In the world of python programming, string handling gets a high priority in many areas. Within the treasure trove of facilities Python possesses, the . strip () method is a strong tool that is seldom acknowledged yet stands out. In this article, we will explore not only “what does .strip do in Python”, but also the intricacies of how it operates, its uses, and the typical questions that arise when we are dealing with it. Mainly, .strip function strip away leading and trailing characters typically whitespace from a string. This would be helpful to do during data cleaning and preparation, where unnecessary spaces can disorganize it and interrupt processing. Furthermore the character masking can be configured to strip specific ones, hence the versatility. However, we further demonstrate its effectiveness with practical cases, which correspond to real-world situations.
Understanding the Basics of .strip() in Python
The strip() is a very useful in-built function within the Python string class, which is specially designed for string stripping of leading and trailing characters. Having good control on this technique is imperative for string operations in Python. Let’s take a closer look at its efficiency and operation.
Structure of .strip()
The .strip() method follows a simple structure:
str.strip([chars])
Here’s a breakdown of its components:
str Parameter
str Parameter is a key participant in the operation of the . strip() methods in Python. It represents the removal of the leading and the tailing characters of a supplied string. Its multifaceted role encompasses several nuances:
- Indispensable Requirement: The stripping of unnecessary characters, emphasized by the parameter str, is mandatory. Devoid of a kernel stringier than it is, the phrase can be restrained to its transformative potentials;
- String Sanctity: The whole input string is shielded against Structural changes via usage of the declare str parameter and remains intact after the stripping process.
Going further, beside the str key, the string is much more than the beginning and the end. It is also the essence of Python. Its invariable existence is the translation of the need of a realistic entity to serve you well while using the .strip() method.
[chars] Parameter
The [chars] parameter, while optional in nature, bestows upon the .strip() method a heightened degree of versatility, enabling users to tailor the stripping process according to specific character criteria. Its nuanced functionality imbues the method with a layer of customization:
- Tailored Stripping: By virtue of the [chars] parameter, users wield the power to delineate a curated set of characters slated for removal from both extremities of the string. This bespoke stripping mechanism affords users unparalleled control over the transformational dynamics of the method;
- Default Behavior: In the absence of explicitly specified characters within the [chars] parameter, the .strip() method defaults to its intrinsic functionality of removing whitespace characters. This intrinsic behavior ensures seamless operation in scenarios where explicit character criteria are not delineated.
The [chars] parameter serves as a veritable canvas upon which users paint the contours of their stripping aspirations. Its optional nature underscores the method’s adaptability, accommodating a spectrum of stripping exigencies with finesse and aplomb.
.strip() effectively sanitizes the string by removing unwanted leading and trailing characters. These characters could be whitespace, newline characters, tabs, or any specified characters provided within the optional chars parameter. Let’s illustrate the usage with some examples:
Example 1: Basic Usage
sentence = " Hello, World! "
stripped_sentence = sentence.strip()
print(stripped_sentence) # Output: "Hello, World!"
In this example, .strip() removes leading and trailing whitespace characters, resulting in the cleaned string “Hello, World!”.
Example 2: Removing Specific Characters
python
Copy code
text = "===Python==="
cleaned_text = text.strip("=")
print(cleaned_text) #
Here, .strip(“=”) removes leading and trailing occurrences of the character ‘=’ from the string, leaving behind the cleaned string “Python”.
Example 3: Using .strip() with Custom Characters
message = "*****Important*****"
cleaned_message = message.strip("*")
print(cleaned_message) # Output: "Important"
In this case, .strip("*") removes the asterisk (*) characters from both ends of the string, resulting in the cleaned string "Important".
How .strip() Works
The .strip() method in Python is a useful tool for string manipulation. Its primary function is to remove whitespace characters from the beginning and end of a string. However, it also offers the flexibility to remove specific characters from the string’s edges.
Default Behavior
By default, the .strip() method removes whitespace characters, such as spaces, tabs, and newline characters, from the start and end of a string. This default behavior is particularly handy when dealing with user input or when parsing text data.
text = " Hello, World! "
stripped_text = text.strip()
print(stripped_text) # Output: "Hello, World!"
In this example, the leading and trailing spaces are removed from the string, leaving only the content in between intact.
Custom Characters
In addition to whitespace, the .strip() method allows you to specify custom characters that you want to remove from the beginning and end of the string. You can do this by passing a string containing the characters you wish to remove as an argument to the method.
python
Copy code
text = "***Hello, World!***"
custom_stripped_text = text.strip('*')
print(custom_stripped_text) # Output
Here, the asterisks (*) surrounding the string are removed because they were specified as the characters to strip.
Comparison with .lstrip() and .rstrip()
Python also provides .lstrip() and .rstrip() methods to strip characters exclusively from the left and right sides of a string, respectively. While .lstrip() removes characters only from the beginning of the string, and .rstrip() removes characters only from the end, .strip() removes characters from both ends simultaneously.
text = " Hello, World! "
left_stripped_text = text.lstrip()
right_stripped_text = text.rstrip()
print(left_stripped_text) # Output: "Hello, World! "
print(right_stripped_text) # Output: " Hello, World!"
In the example above, you can observe the differences between .strip(), .lstrip(), and .rstrip(). While .strip() removes both leading and trailing whitespace, .lstrip() and .rstrip() remove only leading and trailing whitespace, respectively.
Practical Uses of .strip() in Python
The .strip() method in Python is not only a fundamental tool for string manipulation but also finds extensive practical applications across various domains. Let’s explore some of the most common uses of .strip() in real-world scenarios:
Cleaning Data
In data processing tasks, cleanliness and consistency are paramount. Often, data obtained from external sources may contain leading or trailing whitespace characters that need to be removed for accurate analysis. Here, the .strip() method proves invaluable for eliminating unwanted padding or spaces from strings, ensuring data integrity.
data = " John Doe "
cleaned_data = data.strip()
print(cleaned_data) # Output: "John Doe"
By applying .strip() to each data entry, you can standardize the format and facilitate seamless data processing.
Form Validation
In web development, user inputs via forms are susceptible to accidental leading or trailing spaces, which can disrupt data validation processes. Employing .strip() during form validation routines helps mitigate this issue by ensuring that extraneous spaces do not interfere with the validation logic.
user_input = " [email protected] "
stripped_input = user_input.strip()
# Validate email address
if stripped_input == user_input:
# Proceed with validation logic
pass
else:
# Handle invalid input
pass
Here, .strip() aids in maintaining the integrity of user-provided data, enhancing the robustness of web applications.
File Parsing
When reading text files in Python, lines often contain trailing newline characters (‘\n’) that may need to be removed for further processing. The .strip() method offers a convenient solution for cleaning up lines read from text files, ensuring consistency in data handling.
with open('data.txt', 'r') as file:
for line in file:
cleaned_line = line.strip()
# Process cleaned line
By incorporating .strip() into file parsing routines, you can streamline text processing operations and improve code readability.
Example Scenarios
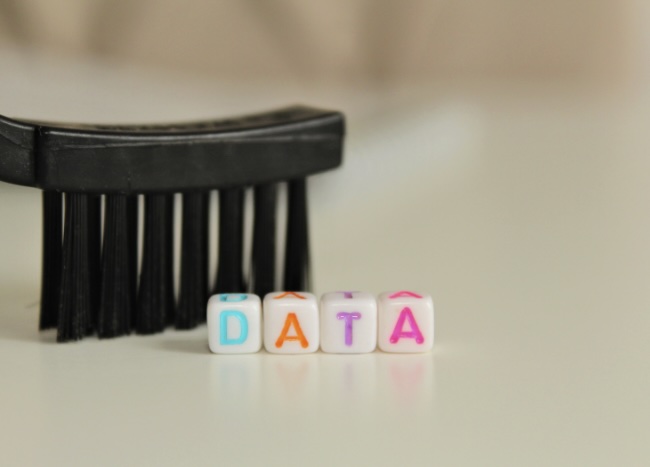
Let’s delve deeper into practical examples that illustrate the usage of the .strip() method in Python across different scenarios:
Data Cleaning
Consider a situation where you have textual data with unnecessary whitespace characters at the beginning and end. To ensure data cleanliness and consistency, you can utilize .strip() to remove these extraneous spaces.
data = ' Hello World '
cleaned_data = data.strip()
print(cleaned_data) # Output: 'Hello World'
In this example, .strip() removes the leading and trailing spaces from the string data, resulting in ‘Hello World’ with no padding.
Removing Specific Characters
Sometimes, you may need to remove specific characters from the edges of a string. For instance, suppose you have a string enclosed within certain characters that you want to eliminate. .strip() can be used with custom characters to achieve this.
data = 'xxHello Worldxx'
cleaned_data = data.strip('x')
print(cleaned_data) # Output: 'Hello World'
Here, the characters ‘x’ surrounding the string are specified as the characters to strip. As a result, .strip(‘x’) removes these characters from both ends, leaving ‘Hello World’ as the cleaned string.
Comparison with .lstrip() and .rstrip()
While .strip() removes characters from both ends simultaneously, Python provides .lstrip() and .rstrip() methods for exclusive removal from the left and right sides, respectively.
data = 'xxHello Worldxx'
left_stripped_data = data.lstrip('x')
right_stripped_data = data.rstrip('x')
print(left_stripped_data) # Output: 'Hello Worldxx'
print(right_stripped_data) # Output: 'xxHello World'
In the example above, .lstrip(‘x’) removes ‘x’ characters only from the left side, while .rstrip(‘x’) removes them from the right side, leaving the content in between unchanged.
Differences Between .strip(), .lstrip(), and .rstrip()
When understanding “what does .strip do in Python,” it’s crucial to differentiate between .strip(), .lstrip(), and .rstrip(). While these methods share the common goal of removing characters from strings, they operate differently based on the placement of the characters to be stripped.
.strip()
The .strip() method removes characters from both ends of a string. It scans the string from the beginning and end simultaneously until it encounters characters that are not in the stripping set. Once such characters are found, it stops and removes all preceding and succeeding characters within the stripping set.
text = "***Hello, World!***"
stripped_text = text.strip('*')
print(stripped_text) # Output: "Hello, World!"
In this example, .strip(‘*’) removes asterisks (*) from both ends of the string, leaving ‘Hello, World!’ as the cleaned text.
.lstrip()
The .lstrip() method removes characters from the left end (start) of a string. It scans the string from the beginning until it encounters a character that is not in the stripping set. It then removes all preceding characters within the stripping set, leaving the rest of the string unchanged.
text = "***Hello, World!***"
left_stripped_text = text.lstrip('*')
print(left_stripped_text) # Output: "Hello, World!***"
Here, .lstrip(‘*’) removes asterisks () from the left end of the string, leaving ‘Hello, World!**’ as the resulting text.
.rstrip()
The .rstrip() method removes characters from the right end (end) of a string. It scans the string from the end until it encounters a character that is not in the stripping set. It then removes all succeeding characters within the stripping set, leaving the rest of the string unchanged.
text = "***Hello, World!***"
right_stripped_text = text.rstrip('*')
print(right_stripped_text) # Output: "***Hello, World!"
Similarly, .rstrip(‘*’) removes asterisks (*) from the right end of the string, resulting in ‘***Hello, World!’ as the output.
Comparison
To summarize, the key differences between .strip(), .lstrip(), and .rstrip() are as follows:
- .strip(): Removes characters from both ends of the string;
- .lstrip(): Removes characters from the left end (start) of the string;
- .rstrip(): Removes characters from the right end (end) of the string.
Handling Unicode Characters with .strip()
Handling Unicode characters with .strip() in Python is a crucial aspect of string manipulation, particularly in scenarios where text data contains a variety of characters from different languages and character sets. This discussion aims to delve deeper into the intricacies of how .strip() interacts with Unicode characters, providing comprehensive explanations, examples, and practical insights.
Understanding .strip()
Before delving into its Unicode handling capabilities, it’s essential to grasp the fundamental functionality of the .strip() method. In Python, .strip() is a built-in method used to remove leading and trailing characters from a string. Its syntax is straightforward:
string.strip([chars])
Where string represents the input string and chars is an optional parameter indicating the characters to be removed. If chars are not specified, .strip() defaults to removing whitespace characters.
Handling Unicode Characters
Unicode characters are essential for representing a wide range of characters from different writing systems worldwide. Python’s support for Unicode enables developers to work seamlessly with text data across diverse linguistic contexts.
.strip() is adept at handling Unicode characters, making it a versatile tool for string manipulation. For instance, consider the following example:
text = u'\u202FSome text\u202F'.strip()
print(text) # Outputs: 'Some text'
In this example, the string u’\u202FSome text\u202F’ contains a non-breaking space Unicode character \u202F. Despite being a non-whitespace character, .strip() successfully removes it from both ends of the string, resulting in ‘Some text’.
Example: Removing Specific Unicode Characters
To further illustrate .strip()’s handling of Unicode characters, let’s explore removing specific Unicode characters from a string. Suppose we have a string with multiple occurrences of non-breaking space characters, and we want to eliminate them:
python
Copy code
text = u'\u202FSome\u202Ftext\u202F'.strip(u'\u202F')
print(text) # Out
In this example, .strip(u’\u202F’) effectively removes all instances of the non-breaking space Unicode character \u202F, resulting in the string ‘Some text’.
Key Points to Remember
- .strip() removes leading and trailing characters from a string;
- It seamlessly handles Unicode characters, including non-breaking spaces;
- Unicode characters can be specified as the parameter to remove specific characters using .strip().
Advanced Uses of .strip() in Python
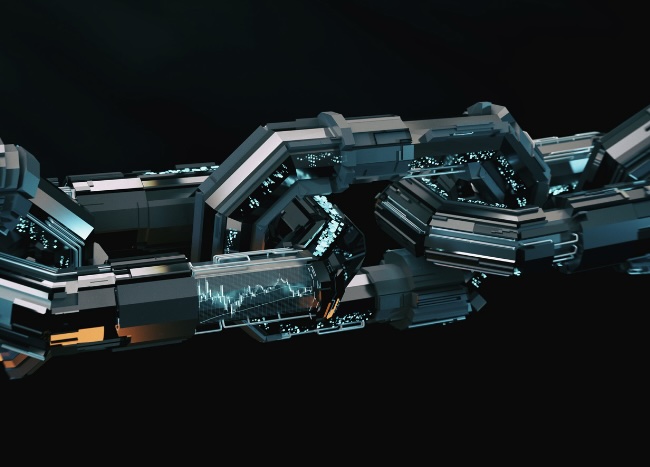
Advanced Uses of .strip() in Python offer developers powerful capabilities for string manipulation, including chain stripping and integration with regular expressions. These advanced techniques enable efficient handling of various text processing tasks with precision and flexibility.
Chain Stripping
One advanced use of .strip() involves chaining it with other string methods, creating concise yet powerful one-liners for string manipulation. This technique proves especially useful for removing specific characters from both ends of a string. Consider the following example:
result = ' Hello World!!! '.strip().rstrip('!')
print(result) # Outputs: 'Hello World'
In this example, .strip() is first applied to remove leading and trailing whitespace characters. Subsequently, .rstrip(‘!’) is chained to remove trailing exclamation marks, resulting in the string ‘Hello World’. This chaining of methods streamlines the code and enhances readability.
Regular Expressions Integration
For more complex patterns and intricate string manipulations, .strip() can be complemented with Python’s re module, enabling the use of regular expressions. Regular expressions offer powerful pattern matching capabilities, allowing developers to handle a wide range of string manipulation tasks with precision. Consider an example where we want to remove all digits from the beginning and end of a string:
import re
text = '123Hello456World789'
result = re.sub('^[\d]+|[\d]+$', '', text)
print(result) # Outputs: 'Hello456World'
In this example, re.sub() is used to substitute matches of the regular expression pattern ^[\d]+|[\d]+$ with an empty string. This pattern matches one or more digits ([\d]+) at the beginning (^) or end ($) of the string. By integrating .strip() with regular expressions, developers gain enhanced flexibility in handling complex string manipulation tasks.
Key Advantages
- Concise and Readable Code: Chaining .strip() with other string methods enables the creation of succinct and easily understandable code for string manipulation;
- Flexibility and Precision: Integration with regular expressions enhances the flexibility and precision of .strip(), allowing developers to handle complex string patterns and manipulations with ease.
Conclusion
Understanding “what does .strip do in Python” is more than a matter of syntactical knowledge. It’s about recognizing the efficiency and potential of Python in handling and manipulating string data. Whether you’re cleaning up user inputs, parsing files, or processing text data, .strip() offers a simple yet effective solution. Its versatility and ease of use make it an essential part of any Python programmer’s toolkit.
In summary, when we talk about “what does .strip do in Python,” we refer to a method that is small in syntax but huge in impact. It underscores Python’s commitment to providing robust tools for effective and efficient programming.
FAQ
The .strip() method in Python serves the purpose of manipulating strings by removing characters from both the beginning and end. It is particularly useful for sanitizing strings by eliminating unwanted leading and trailing characters.
While .strip() removes characters from both ends of a string simultaneously, .lstrip() exclusively removes characters from the left end (start), and .rstrip() exclusively removes characters from the right end (end) of the string. This distinction allows for more precise control over string manipulation.
By default, .strip() removes whitespace characters, including spaces, tabs, and newline characters, from the beginning and end of a string. This default behavior simplifies the process of cleaning up strings, especially when dealing with user input or text data.
Yes, .strip() allows users to specify custom characters to be removed from the string’s edges by passing them as an argument. This feature enables tailored stripping, catering to specific character removal requirements based on the application’s needs.
.strip() finds extensive use in various domains, including data cleaning, form validation, and file parsing. It ensures data integrity by removing unwanted padding or spaces, enhances web application robustness by validating user inputs, and streamlines text processing operations during file parsing.