One of the basic functionality required from you when mastering Python would be the knowledge of how to exit a while loop. This article is a beginner’s guide on fundamentals of while loops and the correct way of applying them. From various methods, to erroneous movements, and the ground rule for while loops, we will take you through all of these, and equip you with the confidence you need to use while loops in your adventures with Python as your companion.
The Basics of While Loops in Python
A while loop in a Python language can be used as one of the most fundamental control flow expressions for iterating a piece of code as the specified condition of repetition is true. This feature is a great advantage and allows one to handle the situation if the number of iterations is either unknown or determined by the particular condition. The while loop structure and basics of its usage are of pivotal importance for any Python coder in the field of writing quality code. Let’s consider a simple example to illustrate the usage of a while loop:
count = 0
while count < 5:
print("Count is:", count)
count += 1
In this example:
- We start with a counter that is initially equal to zero;
- The condition count < 5 is maintained which involves execution of the loop as long as the condition is true;
- Thanks to this iteration, count’s current version is printed and incremented by 1 each time;
- This point comes when count holds the value of 5 or more the condition count < 5 is converted to false, and the loop stops to be executed further.
Key Concepts and Best Practices
Efficiency and absence of mistakes are the fundamental idea of a nice while loop in Python. On the one hand, the main rule of while loops is the comprehension of each element, and, on the other hand, those rules should be followed. Let’s delve into these concepts and practices in detail:
Initialization
Before proceeding to a while loop, it is very important to initially assign any variables included in the loop condition, so as to ensure it runs specifically as envisaged. This will be programmed as the first line in the loop so that the condition can be evaluated right from the starting point. If a variable is not initialized, a program’s behavior may exhibit illegal conditions or unintended outputs. Initialized correctly is particularly counted when the loop condition responds to the value of such variables.
count = 0 # Initialization of loop control variable
while count < 5:
print("Count is:", count)
count += 1
Updating Loop Variables
Therefore, within the loop one must adjust any control variables correctly to avoid creating the infinite loops. Omitting loop variables update can result in an infinite loop that could lock up your system resource and result in the crash of your program. The loop variables are supposed to have their values updated according to special conditions or carrying out mathematical operations.
count = 0
while count < 5:
print("Count is:", count)
count += 1 # Update loop control variable
Termination Condition
Constantly makes sure that the loop’s termination condition will eventually become false. A condition that never stops evaluating to false causes an infinite loop, which results in system instability or even system down. Designing termination conditions that depict termination correctly and avoiding an infinite loop is equally important as the rest of your program logic.
total = 0
while total < 100:
total += 10 # Update loop control variable
print("Total is:", total)
Use of Break and Continue
The break command makes it possible to end the current loop before its time, while the continue command jumps over the remaining actions of others and moves to the next iteration. These statements support the management of the internal code’s flow. Besides, they can be used purposefully to streamline and improve the code’s readability and resourcefulness. On the other hand, the overuse of break and continue is a thing to prevent because it impairs the readability and maintainability of a code, so it must be applied when necessary but not for the sake of expressiveness.
while True:
user_input = input("Enter a number (type 'exit' to quit): ")
if user_input == 'exit':
break # Exit loop if user inputs 'exit'
elif user_input.isdigit():
print("You entered:", user_input)
else:
continue # Skip iteration if user input is not a number
Structure of a While Loop
In Python, a while loop adheres to a straightforward structure:
while condition:
# Code to execute
Through this syntax, the keyword is identified as the starting point of the loop, and the condition defines whether the loop should go through iterations. As long as the status of the statement is True, the block of code is executed within the loop. After the if statement is False, the loop will end and the next statement will be the one executing after the loop.
Why Use While Loops?
While loops are the most important tools in Python programming as they are universal and suitable for processing of single or multiple instances of repetitive operations. Here are some common scenarios where while loops prove beneficial:
- Repetitive Tasks: While loops auto-perform repetitive actions by providing a condition that regulates how the repetition is carried out. This ability enables execution of the same block of code until the developer’s specified condition is fulfilled. Whether you are traversing through a list, or carrying out calculations iteratively, while loop is a general and flexible program structure especially beneficial;
- User Input Processing: The while loop is popular in cases such as this to continue to prompt a user for input until a valid response is provided. With the use of this feature, the interaction between the users and the program is increased, which consequently improves the usability and durability of the program. Through the use of while loops, programmers can efficiently and repeatedly process the user input, which is important, especially in dealing with invalid or unexpected feedback;
- Real-Time Monitoring: The while loops are fundamental in data monitoring applications that need real-time data updates or event-driven systems. API gateways play a key role in programs that provide an immediate environment to continuously monitor data streams or events and updates as conditions change. It may involve checking sensor values, network activities, or human interface through a GUI loop. Hence, loops are there to help you handle various situations by providing the best solution each time.
Ending a While Loop – The Condition Approach
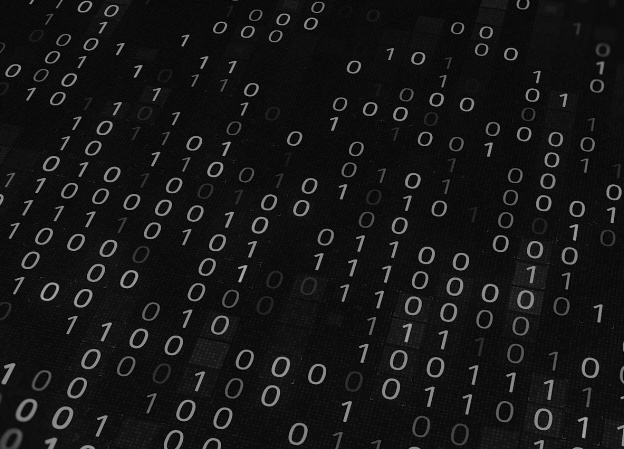
One of the simplest I could say is the condition approach for ending a while loop inside Python. It is done by alternating the loop’s condition from the initial state of True into False once the loop is exited when its conditions are met.
Counter-Controlled Loop
A counter-controlled loop repeats until its counters reach the preset value and break out of the loop. Here’s an example of how to end a while loop using the condition approach in a counter-controlled scenario:
count = 0
while count < 5:
print(count)
count += 1
In this instance, the loop continues while the variable ‘count’ is still less than 5. when the counter is 5, the loop terminates as count < 5 expression becomes False otherwise.
Flag-Based Approach
The flag-based way is one of the few prevalent applications of the condition approach. In this case, a flag variable is being used to be able to run the loop as long as the specified condition is met. Loop start to run while flag hold True and finally, it start executing when a certain condition is met and enter to False.Here’s an example:
loop_active = True
while loop_active:
# Perform tasks
if some_condition:
loop_active = False
In this example, ‘loop_active’ is True (active) all the time until the loop stops executing. But if some_condition is true suggesting that the specific condition to stop the loop has just happened, loop_active is set to False and terminates the loop.
Using the ‘break’ Statement to End a While Loop
The ‘break’ statement is a secret weapon for programmers that permits them to get out of a loop even before the exit condition is satisfied. This statement is very important in managing the execution flow of loops well especially in circumstances where an immediate termination is needed. Let’s focus on the particulars of ‘break’ statement application in ‘while’ loops.
Overview of the ‘break’ Statement
The ‘break’ statement in Python serves a fundamental purpose: to end the execution of a loop immediately. It can be positioned within a loop body and, when met, prevents the loop from running any more iterations and exiting the remaining ones. The ‘break’ feature will be very useful when particular circumstances occur that would make an immediate termination of the loop a mandatory prerequisite. Here’s a breakdown of scenarios where ‘break’ is useful:
- Emergency Exit: The ‘break’ statement is invaluable when exceptions arise suddenly such that a loop is forced to cease execution. This way, the program will not go on running unnecessary iterations after the desired result has already been found or further execution would be pointless;
- Complex Conditions: In instances where the end condition of a loop is complicated or depends on multiple situations, the ‘break’ statement provides a neat and suitable solution. Instead of trying to cover all the possibilities of the loop’s condition, ‘break’ offers a simple exit that is based on specific circumstances.
Example Implementation
Consider the following example demonstrating the usage of the ‘break’ statement within a while loop:
while True:
user_input = input("Enter 'exit' to end the loop: ")
if user_input == 'exit':
break
In this example:
- The while loop runs indefinitely (while True:) remains until the ‘break’ condition is identified;
- In each of the looping cycles (iteration), the user is provided with an opportunity to enter a value;
- If the user enters ‘exit’, the ‘break’ statement is utilized to directly exit the circumstance, not depending on its original condition.
Advantages of Using ‘break’
Aspect | Description |
---|---|
Flexibility | The break statement introduces a flexible approach to terminating loops, over which programmers have only limited control of circumstances that allows them to exit loops under different conditions. |
Efficiency | “break” increases code performance by directly halting the loop execution, this is a very essential situation when there is not necessarily no desirable prolonged iterations. |
Simplicity | Incorporating ‘break’ statements improves loop termination logic readability and facilitates code that is easier to maintain, primarily in advanced scenarios. |
Combining ‘continue’ and ‘break’ in a While Loop
The combination of the ‘continue’ and ‘break’ statements offers a powerful mechanism to control the flow of execution within a ‘while’ loop. Understanding how to effectively use these statements can enhance the flexibility and efficiency of your code.
- continue Statement: The ‘continue’ statement is used to skip the rest of the code within the loop for the current iteration and proceed directly to the next iteration. This means that any code following the ‘continue’ statement within the loop will be ignored, and the loop will immediately jump back to the beginning for the next iteration;
- break Statement: Conversely, the ‘break’ statement is used to completely exit the loop, regardless of whether the loop’s condition still evaluates to True. Upon encountering a ‘break’ statement, the loop is terminated, and the program execution moves to the first line of code after the loop.
Let’s examine a practical scenario where combining ‘continue’ and ‘break’ statements can be beneficial.
python
Copy code
while True:
data = get_data()
if data is None:
continue
if data == 'exit':
break
p
In this example, a ‘while’ loop is initiated with a condition that always evaluates to True, effectively creating an infinite loop until explicitly terminated. Within the loop, the ‘get_data()’ function is called to retrieve some data. If the returned data is ‘None’, the ‘continue’ statement is executed, skipping the rest of the loop’s code and moving to the next iteration.
If the data is not ‘None’, the loop checks whether it is equal to ‘exit’. If it is, the ‘break’ statement is triggered, exiting the loop entirely. Otherwise, the ‘process_data()’ function is called to handle the data.
By combining ‘continue’ and ‘break’ statements in this manner, the loop can efficiently handle various scenarios:
- Skipping Invalid Data: The ‘continue’ statement allows the loop to skip processing invalid or null data, ensuring that only valid data is processed;
- Exiting Loop on Condition: The ‘break’ statement enables the loop to be terminated based on a specific condition, such as when the desired task is completed or when a termination command like ‘exit’ is received.
Common Pitfalls and How to Avoid Them
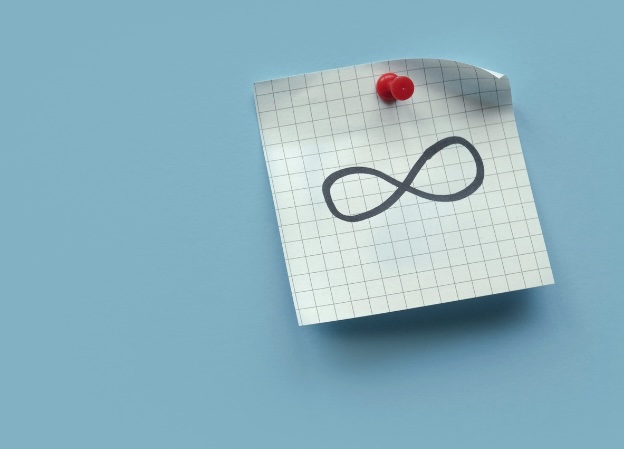
When mastering the art of ending a while loop in Python, it’s crucial to be mindful of common pitfalls that can lead to errors and inefficiencies. By understanding these pitfalls and implementing best practices, you can write more robust and maintainable code. Here are some key pitfalls to watch out for:
Infinite Loops
One of the most common pitfalls when working with while loops is inadvertently creating an infinite loop. An infinite loop occurs when the loop’s condition never evaluates to false, causing the loop to continue indefinitely. This can lead to your program becoming unresponsive or consuming excessive system resources.
To avoid infinite loops, always ensure that the loop’s condition will eventually become false. This typically involves updating loop control variables within the loop or using conditional statements to check for termination conditions. Here’s an example demonstrating how to prevent an infinite loop:
count = 0
while count < 10:
print(count)
count += 1
In this example, the loop will terminate once the count variable reaches 10, preventing it from running indefinitely.
Overuse of break
While the break statement can be a useful tool for prematurely exiting a loop, overusing it can lead to code that is difficult to understand and maintain. Using break excessively can also make it harder to debug logic errors, as it interrupts the natural flow of the loop.
To avoid overusing break, carefully consider whether it’s truly necessary to exit the loop at a given point. In many cases, refactoring your code to use clearer termination conditions or restructuring your loop logic can eliminate the need for a break altogether. Here’s an example illustrating when the break should be used sparingly:
while True:
user_input = input("Enter a number (type 'exit' to quit): ")
if user_input == 'exit':
break
else:
print("You entered:", user_input)
In this example, a break is used judiciously to exit the loop only when the user enters ‘exit’, preventing unnecessary interruptions to the loop’s execution.
Neglecting continue
While break allows you to exit a loop prematurely, the continue statement allows you to skip the rest of the loop’s current iteration and proceed to the next iteration. Neglecting to use continue when appropriate can result in redundant or inefficient code.
To leverage continue effectively, identify situations where certain iterations of the loop should be skipped based on specific conditions. By using continue, you can streamline the processing within your loop and improve code readability. Here’s an example demonstrating the use of continue:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for num in numbers:
if num % 2 == 0:
continue
print(num)
In this example, continue is employed to skip even numbers, allowing only odd numbers to be printed during each iteration of the loop.
Conclusion
Understanding how to end a while loop in Python is a fundamental skill for any aspiring Python programmer. Whether through manipulating the loop’s condition, using break or continue, or avoiding common pitfalls, mastering while loops will significantly enhance your coding proficiency in Python.
FAQ
Yes, you can have multiple break statements, but the loop will exit after the first break is executed.
Continue skips to the next iteration of the loop, while break completely exits the loop.
Technically, yes, through exceptions or by using a return statement in a function. However, these are less common practices.