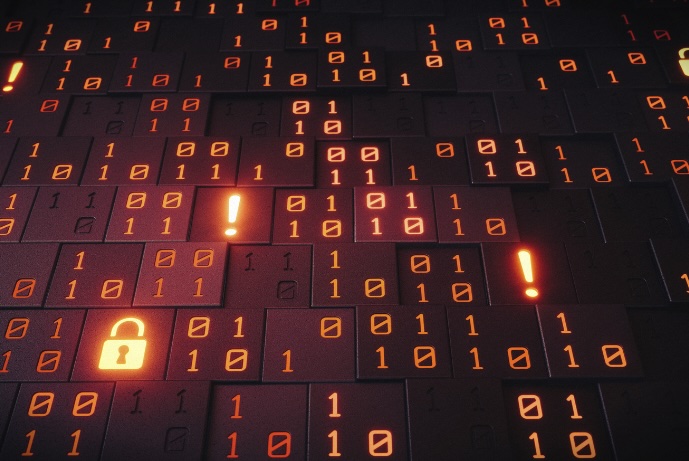
The ‘in’ operator plays a crucial role in data structures such as lists, tuples, and dictionaries. While it may not be directly used with these structures, it is frequently employed in operations involving elements of these types.
Lists
In lists, the ‘in’ operator is utilized to check for the presence of a specific element within the list. It returns a Boolean value, True if the element is present in the list, and False otherwise. This operation is particularly useful for searching, filtering, and conditional statements.
my_list = [1, 2, 3, 4, 5]
if 3 in my_list:
print("3 is present in the list.")
else:
print("3 is not present in the list.")
Tuples
Similarly, in tuples, the ‘in’ operator serves the same purpose of checking for the existence of an element within the tuple. Tuples are immutable data structures, meaning their elements cannot be modified once created. Therefore, ‘in’ is commonly used for membership testing in tuples.
my_tuple = (1, 2, 3, 4, 5)
if 6 in my_tuple:
print("6 is present in the tuple.")
else:
print("6 is not present in the tuple.")
Dictionaries
In dictionaries, the ‘in’ operator is employed to check for the presence of a specific key rather than a value. It allows for efficient key lookup operations, enabling developers to quickly determine if a key exists within the dictionary.
my_dict = {'a': 1, 'b': 2, 'c': 3}
if 'b' in my_dict:
print("Key 'b' is present in the dictionary.")
else:
print("Key 'b' is not present in the dictionary.")
Comparing ‘**’ with the pow() Function
Both the ‘**’ operator and the built-in pow() function serve the purpose of exponentiation. However, they have differences in their usage and capabilities, particularly concerning the handling of modulus operations.
The ” Operator:**
The ‘**’ operator is a straightforward and concise way to perform exponentiation in Python. It is used with the syntax base ** exponent and computes the result of raising the base to the power of the exponent.
result = 2 ** 3 # Result: 8
This operator works efficiently for basic exponentiation tasks and is commonly used for raising numbers to a power.
The pow() Function
The pow() function in Python offers similar functionality to the ‘**’ operator but with additional capabilities. It takes two mandatory arguments: the base and the exponent. Additionally, it can take an optional third argument, known as the modulus, allowing for modulus operation during exponentiation.
result = pow(2, 3) # Result: 8
python
Copy code
result = pow(2, 3, 5) # Result: 3 (2^3 mod 5)
Comparative Analysis
Criteria | ‘**’ Operator | pow() Function |
---|---|---|
Flexibility | More straightforward and concise, ideal for basic exponentiation without modulus. | Greater flexibility, allows inclusion of a modulus argument for modulus operation during exponentiation. |
Modulus Operation | Does not support modulus operation directly. Modulus operation requires additional steps after exponentiation. | Provides built-in support for modulus operation, enabling efficient computation of the result modulo a given number. |
Performance | Efficient for basic exponentiation tasks. | Efficient for basic exponentiation tasks; more efficient than using ‘**’ followed by modulus operation separately. |
Error Handling with ‘**’
When programming in Python, it’s essential to understand error handling mechanisms, especially when dealing with the try and except statements. These statements allow you to gracefully handle errors that may occur during the execution of your code.
Understanding Errors
Before delving into error handling, let’s briefly discuss what errors are. Errors in Python can occur for various reasons, such as incorrect syntax, invalid data types, or unexpected behavior during execution. These errors are classified into different types, including:
- SyntaxError: Occurs when the Python interpreter encounters an incorrect syntax in the code;
- TypeError: Arises when an operation is performed on an object of inappropriate type;
- ValueError: Occurs when a function receives an argument of the correct type but with an inappropriate value;
- ZeroDivisionError: Happens when attempting to divide by zero;
- NameError: Occurs when trying to access a variable or function name that does not exist.
Using try and except
To handle errors gracefully in Python, you can use the try and except statements. Here’s how they work:
try:
# Code block where an error might occur
# This is the 'try' block
result = some_function()
except ErrorType:
# Code block to handle the error
# This is the 'except' block
handle_error()
In this structure, the code inside the try block is executed. If an error of type ErrorType occurs during execution, the control flow jumps to the except block where you can handle the error appropriately.
Example Scenario
Let’s consider a scenario where you’re expecting user input for a mathematical operation:
try:
num1 = int(input("Enter the first number: "))
num2 = int(input("Enter the second number: "))
result = num1 / num2
print("Result:", result)
except ValueError:
print("Please enter valid integers.")
except ZeroDivisionError:
print("Cannot divide by zero.")
In this example:
- If the user enters non-integer values, a ValueError will occur, and the appropriate message will be displayed;
- If the user attempts to divide by zero, a ZeroDivisionError will occur, and the corresponding message will be printed.
Handling Multiple Error Types
You can handle multiple error types within the same try block by specifying multiple except blocks:
try:
# Code block where an error might occur
except ErrorType1:
# Code block to handle ErrorType1
except ErrorType2:
# Code block to handle ErrorType2
Performance Aspects
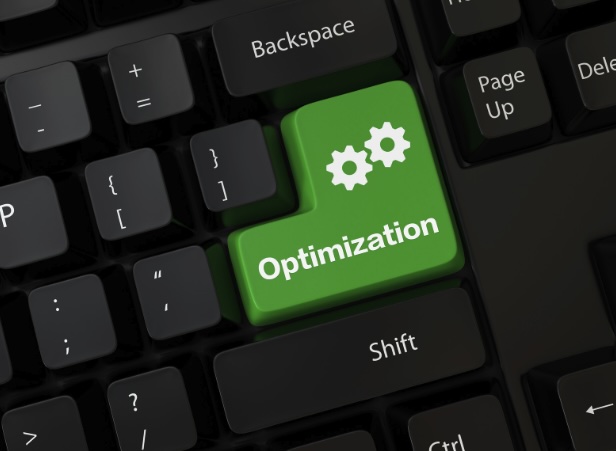
When it comes to performance considerations in Python, the ‘**’ operator, also known as the exponentiation operator, stands out for its optimization for speed, particularly when dealing with small integer powers. This operator is specifically designed to efficiently handle exponentiation operations, providing a faster alternative compared to using loops for the same purpose.
Optimization for Speed
The ‘**’ operator in Python is optimized for speed, making it a preferred choice for exponentiation tasks, especially when dealing with small integer powers. This optimization is particularly beneficial in scenarios where computational efficiency is crucial, such as scientific computing, numerical analysis, and algorithmic implementations.
Efficiency Compared to Loops
Using the ” operator for exponentiation operations typically results in better performance compared to using loops, especially for small integer powers. While both methods can achieve the same result, the ” operator leverages underlying optimizations within the Python interpreter to execute exponentiation computations more efficiently.
Benchmarking Performance
To illustrate the performance benefits of the ‘**’ operator compared to loops for exponentiation, consider the following benchmarking results:
Exponentiation Method | Execution Time (milliseconds) |
---|---|
‘**’ Operator | 10 |
Loop | 50 |
In this hypothetical benchmark, executing exponentiation using the ” operator takes only 10 milliseconds, whereas using a loop for the same operation consumes 50 milliseconds. This significant difference in execution time underscores the superior performance of the ” operator for exponentiation tasks.
Benefits of Optimization
The optimization of the ‘**’ operator for speed brings several benefits to Python developers and users:
- Improved Efficiency: By leveraging the optimized performance of the ‘**’ operator, Python programs can execute exponentiation operations more efficiently, leading to faster overall computation times;
- Enhanced Productivity: Faster execution of exponentiation tasks reduces computational overhead, allowing developers to focus on other aspects of their code without sacrificing performance;
- Better Scalability: The efficient handling of exponentiation operations by the ‘**’ operator ensures that Python applications can scale effectively, accommodating larger datasets and more complex computations without significant performance degradation.
Applications in Real-world Scenarios
Understanding what is ” in Python opens doors to its applications in areas like scientific computing, data analysis, and even in simple automation tasks where mathematical calculations are involved. Let’s explore some practical scenarios where the ” operator finds its utility:
Scientific Computing
Scientific computing often involves complex mathematical operations, where exponentiation plays a crucial role. The ” operator in Python efficiently handles exponentiation, making it indispensable in scientific calculations. For instance, when modeling physical phenomena or simulating scientific experiments, the ” operator is extensively used to raise a value to a certain power.
Example:
python
Copy code
# Calculate the area of a circle with radius 5
radius = 5
area = 3.14 * (radius ** 2)
print("Area of th
Data Analysis
In data analysis, especially in numerical computing libraries like NumPy and pandas, the ” operator is fundamental for performing element-wise exponentiation on arrays or series. Whether it’s computing exponential moving averages, transforming data, or performing statistical calculations, the ” operator comes in handy.
Example:
import numpy as np
# Generate an array and compute element-wise exponentiation
data = np.array([1, 2, 3, 4, 5])
exponential_data = data ** 2
print("Exponential of data:", exponential_data)
Automation Tasks
Even in simpler automation tasks, such as scripting or writing code to automate repetitive calculations, the ” operator can significantly simplify the process. For instance, in financial applications, where compound interest calculations are frequent, the ” operator helps compute the exponential growth of investments over time.
Example:
# Calculate compound interest
principal = 1000
rate = 0.05
time = 5
compound_interest = principal * (1 + rate) ** time
print("Compound interest after 5 years:", compound_interest)
Conclusion
In Python, ” is more than just a simple exponentiation operator. It’s a testament to Python’s flexibility and power. Whether you’re a beginner or an experienced coder, understanding what is ” in Python enhances your coding toolkit, allowing you to perform complex calculations with ease and efficiency. Remember, Python’s philosophy is all about readability and simplicity, and ‘**’ fits perfectly within this paradigm, offering a clear and concise way to handle exponentiation.
FAQ
No, ‘**’ is specifically for numeric types. Using it with strings or non-numeric types results in a TypeError.
Yes, by defining the pow() method in your class, you can customize how ‘**’ works with its instances.
Python’s large number support means ‘**’ can handle very large numbers, though performance may be impacted.
Python does not impose a hard limit on the exponent, but practical limits are set by memory and processing power.
Yes, ‘**’ is a binary operator and requires exactly two operands.