Dive into the world of Python and Linux with this essential guide! Discover the ins and outs of executing Python scripts in the Linux environment, tailored for both beginners and seasoned developers. This article provides a comprehensive journey from basic setup to advanced techniques, ensuring you’re well-equipped to harness the power of Python in Linux. Let’s get started and master the art of Python scripting in Linux!
Getting Started with Python on Linux
Getting started with Python on Linux involves a few essential steps to ensure your system is set up correctly. This guide will walk you through checking whether Python is already installed on your Linux system and, if not, how to install it. Python and Linux are highly compatible, making it a seamless process to get started with Python programming on this operating system.
Checking Python Installation
The first step is to determine whether Python is already installed on your Linux system. To do this, follow these steps:
- Open a Terminal: Launch a terminal window on your Linux system. This can usually be done by searching for “Terminal” in your system’s applications menu;
- Check Python Version: In the terminal, type either python –version or python3 –version and press Enter. This command will display the version of Python installed on your system.
Scenario | Outcome |
---|---|
If Python is installed | The terminal will output the version number, indicating that Python is already set up on your system. |
If Python is not installed | You’ll receive an error message indicating that the command is not recognized, implying that Python is not installed on your system. |
Installing Python
If Python is not installed on your Linux system, you can easily install it using your system’s package manager. Most Linux distributions come with Python pre-installed, but if it’s missing, follow these general steps:
- Open Terminal: Launch a terminal window on your Linux system;
- Use Package Manager: Depending on your Linux distribution, you’ll use a different package manager to install Python. Here are examples for a few common distributions:
Linux Distribution | Installation Command |
---|---|
Ubuntu/Debian | sudo apt-get install python3 |
Fedora | sudo dnf install python3 |
CentOS/RHEL | sudo yum install python3 |
- Replace python3 with python if you prefer Python 2, although it’s recommended to use Python 3 for new projects as Python 2 has reached its end of life.
Running Your First Python Script
Now that you’ve successfully installed Python, it’s time to dive into writing and executing your very first Python script. In this tutorial, we’ll guide you through the process of creating a simple “Hello, World!” program and running it on a Linux system.
Create the Script
To begin, let’s open a text editor. You can use any text editor of your choice, such as Vim, Nano, or even a graphical text editor like Gedit or Sublime Text. Once the text editor is open, follow these steps:
- Open Text Editor: Launch your preferred text editor from the terminal or application menu;
- Write the Script: In the text editor, type the following Python code: print(“Hello, World!”);
- Save the Script: Save the file with a meaningful name and the .py extension. For example, you can save it as hello.py;
- Verify: Ensure that the file is saved in a directory where you can easily locate it later.
Your script should now be ready, containing a single line of code that prints “Hello, World!” to the console.
Run the Script
Now that the script is saved, let’s move on to running it in a Linux environment. Follow these steps:
- Open Terminal: Launch a terminal window. You can usually find the terminal application in your system’s application menu or by searching for “Terminal”;
- Navigate to the Directory: Use the cd command to navigate to the directory where you saved your hello.py script. For example: cd /path/to/directory;
- Execute the Script: Once you’re in the correct directory, type the following command and press Enter: python3 hello.py;
- View Output: After executing the command, you should see the output “Hello, World!” printed to the terminal.
Exploring Different Ways to Execute a Python Script in Linux
In the Linux environment, there exists a multitude of methods for executing Python scripts, each catering to various needs and preferences. Below, we delve into three prominent ways:
Direct Execution
Direct execution involves making your Python script directly executable from the terminal. Here’s a step-by-step breakdown:
- Shebang Line: Begin your Python script with a shebang line, such as #!/usr/bin/env python3. This line informs the system that the script should be executed using the Python 3 interpreter;
- Permissions: Grant execute permission to your script using the chmod command. For instance, chmod +x hello.py assigns execute permissions to the hello.py script;
- Execution: Execute the script directly from the terminal by typing ./hello.py. The preceding ./ signifies that the script resides in the current directory.
Direct execution offers simplicity and convenience, allowing you to run Python scripts with minimal effort.
Using an IDE
Integrated Development Environments (IDEs) provide powerful platforms for writing, debugging, and executing Python scripts. Popular choices include PyCharm and Visual Studio Code (VSCode). Here’s how you can execute Python scripts using an IDE:
- Installation: Install your preferred IDE from the official website or via package managers like apt or snap;
- Open Script: Launch the IDE and open the Python script you wish to execute;
- Execution: Utilize the IDE’s built-in execution capabilities. Typically, you can execute scripts by clicking a “Run” button or using keyboard shortcuts.
IDEs offer a plethora of features beyond simple script execution, including syntax highlighting, code completion, and integrated debugging, enhancing the development experience.
Scheduling with Cron
Linux’s cron scheduler enables automated execution of tasks at specified intervals. This method is ideal for running Python scripts on a recurring basis. Here’s how you can schedule Python script execution with cron:
- Access Cron: Open the cron table using the crontab -e command. This command allows you to edit the cron jobs associated with your user account;
- Define Schedule: Add an entry specifying the schedule at which you want your Python script to run. For example: 0 0 * * * /usr/bin/python3 /path/to/script.py;
- This entry schedules the script to run daily at midnight;
- Save and Exit: Save the changes to the cron table and exit the editor. Cron will automatically pick up the new schedule.
Cron provides a robust solution for automating Python script execution, enabling hands-free operation of tasks according to predefined schedules.
Python Script Arguments and Linux
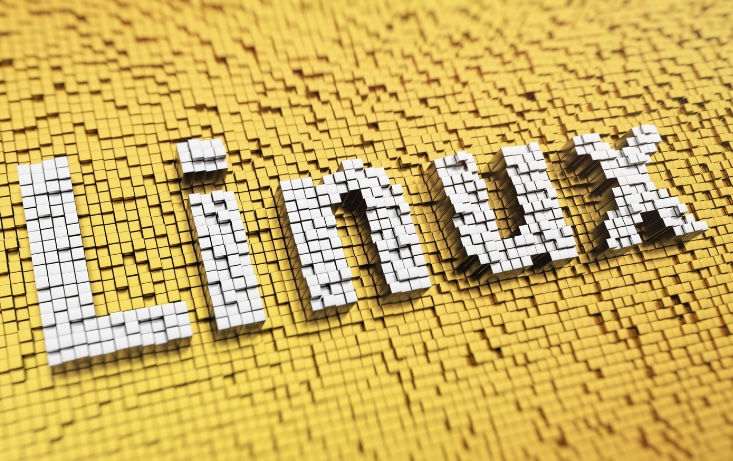
Passing arguments to a Python script can significantly enhance its functionality, enabling dynamic behavior tailored to specific user inputs. In Linux, leveraging command-line arguments provides a convenient way to interact with Python scripts. Let’s delve into how this process works:
Understanding sys.argv
In Python, the sys.argv array is a mechanism for accessing command-line arguments passed to a script. This array contains the script’s filename as the first element (sys.argv[0]), followed by any additional arguments provided by the user. Here’s a breakdown of how it works:
- Usage: After invoking the Python interpreter and specifying the script filename, additional arguments can be appended, separated by spaces. For example: python3 script.py arg1 arg2;
- Accessing Arguments: Within the Python script, you can access the command-line arguments using the sys.argv array. For instance:
import sys
# Accessing arguments
arg1 = sys.argv[1]
arg2 = sys.argv[2]
Utilizing sys.argv, Python scripts gain the flexibility to accept inputs from the command line, enabling customization and adaptability.
Passing Arguments to Python Scripts
Let’s explore a practical example of passing arguments to a Python script in Linux:
- Script Definition: Suppose we have a Python script named script.py that performs a specific task based on user-provided arguments;
- Execution: To execute the script with arguments, navigate to the directory containing the script in the terminal and use the following command format: python3 script.py arg1 arg2;
- Replace arg1 and arg2 with the desired arguments;
- Script Implementation: Within the script.py file, access the passed arguments using sys.argv and incorporate them into the script’s logic as needed.
By passing arguments through the command line, users can customize script behavior dynamically, enhancing its utility and versatility.
Enhancing Script Functionality
Command-line arguments empower Python scripts to adapt to various scenarios and user requirements. Consider the following strategies for maximizing script functionality:
- Error Handling: Implement robust error handling to gracefully manage unexpected inputs or missing arguments;
- Argument Validation: Validate and sanitize user inputs to ensure they adhere to expected formats or constraints;
- Usage Instructions: Provide clear usage instructions and help messages to guide users on how to interact with the script effectively.
By incorporating these practices, Python scripts become more user-friendly and reliable, fostering a positive user experience.
Environment Variables and Python in Linux
Environment variables serve as dynamic storage containers for various types of information, including file paths, configuration settings, and user-defined values. Let’s explore how environment variables interact with Python in the Linux environment:
Introduction to Environment Variables
Environment variables are dynamic values that are part of the operating system’s environment. They can be accessed by all processes running on the system and play a crucial role in determining the behavior and configuration of programs and scripts. Common use cases for environment variables include specifying paths to executables, defining system-wide configuration settings, and storing sensitive information like API keys or passwords.
Setting Environment Variables in Linux
In Linux, environment variables can be set using the export command followed by the variable name and its value. Here’s a breakdown of the process:
- Syntax: Use the export command followed by the variable name, an equal sign, and the desired value. For example: export MY_VAR=value;
- Persistence: Environment variables set using the export command are typically valid for the duration of the current terminal session. To make them persistent across sessions, you can add them to configuration files like .bashrc or .profile.
Accessing Environment Variables in Python
Python provides the os.environ dictionary, which allows you to access environment variables within your Python scripts. Here’s how you can utilize it:
- Import os Module: Begin by importing the os module in your Python script: import os;
- Accessing Variables: You can access environment variables using the os.environ dictionary. For example, to access the value of MY_VAR set earlier: my_var_value = os.environ.get(‘MY_VAR’);
- Error Handling: It’s important to handle cases where the environment variable may not be set. You can use the .get() method with a default value or check for existence using the in operator.
Practical Applications
Environment variables in Python scripts offer versatility and flexibility in managing configurations and settings. Here are some practical applications:
- Configuration Management: Use environment variables to store sensitive information like database credentials or API keys without hardcoding them into your scripts;
- Path Resolution: Set environment variables for commonly used file paths to enhance script portability and maintainability;
- Dynamic Behavior: Adjust script behavior based on environment variables, allowing for customization without modifying the script code.
Debugging Python Scripts in Linux
Linux provides a range of tools specifically designed to aid in the debugging process for Python scripts. One such tool is the built-in debugger called pdb (Python Debugger). Let’s explore how you can utilize pdb and other debugging techniques in the Linux environment:
Introduction to Debugging in Linux
Debugging involves the process of identifying and correcting errors, exceptions, or unexpected behavior in your Python scripts. In Linux, developers have access to a variety of tools and techniques to facilitate this process, ensuring efficient troubleshooting and problem resolution.
Using pdb (Python Debugger)
Python includes a powerful built-in debugger called pdb, which allows developers to interactively debug their scripts. Here’s how you can leverage pdb in your Python scripts:
- Integration: To start debugging with pdb, insert the following line of code at the location where you want to initiate debugging: import pdb; pdb.set_trace();
- Execution: When the interpreter encounters this line during script execution, it halts execution and enters the pdb debugger prompt, enabling you to inspect variables, step through code, and diagnose issues interactively;
- Commands: Once in the pdb prompt, you can use various commands to navigate through the code, inspect variables, set breakpoints, and execute code snippets.
Practical Debugging Techniques
In addition to using pdb, developers can employ various techniques and best practices to debug Python scripts effectively:
- Print Statements: Inserting strategically placed print statements within the code can help track the flow of execution and identify potential issues by observing variable values;
- Logging: Utilize Python’s built-in logging module to record and analyze the behavior of your script, allowing for detailed inspection of events, errors, and variable states;
- Exception Handling: Implement robust exception handling mechanisms to gracefully handle errors and exceptions, providing meaningful error messages and ensuring the stability of your script.
Additional Debugging Tools
While pdb serves as a powerful and versatile debugger, Linux also offers a range of additional debugging tools and utilities tailored for Python development:
- pdb++: An enhanced version of pdb with additional features and improvements, providing an enhanced debugging experience;
- IDE Integration: Integrated Development Environments (IDEs) like PyCharm, VSCode, and Eclipse offer advanced debugging capabilities, including breakpoints, variable inspection, and stack tracing.
Advanced Execution: Virtual Environments and Packages
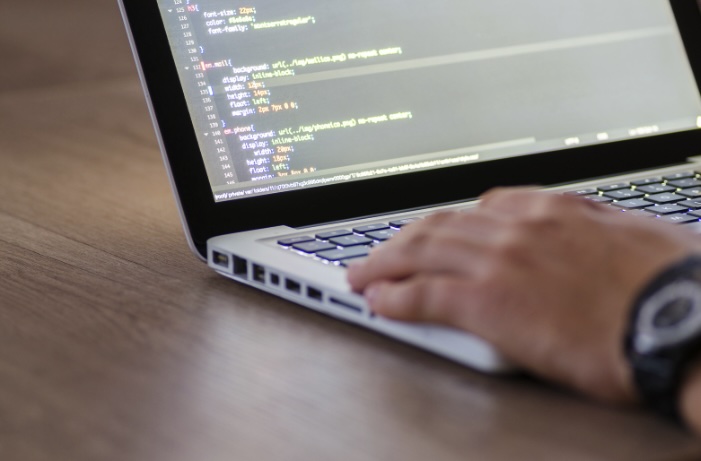
For complex Python projects, managing dependencies and isolating environments become crucial. In the Linux environment, virtual environments and package management tools like pip play pivotal roles in ensuring project integrity and scalability.
Creating a Virtual Environment
Virtual environments provide isolated environments for Python projects, allowing you to manage dependencies independently of the system-wide Python installation. Here’s how you can create and activate a virtual environment:
- Creation: Utilize the python3 -m venv myenv command to create a virtual environment named myenv. This command sets up a directory structure containing a standalone Python interpreter and a copy of the Python standard library;
- Activation: To activate the virtual environment, use the command source myenv/bin/activate. This command modifies the shell’s environment to prioritize the Python interpreter and packages within the virtual environment.
Creating and activating a virtual environment isolates your project’s dependencies, preventing conflicts with other projects or the system-wide Python installation.
Package Management with pip
pip is the de facto package installer for Python, allowing you to easily install, upgrade, and manage Python packages and dependencies. Here’s how you can leverage pip for package management:
- Installation: Use the pip install package_name command to install the desired package into your virtual environment. Replace package_name with the name of the package you wish to install;
- Dependency Resolution: pip automatically resolves and installs dependencies required by the specified package, ensuring that your project has access to all necessary libraries and modules.
pip simplifies the process of managing project dependencies, enabling seamless integration of third-party libraries and modules into your Python scripts.
Practical Application
Let’s consider a practical scenario where virtual environments and package management are essential:
- Project Development: Suppose you’re developing a web application using Django, a popular Python web framework. By creating a virtual environment specifically for your Django project and using pip to install Django and its dependencies, you ensure that your project remains self-contained and portable;
- Dependency Isolation: Each project can have its own virtual environment with its own set of dependencies, allowing for fine-grained control over package versions and preventing conflicts between projects.
Best Practices
To maximize the effectiveness of virtual environments and package management, consider the following best practices:
- Version Control: Include the requirements.txt file in your project repository to document the project’s dependencies. This file can be used to recreate the exact environment using pip install -r requirements.txt;
- Regular Updates: Periodically update packages within your virtual environment using pip install –upgrade package_name to ensure compatibility and security.
Conclusion
Executing a Python script in Linux is not just about typing a few commands. It’s about understanding your environment and the tools at your disposal. Always keep your Python version updated, write clean and understandable code, and don’t be afraid to use debugging tools to track down issues.
By following this guide on how to execute a Python script in Linux, you’re well on your way to becoming proficient in handling Python in the Linux environment. Remember, practice makes perfect, so keep experimenting with different scripts and techniques.
FAQ
Yes, but Python 2 is no longer maintained. It’s recommended to upgrade to Python 3.
Use the & at the end of the command, like python3 script.py &.
python typically refers to Python 2, while python3 is for Python 3. It’s recommended to use Python 3.
Generally, no. However, if the script needs to access restricted files or system settings, you might need sudo privileges.
Read the error messages carefully. They often provide valuable insights into what went wrong. Using a debugger can also be helpful.