Welcome to the wonderful world of Python, where the manipulation of numbers is as easy as pie! Today, we’re going to focus on a specific aspect that often puzzles beginners and pros alike: how to decrement in Python. Decrementing, the act of reducing a number, is a fundamental concept in programming, and mastering it can unlock new doors in your coding journey. So, let’s dive into the fascinating nuances of decrementing in Python and learn how to do it efficiently and effectively.
Understanding the Basics: What is Decrementing?
Before delving into the specifics of decrementing in Python, it’s crucial to understand the fundamental concept of decrementing itself. Decrementing refers to the process of reducing the value of a variable by a certain amount. In programming, this operation is often utilized to decrease the value of a variable by 1. However, it can also encompass subtracting any desired value from the variable. Below are the key points:
Definition
Decrementing involves reducing the value of a variable by a certain amount. In programming, this operation is often utilized to decrease the value of a variable by 1. However, it can also encompass subtracting any desired value from the variable. Key points to note about the definition of decrementing:
- Decrementing refers to the process of reducing the value of a variable;
- It can involve decreasing the value by 1 or subtracting any specified amount from the variable;
- Decrementing is a fundamental concept in programming and is widely used in various scenarios.
Purpose
The primary purpose of decrementing is to decrease the value of a variable by a specified amount. Understanding the purpose of decrementing is crucial for effectively managing variables in programming. Here are the main aspects regarding the purpose of decrementing:
- Decrementing is utilized to decrease the value of a variable by a specified amount;
- It helps in manipulating variable values according to program requirements;
- Decrementing is commonly employed in scenarios where iterative operations or countdowns are involved.
Common Usage
In many programming scenarios, decrementing by 1 is a common requirement. However, decrementing can also involve subtracting any desired value from the variable. Understanding the common usage of decrementing provides insight into its widespread application in programming. Key points about the common usage of decrementing include:
- Decrementing by 1 is prevalent in various programming tasks, such as iterating through lists or arrays;
- It is used in countdowns, loop control, and other iterative processes;
- Decrementing by a specific amount is employed in scenarios where variable values need to be adjusted by a predefined quantity.
Operator
Unlike some programming languages that provide a dedicated decrement operator, Python does not have one. However, there are alternative methods to achieve decrementing in Python. Understanding the absence of a dedicated decrement operator in Python is essential for effectively implementing decrementing operations. Key points regarding the operator aspect of decrementing include:
- Python does not have a dedicated decrement operator like other programming languages;
- Shorthand assignment operators, arithmetic subtraction, functions, and loops are commonly used to achieve decrementing in Python;
- Alternative methods provide flexibility and versatility in implementing decrementing operations in Python code.
Decrementing in Python
Python, renowned for its simplicity and readability, offers alternative methods to achieve decrementing without the presence of a dedicated decrement operator. Let’s explore some of the commonly used techniques:
Using Assignment Operators
In Python, decrementing can be accomplished using assignment operators in conjunction with subtraction. Here’s a basic example:
x = 10
x -= 1 # Decrementing x by 1
print(x) # Output: 9
Utilizing Built-in Functions
Python provides built-in functions like += and -= that can be employed for incrementing and decrementing respectively. Here’s how you can use the -= operator for decrementing:
y = 20
y -= 5 # Decrementing y by 5
print(y) # Output: 15
Employing Custom Functions
For more complex scenarios or when dealing with non-integer decrements, custom functions can be utilized. These functions can encapsulate the decrementing logic based on specific requirements.
def decrement_value(value, decrement_by):
return value - decrement_by
z = 30
z = decrement_value(z, 10) # Decrementing z by 10
print(z) # Output: 20
Comparison with Other Languages
While languages like C or Java offer a decrement operator (–), Python’s approach of utilizing assignment operators aligns with its philosophy of readability and simplicity. Despite the absence of a dedicated decrement operator, Python’s flexibility allows for efficient decrementing through alternative means.
The Pythonic Way: How to Decrement in Python
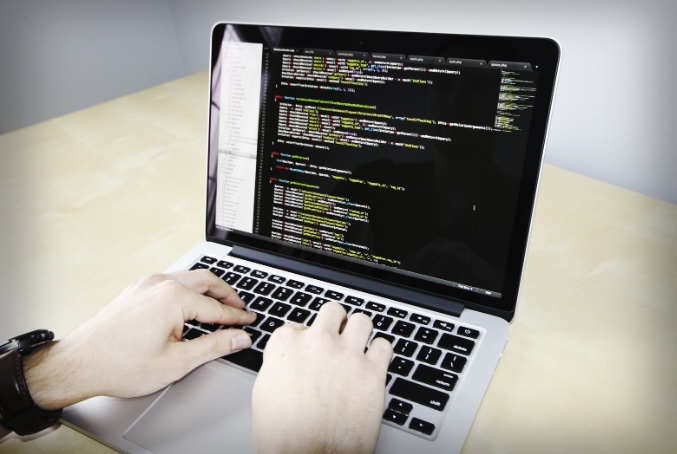
Python, renowned for its readability and simplicity, offers various approaches for decrementing values. Understanding these methods is crucial for efficient programming in Python. In this guide, we will explore two primary methods: using the subtraction operator (-) and the shortcut method.
Using the Subtraction Operator (-)
The subtraction operator (-) is a fundamental arithmetic operator in Python. It is employed to subtract one operand from another. When it comes to decrementing a variable, the subtraction operator can be directly applied as follows:
count = 10
count = count - 1
In the above code snippet, we initialize a variable count with the value 10. Then, we utilize the subtraction operator to decrease the value of count by 1, effectively decrementing it.
The Shortcut Method
Python facilitates a more succinct way to decrement a value through the use of the shortcut method. This method is commonly employed by experienced Python programmers to streamline their code. Instead of explicitly stating count = count – 1, Python provides a shorthand notation:
count -= 1
The above line is equivalent to count = count – 1. It reduces redundancy and enhances code readability, especially in scenarios where decrementing operations are frequently performed.
Comparison
Let’s compare the two methods side by side:
Method | Description |
---|---|
Subtraction Operator | Utilizes the subtraction operator (-) to decrement the value. |
Shortcut Method | Provides a concise notation (count -= 1) to decrement. |
Looping with Decrementing
Decrementing plays a crucial role, particularly within loops. Whether employing while or for loops, decrementing can effectively control the number of iterations, allowing for precise and efficient execution of code blocks.
Using while Loops
while loops are ideal when the number of iterations is not predetermined and depends on a condition. Decrementing within a while loop is straightforward:
python
Copy code
counter = 5
while counter > 0:
print(count
In the above code snippet, we initialize a variable counter with the value 5. The while loop continues iterating as long as the counter is greater than 0. With each iteration, the value of counter is decremented by 1 using the counter -= 1 statement.
Using for Loops
for loops in Python are primarily utilized for iterating over sequences, but they can also be adapted for decrementing purposes:
for i in range(10, 0, -1):
print(i)
In this example, the range() function generates a sequence of numbers from 10 down to 1, with a decrement of 1. The for loop then iterates over this sequence, assigning each value to the variable i and printing it.
Comparison of Looping Methods
Let’s compare the usage of decrementing within while and for loops:
Loop Type | Description |
---|---|
while Loop | Suitable when the number of iterations is not known beforehand and depends on a condition. |
for Loop | Ideal for iterating over sequences or when the range of iterations is predefined, making decrementing predictable. |
Decrementing in Real-world Scenarios
Understanding how to decrement in Python is not just a theoretical concept; it’s a practical skill with numerous applications in real-world scenarios. Let’s explore a couple of examples where decrementing is commonly employed to achieve specific functionalities:
Countdown Timer
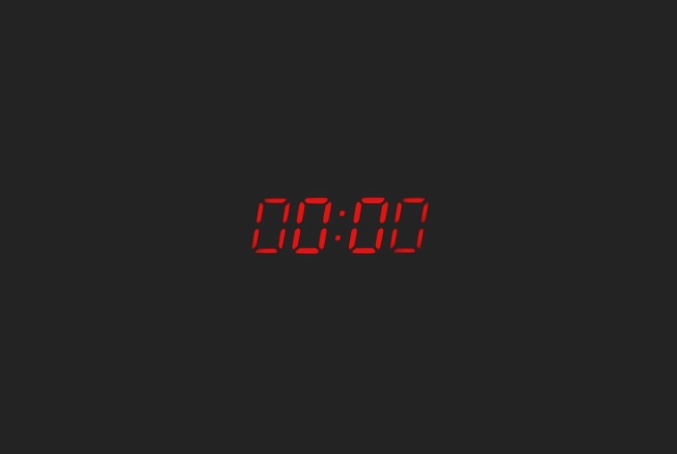
In applications like countdown timers, decrementing is essential for tracking and displaying the remaining time. Consider a scenario where you need to implement a simple countdown timer in a Python application. Decrementing allows you to reduce the remaining time by a specified interval, typically seconds, until it reaches zero. Here’s a basic example:
import time
def countdown_timer(seconds):
while seconds > 0:
print(f"Time remaining: {seconds} seconds")
time.sleep(1) # Wait for 1 second
seconds -= 1 # Decrement the remaining seconds
print("Time's up!")
countdown_timer(10) # Start a countdown timer for 10 seconds
In this example, the countdown_timer function takes the number of seconds as input. It then enters a while loop, continuously printing the remaining time and decrementing the seconds variable until it reaches zero. This functionality is fundamental for creating countdown timers in various applications, such as cooking timers, exam timers, or any situation where time tracking is required.
Games
In game development, decrementing is often utilized for various purposes, such as reducing a player’s health or depleting resources over time. Let’s consider a simple game scenario where the player’s health decreases gradually over time due to environmental hazards:
def player_health():
health = 100
while health > 0:
print(f"Player's health: {health}%")
# Simulate environmental damage
health -= 10 # Decrement player's health
time.sleep(1) # Wait for 1 second
print("Game over - Player is out of health!")
player_health() # Simulate player's health decreasing over time
In this example, the player_health function initializes the player’s health to 100 and enters a while loop. Within the loop, the player’s health is gradually decremented by 10 percent each iteration to simulate damage from environmental hazards. This mechanism adds realism and challenge to the game, enhancing the overall gaming experience.
Conclusion
Understanding how to decrement in Python is a small yet significant step in your Python programming journey. It’s a fundamental concept that, once mastered, can greatly enhance the functionality and efficiency of your code. Keep experimenting with decrementing in different scenarios, and you’ll soon find it an indispensable tool in your Python toolkit.
Remember, the key to mastering how to decrement in Python, as with any programming skill, lies in practice and exploration.
FAQ
Yes! You can decrement by any value by simply changing the number you subtract. For instance, count -= 2 will decrement count by 2.
No, Python does not have a — operator like C or Java. You need to use the -= operator or count = count – 1.
Absolutely! Decrementing works with floating-point numbers just as it does with integers. For example, number -= 0.5.
To decrement each element in a list, you can use a loop:
numbers = [10, 20, 30]
for i in range(len(numbers)):
numbers[i] -= 1
Yes, by using the range() function with a negative step: for i in range(start, end, -step).