Are you curious about how to square something in Python? If so, you’re in for a treat! Squaring numbers is a fundamental operation in mathematics and programming, and Python makes it incredibly easy and fun. In this article, we’ll explore various methods for squaring numbers in Python, ensuring you have a clear understanding of how to square something in Python by the time you reach the end.
Basic Method to Square Something in Python
There are various ways to square a number, each with its own advantages and use cases. Among these methods, the most basic approach involves utilizing the multiplication operator (*). This method is widely used due to its simplicity and efficiency, making it an excellent starting point for beginners diving into Python programming. Let’s delve deeper into this basic method and explore its intricacies.
Understanding the Multiplication Operator
The multiplication operator (*) is a fundamental arithmetic operator in Python, primarily used for performing multiplication operations. When applied to a number with itself, it effectively squares the number. This operation is intuitive and aligns with basic mathematical principles. Here’s a breakdown of how the multiplication operator works in squaring a number:
number = 4
squared_number = number * number
print(squared_number) # Outputs: 16
In this code snippet, we assign the value 4 to the variable number. By multiplying number with itself using the * operator, we obtain the square of 4, which is 16. The result is then stored in the variable squared_number and subsequently printed.
Advantages of the Basic Method
The basic method of squaring a number using the multiplication operator offers several advantages, especially for beginners:
- Simplicity and Readability: The simplicity of the multiplication operator makes the code easy to understand even for those new to programming. The straightforward syntax allows beginners to grasp the concept quickly without delving into complex algorithms or functions;
- Efficiency in Computation: The multiplication operator is highly optimized for performance in Python, ensuring efficient computation of squares. This efficiency is crucial, especially when dealing with large datasets or repetitive calculations, as it minimizes processing time and resource consumption;
- Versatility Across Data Types: This method is not limited to integers; it can be applied to floating-point numbers as well, providing versatility in handling different data types. Whether working with whole numbers or decimals, the multiplication operator delivers consistent results, making it suitable for a wide range of applications.
Exploring Practical Applications
Beyond its simplicity and efficiency, the basic method of squaring a number has practical applications across various domains:
- Mathematical Operations: Squaring numbers is a fundamental operation in mathematics, extensively used in algebra, calculus, and geometry. Python’s basic method facilitates these mathematical computations seamlessly;
- Scientific Computing: In scientific computing and data analysis, squaring numbers is commonly encountered in statistical calculations, signal processing, and numerical simulations. The efficiency of the basic method ensures swift processing of scientific data;
- Algorithmic Solutions: Many algorithms and problem-solving techniques involve squaring numbers as part of their computations. The simplicity and versatility of the basic method make it a valuable tool for implementing algorithmic solutions in Python.
Using the Exponentiation Operator
There’s an elegant alternative to squaring a number using the exponentiation operator (**). This operator is specifically designed for power calculations, making it ideal for squaring numbers effortlessly. Let’s delve into how the exponentiation operator works and explore its advantages in squaring numbers.
Understanding the Exponentiation Operator
The exponentiation operator (**) raises a number to a certain power. When applied to a number to square it, the exponent is set to 2, effectively squaring the number. Here’s how it looks in code:
number = 5
squared_number = number ** 2
print(squared_number) # Outputs: 25
In this example, the variable number is assigned the value 5. By using the exponentiation operator (**) with an exponent of 2, we square the number and store the result in the variable squared_number. Printing squared_number outputs 25, which is the square of 5.
Advantages of the Exponentiation Operator
Using the exponentiation operator for squaring numbers offers several advantages:
- Readability and Mathematical Notation: The exponentiation operator mirrors the mathematical notation for squaring, making the code more intuitive and easier to understand. This similarity to mathematical conventions enhances readability, especially for individuals with a background in mathematics;
- Conciseness and Expressiveness: The use of the exponentiation operator results in concise and expressive code. By directly specifying the exponent as 2, the intention to square the number is clear and unambiguous, reducing the need for additional comments or explanations;
- Flexibility for Other Power Calculations: While primarily used for squaring numbers, the exponentiation operator offers flexibility for performing other power calculations. By adjusting the exponent, it can be used to compute cubes, fourth powers, or any arbitrary power of a number, expanding its utility beyond simple squaring.
Practical Applications and Use Cases
The exponentiation operator finds widespread use in various applications and domains:
- Mathematical Modeling: In mathematical modeling and simulations, the exponentiation operator is essential for raising variables to specific powers, enabling the representation of exponential growth or decay phenomena;
- Engineering Calculations: Engineers often use the exponentiation operator in calculations involving physical quantities and formulas, such as determining the square area of a geometric shape or computing power requirements;
- Scientific Research: Scientists leverage the exponentiation operator for power calculations in fields such as physics, chemistry, and biology, where exponential relationships are prevalent in experimental data analysis and modeling.
Squaring Elements in a List
Squaring elements in a list is a common task, especially when working with numerical data. Python’s list comprehensions provide a concise and elegant way to square each element in a list effortlessly. Let’s delve into how list comprehensions can be utilized to square elements in a list and examine their advantages in terms of readability and efficiency.
Understanding List Comprehensions
List comprehensions are a compact and Pythonic way to create lists based on existing lists or iterables. They allow for concise expression of loops and conditional statements within a single line of code. When used to square elements in a list, list comprehensions offer a streamlined approach to performing this operation. Here’s how it’s done:
numbers = [1, 2, 3, 4]
squared_numbers = [number ** 2 for number in numbers]
print(squared_numbers) # Outputs: [1, 4, 9, 16]
In this example, the list numbers contain the elements [1, 2, 3, 4]. By utilizing a list comprehension, each element in numbers is squared individually, and the results are stored in the list squared_numbers. Printing squared_numbers yields [1, 4, 9, 16], which corresponds to the square of each element in the original list.
Advantages of List Comprehensions
List comprehensions offer several advantages when squaring elements in a list:
- Conciseness and Readability: List comprehensions provide a concise and readable syntax for expressing operations on lists. By encapsulating the squaring operation within a single line of code, list comprehensions enhance code readability and maintainability, especially for simple transformations like squaring elements;
- Efficiency and Performance: List comprehensions are optimized for performance in Python, often outperforming traditional loop-based approaches in terms of speed and resource consumption. This efficiency is particularly beneficial when working with large datasets or performing repetitive operations, as it minimizes computation time and memory overhead;
- Expressiveness and Pythonic Style: List comprehensions embody the Pythonic philosophy of writing clean, expressive, and idiomatic code. They align with the language’s emphasis on simplicity and readability, making code more understandable to both beginners and experienced Python developers.
Practical Applications and Use Cases
The application of list comprehensions extends beyond squaring elements in a list to various data manipulation tasks:
- Data Processing: List comprehensions are widely used in data processing pipelines to transform and filter lists of data efficiently. They facilitate tasks such as mapping, filtering, and aggregation with minimal code overhead;
- Numerical Computing: In numerical computing and scientific computing, list comprehensions are valuable for performing element-wise operations on arrays or vectors. They enable rapid computation of mathematical functions and transformations across large datasets;
- Text Processing: List comprehensions find applications in text processing tasks, such as tokenization, normalization, and feature extraction. They provide a succinct and expressive way to manipulate lists of strings or characters.
Squaring with a Function
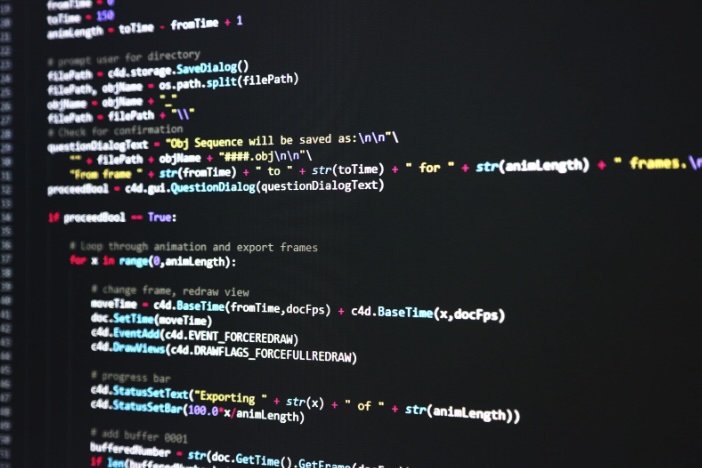
Creating a function to square numbers is a fundamental aspect of programming, particularly when you find yourself needing to square numbers frequently. This approach enhances code organization, reusability, and modularity. Let’s delve into how to define and use a function to square numbers and explore its benefits in terms of readability and efficiency.
Understanding Functions in Python
Functions in Python are blocks of code that perform a specific task and can be reused multiple times throughout a program. They are defined using the def keyword followed by the function name, parameters (if any), and a block of code to execute. Here’s how a function to square a number is defined:
def square(number):
return number ** 2
print(square(6)) # Outputs: 36
In this example, the square function takes a single parameter number and returns the square of that number using the exponentiation operator (**). When calling the function with the argument 6, it returns 36, which is the square of 6.
Advantages of Using a Function
Creating a function to square numbers offers several advantages:
- Reusability and Modularity: Functions promote code reusability by encapsulating a specific task (squaring numbers, in this case) into a reusable unit. Once defined, the function can be called multiple times with different arguments, eliminating the need to rewrite the squaring logic each time it’s needed. This enhances code modularity and reduces redundancy;
- Readability and Maintainability: Using a function improves code readability by providing a descriptive name (square) that clearly communicates its purpose. This makes the code easier to understand for both the original developer and other programmers who may review or collaborate on the codebase. Additionally, functions enable better code organization, separating different functionalities into distinct units for easier maintenance and troubleshooting;
- Flexibility and Extensibility: Functions offer flexibility in terms of parameterization, allowing for variations in input parameters and return values. Additionally, functions can be extended or modified to accommodate additional functionality or handle different use cases. This adaptability makes functions versatile tools for solving a wide range of problems in Python programming.
Practical Applications and Use Cases
The use of functions for squaring numbers extends beyond simple arithmetic operations to various programming tasks:
- Numerical Computations: Functions are essential for performing mathematical computations and transformations in scientific computing, data analysis, and engineering applications. They enable efficient handling of numerical data and facilitate the implementation of complex algorithms involving squaring operations;
- Software Development: In software development, functions play a crucial role in structuring code, promoting code reuse, and enhancing maintainability. They facilitate the development of modular, scalable, and maintainable software systems by encapsulating specific functionalities into reusable components;
- Educational Purposes: Functions are valuable teaching tools for introducing programming concepts and techniques, such as abstraction, encapsulation, and code organization. They provide a practical framework for demonstrating fundamental programming principles and best practices.
Utilizing the Math Library
Python’s math library provides a comprehensive set of functionalities to handle such tasks efficiently. While the math library doesn’t offer a direct method for squaring numbers, it does provide other related functionalities that can be leveraged. Let’s delve into how to utilize Python’s math library to square numbers and explore its capabilities in floating-point arithmetic.
Understanding Python’s Math Library
Python’s math library is a built-in module that provides a wide range of mathematical functions and constants for performing various mathematical operations. These functions cover a broad spectrum of mathematical domains, including algebra, calculus, trigonometry, and statistics. To utilize the math library, it must be imported into the Python script using the import statement. Here’s how to use the math library to square a number:
import math
number = 8
squared_number = math.pow(number, 2)
print(squared_number) # Outputs: 64.0
In this example, the math.pow() function is used to compute the square of the number 8. The first argument is the base (number), and the second argument is the exponent (2). The result, 64.0, is a floating-point number representing the square of 8.
Advantages of Using Python’s Math Library
Although Python’s math library doesn’t offer a dedicated function for squaring numbers, it provides several advantages:
- Versatility in Mathematical Functions: The math library offers a wide range of mathematical functions beyond basic arithmetic operations, including exponentiation, logarithms, trigonometric functions, and more. This versatility enables programmers to perform complex mathematical computations with ease;
- Precision in Floating-Point Arithmetic: Mathematical operations performed using the math library are optimized for precision, especially in floating-point arithmetic. By utilizing floating-point numbers, the math library ensures accurate representation of numerical values, essential for scientific computing and numerical analysis;
- Consistency with Mathematical Notation: Functions in the math library often mirror mathematical notation, making it easier for users familiar with mathematical conventions to understand and use them. This consistency enhances code readability and promotes clarity in expressing mathematical concepts and algorithms.
Practical Applications and Use Cases
Python’s math library finds applications in various domains, including:
- Scientific Computing: In scientific computing and engineering applications, the math library is indispensable for performing complex mathematical computations involving functions such as exponentiation, logarithms, and trigonometry. It facilitates numerical simulations, data analysis, and modeling tasks with precision and efficiency;
- Financial Calculations: The math library is utilized in financial calculations, such as compound interest calculations, present value computations, and risk assessments. Its precise arithmetic operations ensure accurate results in financial modeling and analysis;
- Statistical Analysis: For statistical analysis and data science tasks, the math library provides functions for calculating probabilities, distributions, and descriptive statistics. It supports various statistical techniques and algorithms for exploring and analyzing datasets effectively.
Squaring Numbers: Different Scenarios
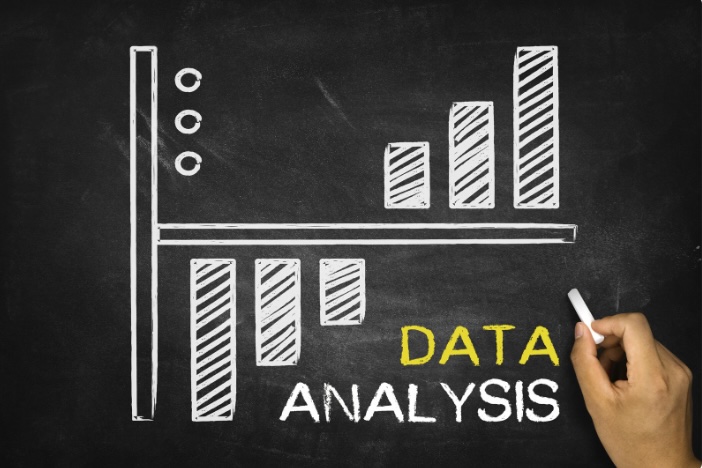
Knowing how to square something in Python is particularly useful in different scenarios:
Data Analysis
In data analysis, squaring numbers plays a crucial role in statistical calculations and data transformations. Some key scenarios where squaring numbers are utilized include:
- Variance Calculation: Squaring deviations from the mean is a fundamental step in calculating variance, a measure of the spread of data points in a dataset;
- Standard Deviation: Standard deviation, another important measure of dispersion, involves squaring deviations from the mean and then taking the square root of the sum;
- Regression Analysis: Squaring independent variables is common in regression analysis, where squared terms are included to model nonlinear relationships between variables.
Game Development
In game development, squaring numbers is frequently used for various calculations and simulations, contributing to the immersive experience of gaming. Some scenarios where squaring numbers are utilized include:
- Distance Calculations: Squaring the distance between two objects is often required for collision detection, determining proximity, or calculating line-of-sight visibility;
- Animation Effects: Squaring time or position values can create interesting animation effects, such as accelerating or decelerating motion, or creating parabolic trajectories;
- Physics Simulations: Squaring velocities or accelerations is common in physics simulations within games to model realistic motion and interactions between objects.
Scientific Computing
In scientific computing, squaring numbers is essential for performing complex calculations and simulations across various scientific disciplines. Some scenarios where squaring numbers are utilized include:
- Numerical Methods: Squaring numbers is a fundamental operation in numerical methods such as finite difference methods, finite element methods, and numerical integration techniques;
- Signal Processing: Squaring signals is often used in signal processing applications, such as power calculations, energy measurements, and spectral analysis;
- Quantum Mechanics: Squaring complex wave functions or probability amplitudes is central to many calculations in quantum mechanics and quantum computing.
Conclusion
Understanding how to square something in Python is a basic yet powerful skill. Whether you’re a beginner or an experienced coder, squaring numbers is a fundamental operation that you’ll find useful in various programming tasks. By following the methods and examples provided in this article, you’ll be well on your way to mastering how to square something in Python, enhancing both your mathematical and programming capabilities.
Remember, practice makes perfect, so don’t hesitate to try these methods out and experiment with squaring different types of numbers.
FAQ
Absolutely! Squaring a negative number in Python follows the same methods and will result in a positive number, as the negative sign is negated during the squaring process.
Yes, you can square floats, and even complex numbers in Python using the same methods.
NumPy, a library for numerical operations, provides vectorized operations to square arrays efficiently, which is incredibly useful in data science and machine learning.