The Python programming language, which is known for its flexibility and power, includes a math module with different operator functions to perform mathematical operations. Herein belongs the operator that brings about exponential function. Specifically, this article delves into the query: Where in addition, which of the mathematical operators would be used to raise 5 to the second power in Python? Squaring a number is basically a process of obtaining the square of that number, and it is important in very many math and science computations. This is the main scope of our research, concerning the mechanisms of this approach to learning in Python.
Understanding Python Exponentiation
At the core of mathematical operations in Python lies the exponentiation operator, denoted as ‘**’. This operator serves as a fundamental tool for raising a number to a certain power. For instance, if one wonders how to raise 5 to the second power in Python, the answer lies in utilizing the exponentiation operator: 5 ** 2. This expression effectively multiplies the number (in this case, 5) by itself the specified number of times (here, 2 times).
Exponentiation is a concept deeply ingrained in mathematical computations and finds extensive use in various fields such as scientific computing, engineering, and finance. In Python, the exponentiation operator provides a concise and intuitive means to perform such computations, offering developers a powerful tool for handling complex mathematical tasks with ease.
Let’s delve deeper into how the exponentiation operator works in Python:
Syntax
The syntax of the exponentiation operator in Python is straightforward. It consists of two operands, separated by the ‘**’ symbol. The first operand represents the base, while the second operand denotes the exponent.
- Example: Consider the expression 5 ** 2. Here, 5 is the base, and 2 is the exponent. When evaluated, this expression yields the result 25, as 5 raised to the power of 2 equals 25;
- Usage: The exponentiation operator is versatile and can be used with both integer and floating-point operands. Additionally, it can handle negative exponents and expressions involving variables and constants;
- Powerful Computations: Beyond simple exponentiation, the exponentiation operator enables developers to perform advanced computations efficiently. For instance, it facilitates calculations involving compound interest, exponential growth, and mathematical modeling.
Example
Python’s exponentiation operator offers flexibility and efficiency in mathematical computations. It empowers developers to handle various scenarios seamlessly.
- Ease of Use: With a clear syntax, the exponentiation operator simplifies expressing mathematical operations, enhancing code readability;
- Compatibility: Whether dealing with integers, floats, negative exponents, or complex expressions, the operator accommodates diverse use cases;
- Performance: Leveraging optimized algorithms, Python’s exponentiation operator ensures efficient computation even for large-scale problems.
Usage
The exponentiation operator in Python supports dynamic computations, making it an indispensable tool in scientific and engineering applications.
- Precision: Python’s exponentiation operator maintains precision even for high-order exponents, ensuring accurate results;
- Versatility: From simple arithmetic to complex mathematical modeling, the operator seamlessly integrates into various computational tasks;
- Expressiveness: With concise syntax, the exponentiation operator enhances code expressiveness, enabling developers to convey mathematical concepts effectively.
Powerful Computations
By leveraging the exponentiation operator, developers can streamline mathematical operations, leading to more efficient and maintainable codebases.
- Code Simplicity: Instead of using lengthy mathematical expressions, developers can utilize the exponentiation operator for concise and readable code;
- Algorithmic Efficiency: The exponentiation operator enables developers to optimize algorithms, enhancing computational performance;
- Problem Solving: From financial modeling to scientific simulations, the exponentiation operator serves as a fundamental tool in solving diverse problems across domains.
By understanding the intricacies of the exponentiation operator in Python, developers gain the ability to leverage its capabilities effectively. Whether working on scientific simulations, financial models, or algorithmic tasks, mastering the exponentiation operator empowers developers to tackle complex problems with confidence.
Syntax and Usage
Exponentiation in Python is denoted using the syntax number ** exponent. This notation closely aligns with the mathematical notation of exponentiation, making it intuitive for users familiar with mathematical concepts. Let’s delve into the syntax and usage of exponentiation in Python, along with some examples to illustrate its functionality.
Syntax
The syntax for exponentiation in Python is simple and straightforward:
number ** exponent |
Here, number is the base value, and exponent is the power to which the base value is raised. The result of the operation is the value of the number raised to the power of the exponent.
Example
Let’s consider an example to illustrate the syntax and usage of exponentiation in Python:
result = 5 ** 2 print(result) # Output: 25 |
In this example, 5 is raised to the power of 2, resulting in 25.
Usage
Exponentiation is commonly used in various computational tasks, such as mathematical calculations, scientific simulations, and data analysis. Here are some key use cases and scenarios where exponentiation plays a crucial role:
- Mathematical Calculations: Exponentiation is fundamental in mathematical calculations involving powers and exponential functions. It is used in mathematical models, equations, and formulas across different domains, including physics, engineering, and finance;
- Scientific Computing: In scientific computing, exponentiation is frequently employed in simulations, modeling physical phenomena, and solving differential equations. It enables researchers and scientists to analyze complex systems and predict outcomes accurately;
- Data Analysis: Exponentiation is utilized in data analysis and statistical computations, particularly in exponential growth models, exponential smoothing techniques, and exponential distribution functions. It helps in analyzing trends, forecasting future values, and understanding the behavior of data over time;
- Algorithm Design: Exponentiation plays a vital role in algorithm design and optimization, especially in algorithms related to cryptography, number theory, and computational geometry. Efficient algorithms for exponentiation, such as exponentiation by squaring, are crucial for enhancing the performance of cryptographic protocols and computational algorithms.
Advantages
The notation number ** exponent offers several advantages in Python programming:
- Intuitive Syntax: The syntax closely resembles mathematical notation, making it intuitive and easy to understand for users familiar with mathematical concepts;
- Flexibility: Exponentiation can be applied to various data types in Python, including integers, floating-point numbers, and even complex numbers. This flexibility allows for versatile usage in different contexts;
- Efficiency: Python’s built-in exponentiation operator is optimized for performance, ensuring efficient computation of power operations even for large numbers and exponents.
Applications of Exponentiation in Python
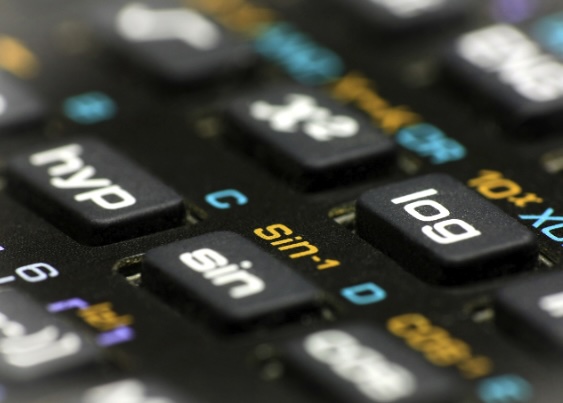
Exponentiation, a fundamental mathematical operation, is extensively utilized in Python programming across various domains. Understanding the operator for raising a number to a power, such as 5 to the second power, is merely the tip of the iceberg. Let’s delve into the diverse applications of exponentiation in Python:
Scientific Calculations
Exponentiation plays a pivotal role in scientific computations, especially when dealing with powers of numbers. Python’s built-in exponentiation operator ** simplifies such calculations.
# Calculating the square of a number
result = 5 ** 2 # Result: 25
Scientific research, engineering, and physics heavily rely on exponentiation for tasks like determining areas, volumes, and forces.
Financial Modeling
In finance, exponentiation is integral for modeling compound interest and predicting financial growth. Compound interest formulas involve raising a base to the power of time multiplied by the interest rate.
# Calculating compound interest
principal = 1000
rate = 0.05
time = 5
compound_interest = principal * (1 + rate) ** time
Financial analysts and economists utilize Python for developing models to forecast investment returns, evaluate risk, and optimize portfolios.
Data Science
Exponentiation finds extensive use in data science, particularly in algorithms and data transformations. It is crucial for statistical computations, such as scaling, normalization, and transformation of data distributions.
import numpy as np
# Generating exponential data
data = np.random.exponential(scale=2, size=1000)
Data scientists leverage exponentiation in machine learning algorithms, feature engineering, and simulation studies to analyze and interpret data effectively.
Common Mistakes and Misconceptions
When delving into Python’s mathematical operations, particularly exponentiation, it’s crucial to understand and avoid common mistakes and misconceptions. Here are some pitfalls to watch out for:
Confusing ” with ‘^’
One prevalent mistake is confusing the ” operator with ‘^’. In many programming languages, including some variations of Python, ‘^’ is used for exponentiation. However, in Python specifically, ‘^’ is a bitwise XOR operator, not an exponentiation operator.
# Incorrect usage of '^' for exponentiation
result = 5 ^ 2 # Result: 7 (Bitwise XOR), not 25 (Exponentiation)
To raise a number to a power in Python, you should use ‘**’ instead of ‘^’.
Misplacing the operator
Another common error occurs when misplacing the exponentiation operator. Placing the operator after the base number, rather than before the exponent, yields incorrect results.
# Incorrect placement of '**' operator
result = 2**5 # Result: 32, not 25 (Exponentiation should be 5**2)
To correctly raise a number to a power, ensure that the base number comes before the ‘**’ operator, followed by the exponent.
Understanding these distinctions is essential for accurately performing exponentiation operations in Python. Let’s summarize these points in a table for clarity:
Mistake | Explanation | Example |
---|---|---|
Confusing ‘**’ with ‘^’ | ‘^’ is a bitwise XOR operator in Python, not an exponentiation operator. Use ‘**’ for exponentiation. | result = 5 ^ 2 |
Misplacing the operator | Placing the exponentiation operator after the base number leads to incorrect results. Ensure the correct order: base_number ** exponent. | result = 2**5 |
By avoiding these common mistakes, you can ensure accurate exponentiation calculations in your Python programs, enhancing their reliability and correctness.
Python’s Math Module and Advanced Exponentiation
Python’s Math module is a powerful tool for performing various mathematical operations with precision and ease. Among its capabilities, the module extends the functionality of exponentiation, offering additional features beyond the built-in operators. Let’s delve into these advanced exponentiation techniques provided by Python’s Math module:
Using math.pow(x, y) for Floating-Point Exponentiation
The math.pow(x, y) function in Python’s Math module is utilized for floating-point exponentiation, where x is the base and y is the exponent. This function returns x raised to the power of y. Unlike the ** operator, which is limited to integer exponents, math.pow() accommodates both integer and floating-point exponents, thus offering enhanced flexibility. Consider the following example:
import math
result = math.pow(2, 3.5) # Evaluates 2^3.5
print(result) # Output: 11.313708498984761
In this example, math.pow(2, 3.5) calculates 2 raised to the power of 3.5, yielding a floating-point result of approximately 11.3137.
Utilizing math.sqrt(x) for Square Roots
Another valuable function provided by Python’s Math module is math.sqrt(x), which is specifically designed for computing square roots. Square roots are the inverse operation of squaring, finding the number that, when multiplied by itself, equals the given input x.
import math
result = math.sqrt(16) # Computes the square root of 16
print(result) # Output: 4.0
In this example, math.sqrt(16) calculates the square root of 16, resulting in 4.0.
Benefits of Python’s Math Module for Advanced Exponentiation
Feature | Description |
---|---|
Precision | Python’s Math module offers high precision in mathematical computations, particularly crucial for scientific and engineering applications. |
Flexibility | With functions like math.pow() accommodating both integer and floating-point exponents, users have greater flexibility in performing exponentiation operations. |
Clarity | Using dedicated functions like math.sqrt() enhances code clarity and readability, making it easier to understand the intention behind mathematical operations. |
Exponentiation and Its Role in Python Programming
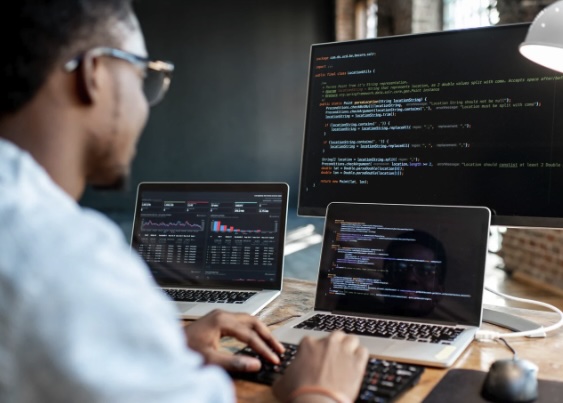
Understanding exponentiation, particularly in the context of Python programming, is fundamental for programmers as it forms the basis for numerous mathematical operations and algorithms. In Python, exponentiation refers to raising a base number to a certain power. For example, raising 5 to the second power can be achieved using the exponentiation operator.
result = 5 ** 2 # Computes 5 raised to the power of 2
print(result) # Output: 25
This operation results in 25, indicating that 5 has been raised to the power of 2.
Importance of Exponentiation in Python Programming
Exponentiation serves as a crucial building block for various computational tasks and algorithmic implementations. Its significance lies in several key aspects:
- Foundation for Complex Operations: Exponentiation serves as the foundation for more complex mathematical operations and algorithms. Many algorithms in fields such as cryptography, numerical analysis, and data science heavily rely on exponentiation for their functionality;
- Essential for Algorithm Design: Understanding the principles of exponentiation is essential for designing efficient algorithms. Algorithms often involve raising numbers to different powers, and having a solid grasp of exponentiation allows programmers to devise optimized solutions;
- Debugging and Optimization: Proficiency in exponentiation aids in debugging and optimizing code. Being able to accurately implement exponentiation ensures that mathematical computations are performed correctly, reducing the likelihood of errors and improving overall code performance.
Examples of Exponentiation in Python Programming
Exponentiation is not only limited to basic arithmetic operations but also finds application in various computational tasks. Some examples include:
- Exponential Growth: Modeling population growth, compound interest, or the spread of infectious diseases often involves exponentiation;
- Matrix Operations: Exponentiation is utilized in matrix operations for tasks such as matrix exponentiation, matrix power, and solving systems of linear equations;
- Cryptographic Operations: Exponentiation plays a crucial role in cryptographic algorithms, such as RSA encryption, where large numbers are raised to specific powers modulo another number.
Comparing Python with Other Programming Languages
Programming languages provide different mechanisms for performing exponentiation, each with its syntax and conventions. In this comparison, we’ll delve into how Python’s exponentiation operator compares with those in Java, JavaScript, and C++.
Python
Python’s exponentiation operator is concise and intuitive, using the double asterisk (**) notation. This operator simplifies the process of raising a number to a certain power, offering a straightforward syntax. For example:
result = 2 ** 3 # Computes 2 raised to the power of 3, resulting in 8
The double asterisk operator (**), also known as the power operator, is widely used in Python due to its simplicity and readability.
Java
In Java, exponentiation is achieved using the Math.pow(x, y) method. This method requires two arguments: the base (x) and the exponent (y), and returns the result as a double value. Here’s how it’s implemented:
double result = Math.pow(2, 3); // Computes 2 raised to the power of 3, resulting in 8.0
Java’s approach to exponentiation involves invoking a predefined method from the Math class, providing flexibility but requiring additional syntax compared to Python.
JavaScript
JavaScript, similar to Python, employs the double asterisk operator (**) for exponentiation. This operator offers a concise and familiar syntax for calculating powers. For instance:
let result = 2 ** 3; // Computes 2 raised to the power of 3, resulting in 8
JavaScript’s usage of the double asterisk operator aligns with Python, enhancing code readability and developer familiarity.
C++
Unlike Python and JavaScript, C++ lacks a built-in exponentiation operator. Instead, developers typically utilize the pow(x, y) function from the cmath or math.h library. This function computes the power of a specified base raised to a given exponent. Here’s how it’s implemented:
#include <iostream>
#include <cmath>
int main() {
double result = pow(2, 3); // Computes 2 raised to the power of 3, resulting in 8
std::cout << "Result: " << result << std::endl;
return 0;
}
C++ requires importing libraries and using a separate function call for exponentiation, adding complexity compared to Python and JavaScript.
Comparison Table
To summarize the differences between these languages’ exponentiation operators, let’s use a comparison table:
Language | Operator | Example | Result |
---|---|---|---|
Python | ** | 2 ** 3 | 8 |
Java | Math.pow(x, y) | Math.pow(2, 3) | 8.0 |
JavaScript | ** | 2 ** 3 | 8 |
C++ | pow(x, y) | pow(2, 3) | 8 |
Conclusion
In answering the query: which mathematical operator is used to raise 5 to the second power in Python, we’ve explored the ‘**’ operator’s syntax, applications, and relevance in various programming scenarios. This knowledge is foundational in Python programming and essential for computational tasks across many fields. As we’ve seen, Python’s approach to exponentiation is intuitive, aligning well with mathematical notation, making it accessible for beginners and efficient for experienced programmers.
FAQ
Yes, Python handles negative exponents. For example, 5 ** -2 results in 0.04.
Yes, the former is an integer operation, while the latter gives a floating-point result.
Yes, Python supports complex numbers in exponentiation operations.