Welcome to the world of Python, where solving puzzles is part of the fun! Today, we’re diving into how to find the largest number in a list, a common task that often pops up in coding challenges. But wait, there’s more! We’ll also explore how to remove numbers from a string and how to add a number to a variable in Python, turning you into a Python wizard in no time!
Finding the Largest Number in a List
When working with Python, you may often encounter situations where you need to find the largest number in a list of values. Python provides multiple methods to accomplish this task. In this educational guide, we will explore two primary approaches: using the built-in max() function and a do-it-yourself (DIY) approach involving a loop. We will discuss the advantages and disadvantages of each method and provide code examples for better understanding.
Using the max() Function
The most straightforward and efficient way to find the largest number in a list is by utilizing Python’s built-in max() function. This function is purpose-built to identify the maximum value within an iterable, such as a list. Here’s how you can use it:
my_list = [3, 7, 2, 5, 6] largest_number = max(my_list) print(“The largest number is:”, largest_number) |
The max() function takes an iterable (in this case, my_list) as its argument and returns the largest value found in the list. It simplifies the process of finding the maximum value and is highly efficient for large datasets.
DIY Approach: Loop Through the List
If you prefer a more hands-on approach or want to understand the underlying mechanics of finding the largest number, you can implement your own solution using a loop. Here’s how it can be done:
my_list = [3, 7, 2, 5, 6] largest_number = my_list[0] for number in my_list: if number > largest_number: largest_number = number print(“The largest number is:”, largest_number) |
In this DIY approach, we initialize largest_number with the first element of the list and then iterate through the list, comparing each element with the current largest_number. If an element is greater, it replaces the current largest_number. This process continues until we’ve checked all elements in the list.
A Comparison of Methods
Now that we’ve explored both methods, let’s compare them to better understand when to use each:
Aspect | Using max() Function | DIY Approach: Loop |
Ease of Use | Extremely simple | Requires manual iteration |
Efficiency | Highly efficient | May be less efficient for large lists |
Code Readability | Concise and clear | More verbose and detailed |
Understanding Process | May not provide insight into the algorithm | Offers a deeper understanding of the process |
When to Use Each Method
- Use max() Function: If you need a quick and efficient solution to find the largest number in a list without delving into the underlying logic, the max() function is the preferred choice. It is especially beneficial for large datasets where performance is crucial;
- Use DIY Approach with a Loop: If you want to gain a deeper understanding of how the largest number is determined and are interested in manual coding, the DIY approach with a loop is suitable. This method also allows for customization and modification of the process if needed.
Removing Numbers from a String in Python
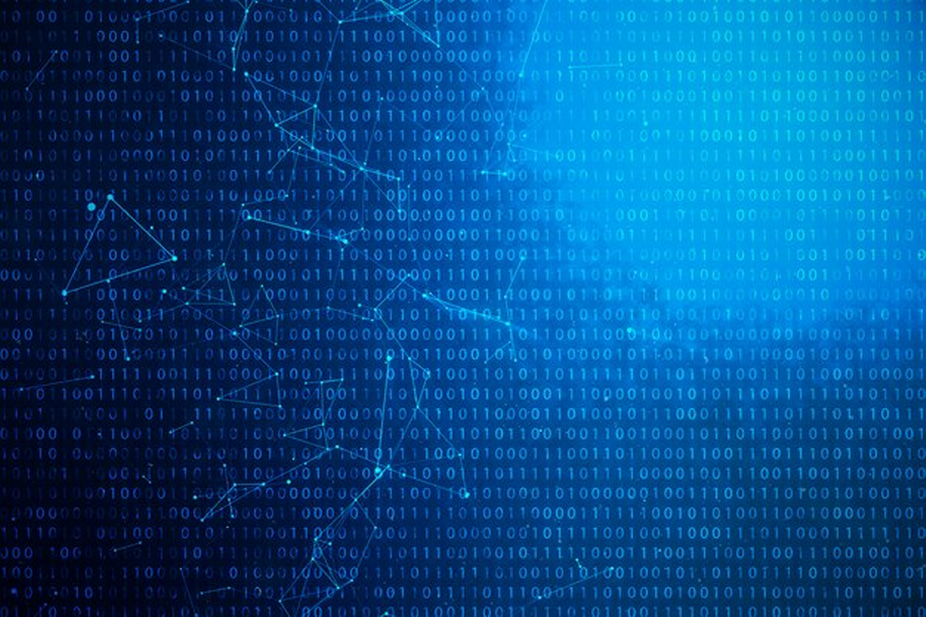
In various programming tasks, you might encounter scenarios where you need to clean up a string by removing numerical digits. Whether you are processing user inputs, parsing text data, or transforming strings for specific purposes, knowing how to efficiently remove numbers from a string in Python is a valuable skill. Let’s explore two primary methods to achieve this: using regular expressions and employing an iterative approach.
Regular Expressions to the Rescue
Regular expressions, often abbreviated as “regex,” are a powerful tool for pattern matching and text manipulation in Python. Removing numbers from a string is straightforward using the re module, which provides support for regular expressions. Here’s how you can accomplish this task with regular expressions:
import re my_string = “Python 3 is more popular than Python 2” clean_string = re.sub(r’\d+’, ”, my_string) print(clean_string) |
Explanation:
- import re: Import the re module, which allows you to work with regular expressions;
- re.sub(r’\d+’, ”, my_string): Use the re.sub() function to replace all occurrences of one or more digits (\d+) in my_string with an empty string ”.
This method efficiently removes all numerical digits from the input string, leaving you with a clean version of the text.
Iterative Approach
Alternatively, you can remove numbers from a string by iterating through the characters of the string and selectively appending non-digit characters to a new string. This approach is more manual but gives you greater control over the process. Here’s how you can do it:
my_string = “Python 3 is more popular than Python 2” clean_string = ”.join([char for char in my_string if not char.isdigit()]) print(clean_string) |
Explanation:
- my_string = “Python 3 is more popular than Python 2”: Define the input string;
- ”.join([char for char in my_string if not char.isdigit()]): Iterate through each character (char) in my_string using a list comprehension. If the character is not a digit (checked using char.isdigit()), it is included in the list. Then, join() is used to concatenate the selected characters into a single string.
This method offers more granular control and customization options, making it suitable for specific scenarios where you need to preserve certain characters or handle special cases.
A Comparison of Methods
Let’s compare the two methods to help you decide which one to use:
Aspect | Regular Expressions | Iterative Approach |
Ease of Use | Simple and concise | Requires manual coding |
Efficiency | Highly efficient | May be less efficient for very long strings |
Customization and Control | Limited | High degree of control |
Readability of Code | Clear and concise | More verbose and detailed |
Handling Special Cases | Limited flexibility | Greater flexibility |
When to Use Each Method
- Use Regular Expressions: When you want a quick and efficient way to remove numbers from a string, especially for large strings where performance matters, regular expressions are an excellent choice. They provide a concise solution without the need for detailed manual coding;
- Use Iterative Approach: If you need more control over the process, such as preserving specific characters or handling special cases, the iterative approach is a better fit. It allows you to fine-tune the removal process according to your requirements.
Adding a Number to a Variable in Python
The Basics
Adding a number to a variable is one of the fundamental operations in Python. It’s straightforward and an essential part of manipulating data.
number = 10 number += 5 # This is the same as number = number + 5 print(“Updated number:”, number) |
In the Context of a Loop
This concept often comes up in loops where you increment or update a variable:
number = 0 for i in range(5): number += i # Adds the current value of i to number print(“Current number:”, number) |
Conclusion
Congratulations! You’ve not only learned how to find the largest number in a list in Python but also mastered how to remove numbers from a string and how to add a number to a variable. These skills are crucial in your Python journey, providing a strong foundation for tackling more complex problems.
Remember, practice is key. Try out these techniques, experiment with variations, and watch as your Python skills grow.
FAQ
Q1: Can I use the max() function with a list of strings?
A1: Yes, max() works with lists of strings too! It finds the ‘largest’ string based on alphabetical order.
Q2: What happens if my list is empty when I use max()?
A2: You’ll get a ValueError. To handle this, you can check if the list is empty before using max().
Q3: Are there other ways to remove numbers from a string in Python?
A3: Yes, besides regular expressions and iterative methods, you can also use list comprehensions or filter functions.
Q4: Is it possible to add non-integer numbers to a variable?
A4: Absolutely! You can add floats, and even in some contexts, strings and other data types to a variable in Python.
Q5: What if I want to find the smallest number in a list?
A5: Use the min() function, which works similarly to max() but finds the smallest value.