Encountering the “length of values does not match length of index” error in Python can be a bit of a headache, especially for those new to coding. This error typically arises when working with data structures like lists and Pandas DataFrames. But fear not! This article is your guide on how to fix this error in Python and ensure a smooth coding experience.
Understanding the Error
Before delving into potential solutions, it’s essential to grasp the meaning behind the error message “length of values does not match length of index” in Python. This error typically arises when attempting to assign a list of values to either a DataFrame or a Series, and the number of elements in the provided list does not align with the number of rows or columns in the DataFrame.
To illustrate, imagine a DataFrame containing 5 rows. If an attempt is made to assign a list with 4 or 6 elements to one of its columns, Python will raise this error.
Potential Causes
Several common scenarios can lead to encountering this error:
- Mismatched Lengths: The most straightforward cause is providing a list with a different number of elements compared to the DataFrame’s number of rows or columns;
- Incorrect Indexing: An indexing error might occur where the provided list is supposed to match the DataFrame’s index or column labels but fails to do so;
- Data Cleaning Issues: In some cases, discrepancies in data cleaning or preprocessing steps can result in a mismatch between the expected and actual lengths of the data being assigned;
- Data Import Errors: When importing data from external sources, inconsistencies in data formatting or unexpected changes in the dataset structure might lead to this error.
Solutions
To resolve the “length of values does not match length of index” error, consider the following solutions:
- Verify Data Integrity: Double-check the integrity of the data being assigned to the DataFrame or Series. Ensure that the length of the list matches the number of rows or columns it is intended to fill;
- Review Data Structures: Confirm that the indexing aligns correctly between the DataFrame and the provided list. Ensure that index labels or column names match accordingly;
- Data Cleaning: Review any data cleaning or preprocessing steps performed prior to assigning values to the DataFrame. Ensure that these steps are consistent and do not inadvertently alter the data dimensions;
- Debug Import Processes: If the error occurs during data import, thoroughly review the data source and import processes for any inconsistencies or errors in data formatting;
- Utilize Appropriate Functions: Make use of appropriate DataFrame or Series methods for assigning values, such as .loc[] or .iloc[], to ensure proper alignment with DataFrame dimensions.
Example Code
Consider the following example demonstrating how to assign values to a DataFrame without encountering the “length of values does not match length of index” error:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3, 4, 5]})
# Assign values to a new column
new_column_values = [10, 20, 30, 40, 50]
df['B'] = new_column_values
In this example, the length of new_column_values matches the number of rows in the DataFrame, preventing any errors during an assignment.
Common Scenarios Leading to the Error
Encountering errors is a common occurrence. One such error that frequently arises is the “Length Mismatch Error.” Understanding the scenarios that commonly lead to this error is crucial for effectively troubleshooting and preventing it. Below are the three primary scenarios where this error typically occurs:
- Data Importing: When importing data from various sources, mismatches in expected row counts can occur. This discrepancy often arises due to differences in data formats, missing values, or inconsistencies in the data structure. For instance, importing CSV files with missing or extra rows, or importing data from databases where the expected row count does not match the actual count, can lead to a length mismatch error;
- Data Manipulation: Data manipulation operations frequently involve altering the length of data structures, such as appending data to a list or DataFrame. Any inconsistency in the dimensions or lengths of the data being manipulated can lead to a length mismatch error. This scenario commonly occurs when attempting to concatenate or merge data structures of different lengths, resulting in inconsistencies that trigger the error;
- Merging Data: Merging datasets is a fundamental aspect of data analysis, but it can also be a source of errors. Combining datasets with different lengths can result in a length mismatch error. This situation typically arises when performing operations like inner, outer, left, or right joins on DataFrames, where the lengths of the merging columns do not align correctly.
To better understand these scenarios, consider the following examples:
Example | Description |
---|---|
Data Importing | Suppose you’re importing data from multiple CSV files into a DataFrame. One of the files contains an extra row compared to the others. When you attempt to concatenate these DataFrames, a length mismatch error occurs due to the mismatch in row counts. |
Data Manipulation | You have a list of customer IDs and a corresponding list of transaction amounts. When attempting to create a DataFrame by combining these lists, you accidentally truncate one of the lists, resulting in a length mismatch error. |
Merging Data | You’re merging two DataFrames on a common key, such as customer ID. However, one of the DataFrames contains duplicate entries for certain IDs, leading to discrepancies in row counts and triggering a length mismatch error during the merge operation. |
Checking Data Lengths
When encountering errors related to data structures in Python, such as mismatches between the length of lists or dataframes, it’s crucial to perform thorough checks to identify and rectify the issue. This guide outlines the steps to verify data lengths and offers a solution to handle discrepancies effectively.
Verifying Data Lengths
The initial step involves confirming that the number of elements in your list or any iterable corresponds to the number of rows or columns in your DataFrame. This can be achieved by using the len() function to determine the length of both the list and the DataFrame.
# Example: Checking lengths
length_of_list = len(your_list)
length_of_dataframe = len(your_dataframe)
if length_of_list == length_of_dataframe:
# Proceed with your operation
pass
else:
# Handle the mismatch
print("Error: Length mismatch between list and DataFrame.")
Handling Length Mismatch
If the lengths of the list and the DataFrame do not match, it’s essential to address this discrepancy to prevent errors and ensure accurate data processing. Here are some strategies to handle length mismatches effectively:
- Logging and Error Reporting: Implement logging mechanisms to record the occurrence of length mismatches. This facilitates tracking and debugging of issues during runtime;
- Data Trimming or Padding: If the difference in lengths is insignificant and the data integrity can be maintained, consider trimming or padding the data to align the lengths;
- Data Validation: Prioritize data validation procedures to identify inconsistencies or anomalies in the dataset. This helps in detecting potential issues early on and ensures data integrity;
- Data Cleaning: Perform data cleaning operations to eliminate redundant or erroneous entries that may contribute to length discrepancies.
Implementing Error Handling:
To handle length mismatches gracefully and maintain the robustness of your Python code, incorporate error-handling mechanisms. This involves using try-except blocks to catch exceptions and executing appropriate error-handling routines.
try:
# Perform data processing operations
# ...
except LengthMismatchError as e:
# Handle length mismatch error
print(f"Error: {e}")
except Exception as e:
# Handle other exceptions
print(f"Error: {e}")
Reshaping Data Appropriately
Reshaping data is essential to ensure compatibility between different data structures and facilitate seamless analysis and manipulation. Here, we’ll explore two methods for reshaping data in Python: Pandas reindex and list slicing.
Pandas reindex
Pandas, a powerful data manipulation library in Python, provides the reindex method to conform a DataFrame to a new index, with optional filling logic. This method is particularly useful when you need to realign the rows or columns of a DataFrame according to a new set of labels or indices.
# Example: Using Pandas reindex
your_dataframe = your_dataframe.reindex(range(len(your_list)))
Key Points about Pandas Reindex:
- Index Realignment: The reindex method realigns the DataFrame’s index to match the provided range, ensuring consistency with the length of the list;
- Filling Logic: Optionally, you can specify filling logic to handle missing values that may arise due to index realignment;
- Data Preservation: Despite index manipulation, Pandas ensures that the original data integrity is preserved, maintaining the association between index labels and corresponding data.
List slicing:
List slicing is a fundamental technique in Python for extracting a portion of a list. When reshaping data, list slicing can be employed to adjust the size of a list to match the size of a DataFrame. This ensures that the data in the list aligns appropriately with the DataFrame for subsequent operations.
# Example: Using list slicing
your_list = your_list[:len(your_dataframe)]
Key Points about List Slicing:
- Size Adjustment: List slicing enables you to resize the list by truncating or extracting elements based on the length of the DataFrame. This ensures that the length of the list matches the size of the DataFrame;
- Efficiency: List slicing operations in Python are efficient and performant, making them suitable for reshaping data without significant overhead;
- Data Alignment: By adjusting the size of the list to match the DataFrame, data alignment is maintained, facilitating seamless data processing and analysis.
Utilizing DataFrame Operations
Pandas, a powerful data manipulation library in Python, provides various functions to effectively manage DataFrame sizes. Understanding how to leverage these operations is crucial for efficiently handling data within your projects or analyses. Let’s delve into three essential DataFrame operations: .assign(), .drop(), and .fillna().
.assign() Function
The .assign() function enables users to add new columns to a DataFrame safely. This function returns a new DataFrame with the added columns without modifying the original DataFrame. Here’s how you can use it:
import pandas as pd
# Sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3],
'B': [4, 5, 6]})
# Adding a new column 'C' using .assign()
df_new = df.assign(C=[7, 8, 9])
print("Original DataFrame:")
print(df)
print("\nDataFrame after adding column 'C':")
print(df_new)
In this example, a new column ‘C’ is added with values [7, 8, 9] to the DataFrame df.
.drop() Function
The .drop() function is used to remove rows or columns from a DataFrame based on specified labels. This function returns a new DataFrame with the specified rows or columns removed, leaving the original DataFrame unchanged. Here’s how you can utilize it:
# Dropping column 'B' using .drop()
df_new = df.drop(columns=['B'])
print("Original DataFrame:")
print(df)
print("\nDataFrame after dropping column 'B':")
print(df_new)
In this example, the column 'B' is dropped from the DataFrame df, resulting in the DataFrame df_new without the 'B' column.
.fillna() Function
The .fillna() function is employed to fill missing values within a DataFrame. This function is particularly useful after resizing operations to handle any resulting missing data. Here’s a demonstration:
# Introducing missing values
df.loc[1, 'B'] = pd.NA
# Filling missing values using .fillna()
df_filled = df.fillna(0)
print("Original DataFrame:")
print(df)
print("\nDataFrame after filling missing values:")
print(df_filled)
In this example, a missing value (pd.NA) is introduced to the DataFrame df. The .fillna() function fills this missing value with 0, resulting in the DataFrame df_filled.
Error Prevention Techniques
Error prevention is a crucial aspect of data management and software development. By implementing effective techniques, you can minimize the occurrence of errors and ensure the reliability of your systems. Let’s explore some key strategies for error prevention:
Consistent Data Sources
Maintaining consistency in data sources is fundamental to preventing errors. Inconsistencies in row counts can lead to data corruption and inaccuracies in analysis. Here’s how you can ensure consistency:
- Data Profiling: Conduct thorough data profiling to identify inconsistencies in row counts across different data sources;
- Standardization: Standardize data formats and structures across all data sources to avoid discrepancies;
- Validation Rules: Define validation rules to enforce consistent data entry and processing.
Regular Checks
Regular checks are essential to detect errors early in the data manipulation process. By implementing frequent size checks, you can identify anomalies and discrepancies before they escalate. Consider the following techniques:
- Automated Scripts: Develop automated scripts to perform size checks at regular intervals during data manipulation tasks;
- Threshold Monitoring: Set thresholds for acceptable row counts and trigger alerts when deviations occur beyond predefined limits;
- Logging Mechanisms: Implement logging mechanisms to record size discrepancies and track changes over time.
Use of Assertions
Assertions play a vital role in validating assumptions and detecting errors in code. By incorporating assertions into your codebase, you can proactively identify size mismatches and prevent potential issues. Here are some effective practices:
- Precondition Assertions: Include precondition assertions to validate input data before processing, ensuring compatibility with expected row counts;
- Postcondition Assertions: Add postcondition assertions to verify the output data against predefined criteria, confirming the integrity of the manipulation process;
- Error Handling: Implement error-handling mechanisms to gracefully handle assertion failures and provide informative feedback to users.
Advanced Solutions
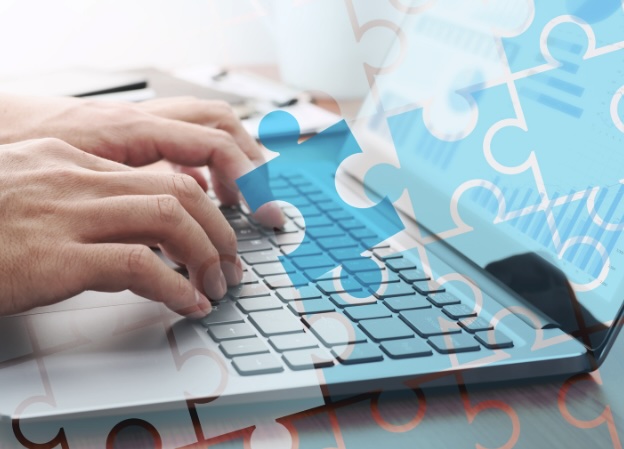
In intricate and demanding scenarios, advanced solutions are necessary to tackle challenges effectively. By employing custom functions and robust error-handling mechanisms, you can address complex issues with finesse and precision.
Custom Functions
Custom functions offer tailored solutions to specific requirements, allowing for seamless adaptation to diverse data scenarios. These functions can be designed to automate size adjustments before assigning values, ensuring compatibility and consistency.
- Dynamic Resizing: Develop custom functions capable of dynamically resizing data structures based on input parameters and requirements;
- Parameterized Inputs: Incorporate parameterized inputs into custom functions to accommodate varying data sizes and formats;
- Conditional Logic: Implement conditional logic within custom functions to intelligently adjust sizes based on predefined conditions and criteria.
Function Name | Description |
---|---|
resize_array() | Automatically adjusts the size of an array before assignment. |
resize_dataframe() | Dynamically resizes DataFrame columns based on input parameters. |
resize_matrix() | Custom function to resize matrices to match specified dimensions. |
Error Handling
Error handling is crucial in mitigating risks and ensuring the robustness of software systems. Utilizing try-except blocks enables graceful handling of errors, preventing program crashes and maintaining user satisfaction.
- Exception Handling: Employ try-except blocks to anticipate potential errors and gracefully handle them during execution;
- Error Logging: Integrate error logging mechanisms to capture and document exceptions for troubleshooting and analysis;
- Fallback Strategies: Implement fallback strategies within try-except blocks to provide alternative paths of execution in case of errors.
Code Example | Description |
---|---|
python try:<br> # Code block with potential error<br>except Exception as e:<br> # Handle the error gracefully<br> log_error(e)<br> # Perform fallback actions<br> | Example of using try-except block for error handling in Python. |
try {<br> // Code block with potential error<br>} catch (Exception e) {<br> // Handle the error gracefully<br> logError(e);<br> // Perform fallback actions<br>} | Example of using try-catch block for error handling in Java. |
Conclusion
Fixing the “length of values does not match length of index” error in Python involves a thorough understanding of your data structures and ensuring that the size of the data you are working with matches the target DataFrame or Series. By employing methods such as checking data lengths, reshaping data, utilizing DataFrame operations, and implementing error prevention techniques, you can effectively address this common issue. Remember, regular data audits, a clear understanding of your data’s structure, and maintaining a log of errors and solutions are key practices that not only help in resolving this error but also enhance your overall proficiency in data handling and analysis in Python.
FAQ
It means the number of elements you’re trying to assign to a DataFrame or Series does not match its size.
Ignoring it might lead to incomplete or incorrect data analysis. It’s best to resolve it.
It’s most common with Pandas but can occur with other data structures as well.