Python, a highly adaptable and intuitive programming language, has amassed substantial acclaim due to its straightforwardness and capability. You have arrived at the appropriate location if you wish to acquire knowledge on formulating Python scripts. This comprehensive guide will provide a systematic explanation of the fundamentals of Python script writing, enabling even novices to commence the process with assurance.
Getting Started with Python Scripting
Verify that you have installed all of the required requirements before diving into Python scripting. You may reliably set up your environment for Python programming by following these steps:
A Computer with Python Installed
First things first, make sure your computer has Python installed. The most recent stable version of Python, 3.x, is what we advise. The official Python website makes it easy to download and install Python on any computer at python.org. This ensures that you have access to all the latest features and enhancements, providing a smooth and efficient Python scripting experience.
A Text Editor
A text editor is an essential tool for writing, editing, and saving Python scripts. Pick a text editor that suits your needs and tastes; it will have a significant impact on how you code. Here are a few well-liked choices, along with links to each:
- Notepad++ (Windows): For Windows users, Notepad++ is a lightweight and efficient text editor widely favored by programmers. It offers coding-specific features such as syntax highlighting, auto-completion, and a user-friendly interface;
- Sublime Text (Cross-platform): Sublime Text is a cross-platform text editor known for its speed and versatility. Highly customizable and supportive of various programming languages, it’s an excellent choice for Python scripting on multiple operating systems;
- Visual Studio Code (Cross-platform): Visual Studio Code (VS Code) is a popular open-source text editor developed by Microsoft. It boasts a vast ecosystem of extensions, including a robust Python extension that enhances your scripting experience with debugging capabilities, integrated terminals, and more. This makes it an excellent choice for both beginners and experienced developers.
Installation Guide
Before you can begin writing Python scripts, you need to ensure that Python is installed on your computer. Follow these steps:
Checking for the Presence of Python
Before delving into the world of Python programming, it’s crucial to ensure that Python is properly set up on your system. This initial step is essential for a seamless experience in writing and executing Python scripts. Fortunately, checking for the presence of Python on your system is a straightforward process.
Begin by opening a terminal or command prompt, depending on your operating system. Once you have a terminal or command prompt open, you can determine whether Python is already installed by entering the following command:
python –version
Upon executing this command, if Python is installed on your system, you’ll receive a prompt displaying the version number of Python. However, if Python is not detected, it’s necessary to proceed with the installation.
Downloading and Installing Python
The definitive resource for acquiring Python is the official Python website, located at python.org. The location grants you access to the most recent Python version compatible with your operating system, be it Linux, Windows, or macOS. In order to achieve a successful installation, kindly adhere to the comprehensive installation guidelines that are available on the website.
You will have a completely functional Python environment on your computer, ready to write and execute Python scripts, once the installation is complete.
Preparing Your Environment for Python Scripting
Now that Python is securely installed on your computer, the next step involves setting up your development environment for Python scripting. To begin, you’ll need to open your preferred text editor. You have the flexibility to choose any text editor you prefer, whether it’s Notepad on Windows, TextEdit on macOS, or even more sophisticated editors like Visual Studio Code. For this illustrative example, we’ll use a basic text editor to keep things simple.
Crafting Your First Python Script
With your chosen text editor at the ready, it’s time to write your inaugural Python script. In the editor, type the following straightforward Python command:
print(“Hello, World!”)
This single line of code instructs Python to output the text “Hello, Python!” to the console when the script is executed. It serves as a common introductory script in the world of programming and is often the starting point for many Python beginners.
Saving Your Python Script
Before proceeding to execute your Python script, ensure that you save it with a “.py” file extension. In our example, let’s name the file “hello.py.” This file extension informs your computer that the content of the file is Python code, allowing it to be recognized and executed as such.
Running Your Python Script
Executing your freshly crafted Python script involves a few simple steps:
- Open a terminal or command prompt on your system;
- Navigate to the directory where you saved your “hello.py” file using the cd command. For instance: cd path/to/your/script/directory;
- Run the script by typing the following command: python hello.py
Upon successful execution, you will witness the output “Hello, Python!” gracefully displayed in the terminal, marking your first accomplishment in the world of Python programming.
Understanding the Basics of Python Scripting
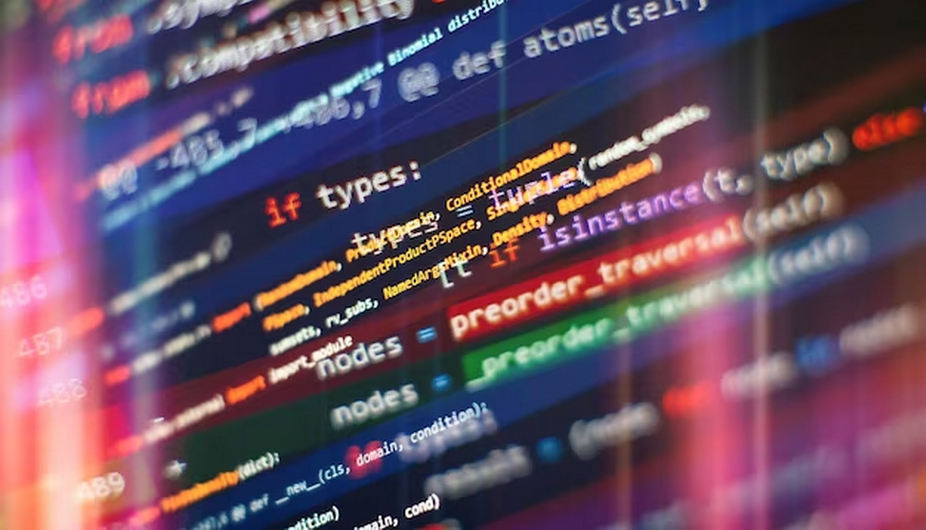
Python is renowned for its readability and simplicity, making it an excellent choice for both beginners and experienced programmers. This guide will walk you through key Python basics, including variables and data types, control structures, and functions.
Variables and Data Types
Variables in Python serve as containers for storing data. They play a pivotal role in programming, allowing you to manipulate and work with information. Python offers various data types, each tailored to accommodate specific kinds of data. Below is a brief overview of commonly used data types in Python:
Data Type | Description | Example |
int | Integer values without decimal points | age = 25 |
float | Floating-point numbers with decimals | pi = 3.14159 |
str | Strings, which represent text | name = “Python Learner” |
bool | Boolean values, True or False | is_student = True |
list | Ordered collection of items | fruits = [“apple”, “banana”, “cherry”] |
In the provided example:
name = “Python Learner”
age = 25
print(name, “is”, age, “years old.”)
We declare two variables, ‘name’ and ‘age’, with respective data types of string and integer, and then print a message that incorporates these variables.
Control Structures
Control structures are essential for adding logic and decision-making capabilities to your Python scripts. They enable your code to respond dynamically to different conditions. Two primary control structures in Python are ‘if’ statements and loops.
Example of an ‘if’ statement in a Python script:
if age > 18:
print(“Adult”)
else:
print(“Minor”)
In this code snippet, an ‘if’ statement checks whether the ‘age’ variable is greater than 18. If the condition is met, it prints “Adult”; otherwise, it prints “Minor.” This demonstrates how Python scripts can make decisions based on data and execute different code paths accordingly.
Functions
Functions are a fundamental concept in Python and most programming languages. They encapsulate reusable blocks of code, enhancing the organization and maintainability of your scripts. Functions can accept parameters (inputs), perform operations, and return results if necessary.
Defining a function in Python:
def greet(name):
print(“Hello”, name + “!”)
Using the function:
greet(“Alice”)
Here, we define a function ‘greet’ that takes a ‘name’ parameter and prints a personalized greeting. We then call the function with the argument “Alice,” resulting in the output “Hello Alice!”.
Advanced Python Scripting Concepts
After gaining a solid understanding of the fundamentals of Python scripting, it’s time to delve into more advanced concepts that will enhance your programming skills. Let’s explore three essential areas: working with libraries, error handling, and file handling. These topics are crucial for anyone looking to become proficient in Python scripting.
Working with Libraries
Python’s versatility and power are amplified by its extensive collection of libraries, which provide ready-made functions and modules for various tasks. Understanding how to leverage libraries can greatly enhance your Python scripting capabilities. Here are a few essential libraries and their common use cases:
Requests for HTTP Requests
The requests library is used to send HTTP requests and handle responses. It simplifies tasks like making GET and POST requests to web services, retrieving data from APIs, and interacting with web resources.
Example:
import requests
response = requests.get(“https://api.example.com/data”)
if response.status_code == 200:
data = response.json()
# Process the data
else:
print(“Error: Failed to fetch data”)
Pandas for Data Analysis
Pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames for handling structured data and offers tools for data cleaning, transformation, and exploration.
Example:
import pandas as pd
data = pd.read_csv(“data.csv”)
# Perform data analysis operations
Numpy for Numerical Computations
Numpy is a fundamental library for numerical and scientific computing in Python. It offers arrays and functions for performing mathematical operations efficiently.
Example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
result = np.square(arr)
Error Handling
Robust error handling is essential in Python scripting to gracefully manage unexpected situations and ensure your scripts run smoothly. The try-except block is a fundamental construct for handling exceptions.
Using try-except Blocks
A try-except block allows you to catch and handle exceptions that may occur during script execution. This prevents the script from crashing and enables you to provide meaningful error messages.
Example:
try:
# Code that might raise an exception
result = 10 / 0
except ZeroDivisionError as e:
print(f”Error: {e}”)
File Handling
Python scripts frequently interact with files, whether it’s reading data from files or writing results to them. The built-in open function is the primary tool for file handling.
Using the open Function
The open function is used to open files in different modes (e.g., read, write, append). It returns a file object that you can use to perform file operations.
Example (Reading from a File):
try:
with open(“data.txt”, “r”) as file:
content = file.read()
# Process the file content
except FileNotFoundError as e:
print(f”Error: {e}”)
Example (Writing to a File):
try:
with open(“output.txt”, “w”) as file:
file.write(“Hello, world!”)
except IOError as e:
print(f”Error: {e}”)
Tips and Best Practices
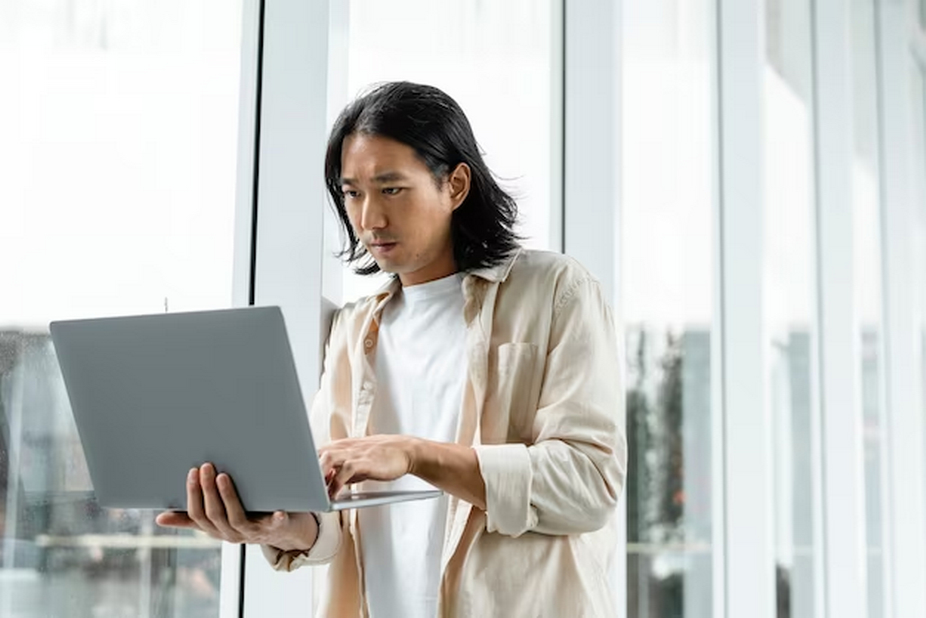
Code readability is a critical aspect of software development, especially in Python, which is known for its simplicity and readability. Writing clear and concise code enhances collaboration, maintainability, and overall code quality. Let’s explore several tips and best practices to improve code readability in Python.
Consistent Naming Conventions
One of the fundamental aspects of writing readable code is using consistent and meaningful variable and function names. Adopting a naming convention and sticking to it across your project will make your code more understandable and accessible to other developers.
Naming Type | Convention Example | Description |
Variables | word_count | Use lowercase with underscores for variables. |
Functions | calculate_area() | Use lowercase with underscores for function names. |
Constants | PI = 3.14159265 | Use uppercase letters with underscores for constants. |
Classes | CarModel | Use CamelCase for class names. |
Modules | my_module.py | Use lowercase with underscores for module names. |
Commenting
Commenting your code is essential for explaining complex logic and providing context to your codebase. Use comments to describe the purpose of functions, classes, and important sections of your code. Be concise and to the point, and make sure your comments stay up-to-date as your code evolves.
# Function to calculate the area of a circle
def calculate_area(radius):
“””
Calculate the area of a circle.
:param radius: The radius of the circle.
:return: The area of the circle.
“””
return 3.14159265 * radius ** 2
Regular Testing
Testing your Python scripts frequently is a crucial practice to catch errors and bugs early in the development process. Python offers various testing frameworks, such as unittest and pytest, to help you write and automate tests for your code. By maintaining a suite of tests, you ensure that code changes do not introduce unintended issues.
Proper Indentation and Spacing
Python relies on proper indentation to define code blocks. Use consistent indentation, typically four spaces per level, to improve code readability. This ensures that the structure of your code is visually clear. PEP 8, Python’s style guide, provides detailed guidelines on code formatting, including indentation and spacing.
def function_with_proper_indentation():
if condition:
print(“Indented correctly”)
else:
print(“Indented consistently”)
Avoid Magic Numbers
Magic numbers are hard-coded numeric values without explanation. Replace magic numbers with named constants or variables to make your code more self-explanatory.
# Magic Number
area = 3.14159265 * radius ** 2
# Improved with Constants
PI = 3.14159265
area = PI * radius ** 2
Modularize Your Code
Divide your code into smaller, logically organized modules and functions. This promotes code reusability and simplifies debugging. Each function or module should have a clear, single responsibility.
Consistent Code Style
Adhering to a consistent code style across your project is crucial for readability. PEP 8 provides comprehensive guidelines for Python code style. You can use code linters like flake8 to automatically check your code for adherence to these standards.
Conclusion
Learning how to write a Python script can be a fun and rewarding experience. With the basics covered in this guide, you’re well on your way to becoming proficient in Python scripting. Remember, practice is key, so keep experimenting with different scripts and projects to enhance your skills.
FAQ
Q: Do I need any special software to write Python scripts?
A: No, a basic text editor and Python installed on your computer are all you need to start scripting.
Q: How do I run a Python script?
A: Open a command line or terminal, navigate to the script’s directory, and type python scriptname.py.
Q: Can I write Python scripts on my smartphone?
A: While it’s more common to write scripts on a computer, there are mobile apps available that allow you to write and run Python scripts.
Q: Are there any good resources for learning how to write Python scripts?
A: Yes, numerous online tutorials, books, and courses are available to help you learn Python scripting.
Q: How important is it to follow naming conventions in Python scripting?
A: Very important. Consistent naming conventions improve readability and maintainability of your scripts.