Python, a programming language that is both popular and flexible, has multiple methods for manipulating the mathematical constant Pi (π). This article explores the several ways to import Pi into Python, write Pi in Python, and import Pi into Python. We’ll cover everything from the most fundamental to the most complex methods, so that programmers of all skill levels can learn Python and Pi.
What is Pi?
Pi is a mathematical constant that represents the relationship between the circumference (C) and diameter (d) of a circle. It is typically represented as π. Here is how this relationship is stated: Pi (π) ≈ 3.14159
The fact that pi defies exact representation as a finite decimal or simple fraction indicates that it is an irrational number. There is no repetition in its decimal representation; it continues indefinitely. Although 3.14159 is the generally used estimate, more precise numbers can be computed as needed.
Why Use Pi in Python?
Python is a multi-purpose computer language that has many applications in many fields, such as engineering, mathematics, and science. Pi plays a crucial part in mathematical calculations, which is why it is significant in Python programming. It includes:
Geometry
Geometry relies on pi for computing spherical and circle parameters. You can use Python to find the area and circumference of a circle by just plugging in Pi. As an illustration, the formula can be used to determine the circumference of a circle given its diameter (d):
C = π * d
In Python, this can be implemented as:
import math
diameter = 10
circumference = math.pi * diameter
Trigonometry
Pi is essential in trigonometry, especially when working with angles measured in radians. It is used to convert between degrees and radians, simplifying trigonometric calculations in Python. To find the sine of an angle in degrees, you can use the math.sin() function as follows:
import math
angle_in_degrees = 45
angle_in_radians = math.radians(angle_in_degrees)
sine_value = math.sin(angle_in_radians)
Scientific Computations
In scientific computing and simulations, Pi is frequently used in equations related to physics, engineering, and mathematics. It is indispensable for solving differential equations, modeling physical phenomena, and conducting numerical simulations. Python’s extensive library ecosystem, including NumPy and SciPy, provides tools for incorporating Pi into these calculations.
The Basics: Writing Pi in Python Without Importing
Direct Declaration
One of the simplest ways to represent Pi in Python is by directly declaring it in your code as a variable. This method involves assigning the approximate value of Pi to a variable, making it readily available for use in your calculations. Here’s how you can achieve this:
pi = 3.14159
Advantages:
- Simplicity: Direct declaration is straightforward and easy to implement;
- Readability: The code is clear and intuitive, as you explicitly define Pi.
Limitations:
- Lack of Precision: While 3.14159 is a commonly used approximation for Pi, it is not entirely precise. Pi is an irrational number with an infinite decimal expansion, so this approximation is an oversimplification. For applications requiring high precision, this method falls short.
Using String Formatting
To increase the precision of your Pi representation without importing external libraries, you can use string formatting. This approach allows you to specify the number of decimal places you want to display. Here’s how you can achieve this:
pi = “{:.5f}”.format(22/7)
In this code snippet:
- “{:.5f}” is a format string that instructs Python to display the floating-point number with five decimal places;
- 22/7 is a common fractional approximation of Pi.
Advantages:
- Improved Precision: String formatting lets you control the precision of Pi. By adjusting the number of decimal places in the format string, you can increase or decrease precision as needed.
Limitations:
- Rational Approximation: While using the fraction;
- 22/7 is a better approximation than 3.14159, it is still an approximation. Pi’s true value is an irrational number, so any rational approximation will have some level of inaccuracy.
How to Import Pi in Python Using the math Module
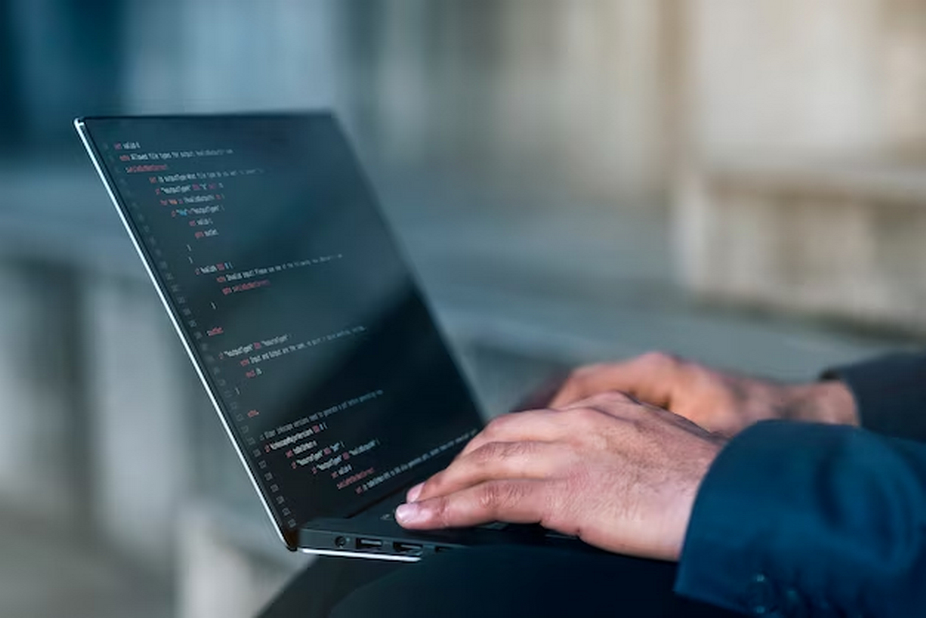
The math module is a fundamental part of Python’s standard library. It contains a plethora of mathematical functions and constants that can assist programmers in performing complex mathematical calculations. One of its key constants is Pi, denoted by the Greek letter π.
Importing Pi from the math Module
To access Pi (π) from the math module, follow these simple steps:
- Import the math Module: Begin by importing the math module into your Python script. This is done using the import statement as follows: import math;
- Assign Pi (π) to a Variable: After importing the math module, you can assign the value of Pi to a variable of your choice. Conventionally, it is named pi for clarity and consistency: pi = math.pi.
Now, you have successfully imported the mathematical constant Pi (π) into your Python script and assigned it to the variable pi.
Advantages of Using the math Module for Pi
Using the math module to access Pi (π) in Python offers several advantages:
- High-Precision Value of Pi: The math module provides a highly accurate value of Pi (π) to your Python program. This precision is particularly crucial for scientific and engineering applications where exact mathematical constants are required for accurate calculations;
- No Need to Manually Define Pi: By utilizing the math module, you eliminate the need to manually define the value of Pi in your code. The module handles the storage and retrieval of Pi, ensuring that you always have access to the correct, up-to-date value without the risk of inaccuracies introduced by manual declarations.
Practical Applications of Pi in Python
Pi (π) is a versatile mathematical constant, and using it with the math module opens up numerous possibilities for calculations. Here are some practical applications where Pi can be employed:
- Geometry: Pi is frequently used to calculate the circumference and area of circles, as well as the volume and surface area of spheres and cylinders;
- Trigonometry: Pi plays a pivotal role in trigonometric functions such as sine, cosine, and tangent, which are essential for various mathematical and scientific computations;
- Physics: In physics, Pi is used in equations related to waves, oscillations, and rotations. For example, it is used in calculating the period and frequency of oscillating systems;
- Engineering: Engineers rely on Pi when designing structures, calculating stress and strain in materials, and solving problems related to fluid dynamics.
Advanced Techniques: Other Ways to Import Pi into Python
Numpy is a widely-used Python library designed specifically for numerical computations. It provides efficient tools for working with arrays, matrices, and mathematical operations. When it comes to importing Pi using numpy, the precision and capabilities it offers can be advantageous in certain scenarios.
Importing Pi from numpy
To import Pi (π) from numpy, follow these steps:
- Import the numpy Module: Begin by importing the numpy module, typically using the conventional alias np for brevity: import numpy as np;
- Assign Pi (π) to a Variable: After importing numpy, you can assign the value of Pi to a variable, often named pi, like this: pi = np.pi.
Now, you have successfully imported Pi (π) into your Python script using the numpy library.
Using sympy for Symbolic Mathematics
Sympy is a Python library that specializes in symbolic mathematics. It allows you to work with mathematical symbols, equations, and expressions symbolically, making it particularly useful for algebraic and calculus-related tasks.
To import Pi (π) using sympy, follow these steps:
- Import Pi from sympy: In sympy, Pi is readily available as a symbol. To import it, use the following statement: from sympy import pi
With this import, you can now use Pi symbolically in your Python code.
Comparing Precision
Different methods of importing Pi into Python offer varying levels of precision. Here is a comparison of the precision levels for Pi obtained through these methods:
Method | Precision |
Direct | Limited |
math | High |
numpy | Very High |
sympy | Symbolic |
- Direct: This refers to manually specifying the value of Pi in your code, which has limited precision due to the finite number of digits you can provide;
- math: Using the math module provides a high level of precision for practical numerical calculations;
- numpy: The numpy module offers very high precision, making it suitable for scientific and engineering applications that demand extreme accuracy;
- sympy: sympy allows for symbolic representation of Pi, making it ideal for symbolic mathematics, algebraic manipulation, and exact mathematical expressions.
Practical Applications: Using Pi in Python Projects
Pi (π) is a fundamental mathematical constant with diverse applications across various fields such as science, engineering, and mathematics. Let’s explore practical applications of Pi in Python projects, providing examples and code snippets to illustrate its usage.
Calculating the Area of a Circle
Calculating the area of a circle is a classic application of Pi. Given the radius (r) of a circle, you can use the formula: Area = π * r^2
In Python, you can compute the area of a circle using Pi from the math module with the following code:
import math
radius = 5
area = math.pi * radius ** 2
Computing the Circumference of a Circle
Another common calculation involving Pi is finding the circumference of a circle. The formula for the circumference (C) of a circle is: Circumference = 2 * π * r
You can use Pi from the math module to compute the circumference in Python:
import math
radius = 5
circumference = 2 * math.pi * radius
Trigonometric Calculations in Scientific Projects
In scientific projects, Pi is essential for trigonometric calculations, including sine, cosine, and tangent functions. For instance, in projects related to oscillations, waveforms, or angular measurements, Pi is a fundamental constant.
Here’s an example of calculating the sine of an angle (in radians) using Pi:
import math
angle_radians = math.pi / 4 # Example angle in radians
sin_value = math.sin(angle_radians)
Area of a Circle
To calculate the area of a circle in Python, you can use the formula mentioned earlier:
import math
radius = 5
area = math.pi * radius ** 2
In this code snippet:
- We first import the math module to access Pi (π);
- Then, we define the radius of the circle as 5 (you can replace it with any desired value);
- Finally, we calculate the area using the formula π * r^2, where math.pi provides the value of Pi.
Conclusion
Understanding how to write Pi in Python, how to import Pi in Python, and how to import Pi into Python is a valuable skill for any programmer working with mathematical concepts. The flexibility of Python in handling constants like Pi makes it an ideal choice for a wide range of applications, from basic geometric calculations to complex scientific computations. With the knowledge of Pi in Python, the possibilities are as vast as the digits of Pi itself!
FAQ
Can I use Pi in Python without importing any modules?
Yes, you can define it manually, but for precision, it’s better to import it.
Which module provides the most accurate value of Pi?
numpy offers very high precision, but for most purposes, math.pi is sufficient.
Is it necessary to understand Pi for Python programming?
It’s not essential for all aspects of Python, but for mathematical and scientific computations, it’s very useful.
Can I use Pi in game development with Python?
Yes, especially in games involving physics or geometry.