Python, high level, general purpose, and powerful programming language, has its main attraction in its simplicity and readability. One essential point that affects the Python readability is its way of arguments handling in function calls. In the following piece, we’ll be discussing the critical aspect of “what a positional argument in Python is”, focusing on its meaning, the way you use it, and its peculiarities.
The Basics of Positional Arguments
In Python programming, positional arguments are fundamental in using functions and the underlying concept must be understood. Positional parameters are a class of function parameters with the input values order influencing their behavior. To be able to master argparse which needs position arguments, one should know the basics of the function arguments in Python.
Function Arguments in Python
When defining python functions you usually specify the parameters inside the definition. These parameters are placeholders for the values that will be provided while the function is invoked. These values are arguments which are passed to the function along with its invocation. Consider the following Python function definition:
def greet(first_name, last_name):
print(f"Hello, {first_name} {last_name}!")
In this function greet, first_name and last_name are parameters that require values to be passed when the function is called. Let’s examine the correct usage of positional arguments with an example:
# Correct usage of positional arguments
greet("John", "Doe")
In the example given above the “John” and “Doe” are positional arguments. They are passed to the greet function as first_name and last_name in the order expected by the parameters. For instance, “John” is stored in variable first_name while “Doe” is stored in variable last_name.
Why Use Positional Arguments?
Positional arguments in Python play a pivotal role in enhancing the clarity, simplicity, and logical flow of function calls. They are essential components of Python programming, offering several benefits to developers. Let’s delve deeper into the significance of using positional arguments:
Clarity Enhances Readability and Comprehensibility
Positional arguments contribute significantly to the readability and comprehensibility of function calls. They provide a clear indication of which value corresponds to each parameter, thus minimizing ambiguity and confusion. When developers use positional arguments, it becomes easier to discern the purpose and behavior of the function. This clarity simplifies the understanding of code, making it more accessible to developers who may need to maintain or debug it in the future. Consider the following function call with positional arguments:
result = calculate_area(length, width)
In this example, the positional arguments length and width directly correspond to the parameters of the calculate_area function. This straightforward syntax enhances readability and aids in understanding the function’s purpose without the need for explicit parameter names.
Simplicity Streamlines Function Calls
One of the primary advantages of positional arguments lies in their simplicity. Unlike keyword arguments, which necessitate specifying parameter names along with values, positional arguments allow developers to pass arguments directly without explicitly mentioning parameter names. This streamlined syntax reduces the cognitive load on developers and simplifies the process of calling functions. Let’s compare function calls with positional and keyword arguments:
Positional arguments:
result = calculate_area(length, width)
Keyword arguments:
result = calculate_area(length=length_value, width=width_value)
In the positional argument example, developers can pass values directly without specifying parameter names, making the function call concise and straightforward.
Order Matters for Logical Integrity
Positional arguments enforce a specific order of arguments, which is essential for maintaining the integrity and logic of functions. The order in which arguments are passed directly influences the behavior and output of a function. By adhering to the prescribed order of positional arguments, developers ensure that the function operates as intended and produces accurate results. Consider a function that calculates the total cost based on the unit price and quantity:
total_cost = calculate_total_cost(unit_price, quantity)
In this example, the order of positional arguments (unit_price followed by quantity) is crucial. Switching the order would lead to incorrect results, highlighting the importance of maintaining the specified sequence.
To illustrate the importance of positional arguments, consider the following example:
def calculate_area(length, width):
"""
Calculate the area of a rectangle.
Parameters:
length (float): Length of the rectangle.
width (float): Width of the rectangle.
Returns:
float: Area of the rectangle.
"""
return length * width
# Function call using positional arguments
area = calculate_area(5, 3)
print("Area of the rectangle:", area)
In this example, the function calculate_area accepts two positional arguments: length and width. The order in which these arguments are passed (length first, then width) is crucial for obtaining the correct area of the rectangle.
Positional Arguments vs. Keyword Arguments
Understanding the distinctions between these two types of arguments is crucial for writing clear and effective code. Let’s delve into the comparison between positional arguments and keyword arguments to gain a comprehensive understanding of their differences and use cases:
Positional Arguments
Positional arguments are passed to a function in a specific order defined by the function’s parameter list. Their values are assigned based on their position in the function call. Key characteristics of positional arguments include:
- Order Dependency: The order in which positional arguments are passed must match the order of parameters in the function definition;
- No Explicit Naming: Positional arguments do not require specifying parameter names during the function call.
python
Copy code
def greet(first_name, last_name):
"""
Function to greet a person.
Parameters:
first_name (str): First name of the person.
last_name (str): Last name of the person.
"""
print("Hello", first_name, last_name)
# Function call using positional arguments
gre
Keyword Arguments
Keyword arguments allow developers to specify parameter names along with their values during function calls. Unlike positional arguments, the order of keyword arguments is not significant. Key features of keyword arguments include:
- Parameter Naming: In keyword arguments, parameters are explicitly named, which enhances readability and allows for flexibility in argument order;
- Order Independence: Keyword arguments can be passed in any order since their values are associated with parameter names.
# Function call using keyword arguments
greet(last_name="Doe", first_name="John")
Comparison
Let’s compare positional arguments and keyword arguments based on several factors:
Factor | Positional Arguments | Keyword Arguments |
---|---|---|
Order Dependency | The order of arguments matters. | The order of arguments is not significant. |
Naming | No explicit naming required. | Parameters are explicitly named. |
Readability | May lead to confusion if the order is not apparent. | Enhances readability due to explicit parameter names. |
Flexibility | Limited flexibility in argument order. | Allows flexibility in argument order. |
Example | greet(“John”, “Doe”) | greet(last_name=”Doe”, first_name=”John”) |
Mixing Positional and Keyword Arguments
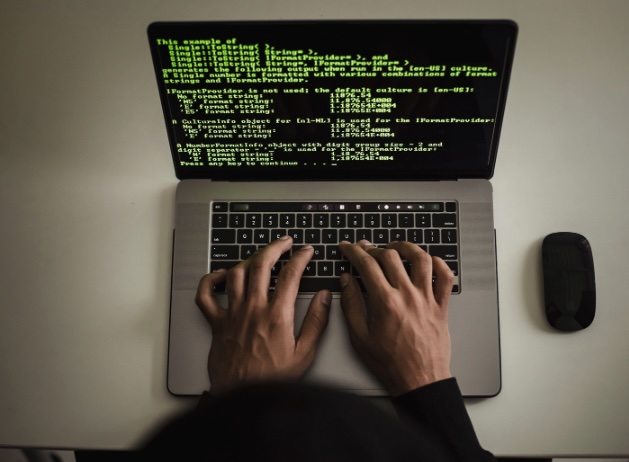
In Python, functions offer the flexibility to accept a combination of both positional and keyword arguments. This feature enhances the versatility and usability of functions, allowing developers to tailor function calls to their specific needs. However, it’s essential to understand the rules governing the usage of positional and keyword arguments, particularly when mixing them within a single function call.
Mixing Positional and Keyword Arguments
When mixing positional and keyword arguments in Python functions, there are certain rules that must be followed to ensure proper syntax and execution. The key points to remember are:
- Positional Arguments First: Positional arguments must always precede keyword arguments in function calls. This means that all arguments without explicit parameter names must be provided before any arguments with parameter names;
- Parameter Correspondence: When using a mix of positional and keyword arguments, it’s crucial to ensure that each argument corresponds correctly to its respective parameter in the function definition. Failure to do so may lead to unexpected behavior or errors.
To illustrate this concept, consider the following example:
def mix_example(a, b, c):
"""
Function to demonstrate mixing positional and keyword arguments.
Parameters:
a (int): First parameter.
b (int): Second parameter.
c (int): Third parameter.
"""
print(a, b, c)
# Correct usage of mixing positional and keyword arguments
mix_example(1, b=2, c=3)
# Incorrect usage (will raise a SyntaxError)
# mix_example(a=1, 2, 3)
In the correct usage example, positional argument 1 is followed by keyword arguments b=2 and c=3, adhering to the rule of positional arguments preceding keyword arguments.
Conversely, the incorrect usage attempts to provide a positional argument (2) after a keyword argument (a=1). This violates the rule of positional arguments coming before keyword arguments and will result in a SyntaxError.
Benefits of Mixing Positional and Keyword Arguments
By allowing a mix of positional and keyword arguments, Python provides developers with enhanced flexibility and expressiveness when calling functions. This feature offers the following benefits:
- Customization: Developers can customize function calls based on their requirements, using a combination of positional and keyword arguments to tailor the behavior of the function;
- Clarity: Mixing positional and keyword arguments can improve the readability and clarity of function calls by providing additional context through explicit parameter names;
- Adaptability: The ability to mix positional and keyword arguments makes functions more adaptable to different use cases and scenarios, increasing their reusability and versatility.
Advanced Concepts
Advanced concepts like positional-only parameters and variadic positional arguments offer developers powerful tools for creating flexible and expressive functions. These features, introduced in Python 3.8 and earlier versions, respectively, enhance the versatility and usability of functions, enabling developers to handle a wide range of scenarios effectively.
Positional-Only Parameters
Positional-only parameters, introduced in Python 3.8, allow developers to specify parameters that can only be passed positionally, prohibiting their use as keyword arguments. This is denoted by using a forward slash / in the function definition. Key points about positional-only parameters include:
- Syntax: Positional-only parameters are indicated by placing a / before the parameters in the function definition;
- Usage: These parameters can only be provided as positional arguments during function calls, not as keyword arguments.
def pos_only_arg(arg, /):
"""
Function with positional-only parameter.
Parameters:
arg: Positional-only parameter.
"""
print(arg)
# Correct usage
pos_only_arg(10)
# Incorrect usage (will raise a TypeError)
# pos_only_arg(arg=10)
In the provided example, the function pos_only_arg accepts a single positional-only parameter arg. Attempts to pass this parameter as a keyword argument will result in a TypeError.
Variadic Positional Arguments
Variadic positional arguments, often referred to as “args” in Python, allow functions to accept an arbitrary number of positional arguments. This capability is useful when the exact number of arguments is unknown or can vary. Key points about variadic positional arguments include:
- Syntax: Variadic positional arguments are denoted by using the *args syntax in the function definition, where args is a tuple containing all provided positional arguments;
- Usage: Functions with variadic positional arguments can accept any number of positional arguments during function calls.
def multiple_args(*args):
"""
Function to handle multiple positional arguments.
Parameters:
*args: Variadic positional arguments.
"""
for arg in args:
print(arg)
# Function call with multiple positional arguments
multiple_args(1, 2, 3, 4)
In this example, the function multiple_args accepts a variadic positional argument *args, allowing it to receive any number of positional arguments provided during the function call.
Benefits and Use Cases
This feature offers the following benefits:
- Flexibility: Positional-only parameters and variadic positional arguments provide developers with greater flexibility in function design, enabling them to handle diverse input scenarios;
- Expressiveness: These advanced concepts contribute to the expressiveness of Python code, allowing developers to write concise and elegant functions;
- Error Prevention: Positional-only parameters help prevent potential errors caused by misusing parameters as keyword arguments, enhancing code robustness.
Conclusion
Positional arguments in Python are a fundamental part of function definition and calling. They provide a straightforward way to pass data to functions, enforce a clear contract between the function and the caller, and contribute to Python’s overall readability and simplicity. Understanding what a positional argument in Python is not only helps in writing better code but also in appreciating the design choices of Python as a language.
FAQ
Yes, but positional arguments must come before any keyword arguments in the function call.
Python will raise a TypeError, indicating that the function is missing required positional arguments.
It depends on the function definition. If the function defines positional parameters, then they are mandatory unless default values are provided.
Yes, by setting default values for them in the function definition.
Technically, there is no hard limit. However, for readability and maintainability, it’s advisable to keep the number reasonable.
They are not as common as regular positional arguments but are used in specific scenarios to prevent ambiguity.