Python, the versatile and widely used programming language, offers various ways to exit a function. Understanding these methods is crucial for writing efficient and effective code. In this article, we’ll explore different approaches to exiting functions in Python, including how to pass a list into a function, apply a function to a list, mock a function, and test lambda functions locally.
Basics of Exiting a Function in Python
Exiting a function in Python is a fundamental concept in programming. It involves controlling the flow of your code within a function and, in some cases, returning a value to the calling code. In this discussion, we will explore the basics of exiting a function in Python.
Using the Return Statement
In Python, the return statement is one of the main ways to end a program. The return line ends the function and lets you send a value back to the code that called it. This is an example:
def example_function():
# Some code
return “Hello, World!”
In this example, when the function example_function() is called, it will execute the code within the function and then return the string “Hello, World!”. The calling code can capture and use this returned value as needed.
How to Pass a List into a Function in Python
Passing a list into a function in Python is a common operation when you want to perform operations on a set of data. It’s a straightforward process that involves declaring a function with a parameter that represents the list and then passing the list when calling the function.
Example of Passing a List to a Function
def process_list(my_list):
# Process the list
return sum(my_list)
numbers = [1, 2, 3, 4, 5]
result = process_list(numbers)
In this example, the function process_list() takes a single parameter, my_list, which represents the list of numbers. When the function is called with the numbers list, it calculates the sum of the numbers in the list using the sum() function and returns the result. The result variable will contain the sum of the numbers.
Applying a Function to a List in Python
Applying a function to each element in a list is a common task in Python programming. There are several methods to achieve this, including using a for loop, the map function, or list comprehensions. We will explore each of these methods.
Utilizing a For Loop
One of the fundamental methods to implement a function on each item within a list is through the application of a for loop. Let’s delve into an illustrative example:
def square(number):
return number * number
numbers = [1, 2, 3, 4, 5]
squared_numbers = []
for num in numbers:
squared_numbers.append(square(num))
In the code snippet above, we have defined a simple square() function designed to calculate the square of a given number. A list called numbers has also been established, containing a sequence of integers from 1 to 5. The empty list squared_numbers is initialized to store the squared values.
Within the subsequent for loop, each element in the numbers list undergoes the square function transformation, and the resultant squared values are systematically appended to the squared_numbers list. This process continues iteratively until all elements have been processed.
Leveraging the map Function
Alternatively, you can employ the map() function as a more succinct and efficient approach to apply a function to each element within a list. The map() function returns an iterable, which can be effortlessly converted into a list, containing the outcomes of applying the designated function to each element:
squared_numbers = list(map(square, numbers))
In the provided code snippet, the map() function seamlessly applies the square() function to each element within the numbers list, systematically producing a list of squared numbers as a result.
Harnessing List Comprehensions
Another elegant and easily readable method for generating lists while applying a function to each element of an existing list is through the utilization of list comprehensions:
squared_numbers = [square(num) for num in numbers]
In this example, the list comprehension iterates through the numbers list, systematically applying the square() function to each element. The result is a list that contains the squared values, created in a concise and efficient manner.
How to Mock a Function in Python
Mocking a function in Python is a crucial technique, primarily used in unit testing. It involves substituting a real function with a fake version that simulates its behavior. This allows you to isolate and test specific parts of your code without relying on the actual implementation of the function. Let’s delve into the details of how to mock a function in Python.
Using unittest.mock
The unittest.mock module provides tools for mocking functions and objects in Python. In the context of unit testing, you can use the MagicMock class to create a mock function with predefined behavior. Here’s an example:
from unittest.mock import MagicMock
def test_my_function():
# Create a mock function that returns 10
external_function = MagicMock(return_value=10)
# Perform your test using the mock
assert external_function() == 10
In this example, external function is a mock function that simulates the behavior of a real function. You can define its return value using return_value, and when you call external_function(), it will return 10 as specified.
Mocking is especially useful when you want to control the behavior of external dependencies, such as API calls or database access, during unit testing.
How to Close a Function in Python
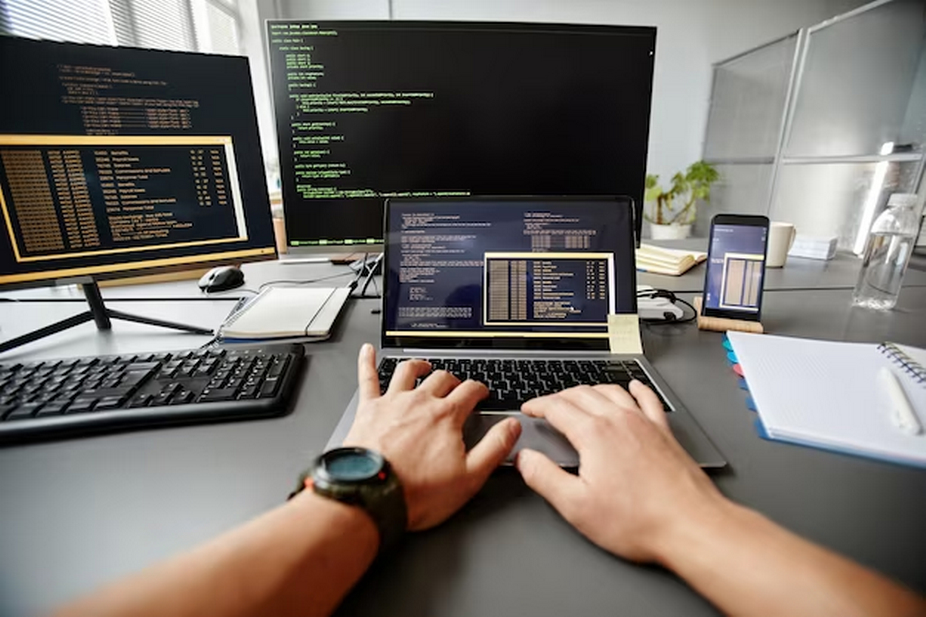
Closing a function in Python typically refers to ensuring that a function exits correctly and performs any necessary cleanup tasks. This is particularly important in the context of resource management, such as file handling or database connections. Let’s explore how to close a function in Python effectively.
Closing a File Handling Function
def read_file(file_path):
# Open the file for reading
with open(file_path, ‘r’) as file:
data = file.read()
# The function closes here, releasing the file resource
return data
In this example, the read_file() function opens a file specified by file_path for reading using the with statement. The with statement ensures that the file is automatically closed when the block of code inside it is exited, even if an exception occurs. This is a best practice for handling files in Python, as it guarantees that resources are released properly.
Properly closing functions is not limited to file handling; it also applies to other resource management tasks like database connections, network sockets, or even cleaning up data structures to prevent memory leaks.
How to Test Lambda Function Locally in Python
Testing lambda functions locally in Python is crucial, especially when you’re developing serverless applications that rely heavily on these small, self-contained units of code. Local testing allows you to debug and validate your lambda functions before deploying them to a serverless environment like AWS Lambda. Here’s how you can test a lambda function locally:
Testing a Lambda Function
# Define a lambda function
lambda_function = lambda x: x * x
# Test the lambda function locally
assert lambda_function(5) == 25
In this example, we define a simple lambda function that squares its input. To test it locally, we call the lambda function with an argument of 5 and assert that the result is 25. This demonstrates how you can quickly verify the behavior of a lambda function without the need for deployment.
Local testing of lambda functions can save time and resources by catching errors and ensuring that your code works as expected before deploying it to a serverless environment.
Conclusion
By understanding these key aspects of Python functions, from how to pass a list into a function to testing lambda functions locally, you can write more efficient and effective Python code. Remember, practice and experimentation are key to mastering Python programming!
FAQ
Q: What is the best way to end a Python function?
A: Python function termination strategies are complex, depending on the function’s goals and needs. The discerning coder must negotiate this terrain.
Q: Can Python functions return many values?
A: Python’s diverse arsenal lets functions return a tuple of values. This amazing power lets developers effortlessly explain multiple outcomes.
Q: How do you test a Python function that interacts with external entities?
A: The cutting-edge method for analyzing a Python code caught in a complex web of external resources is’mocking.’ By simulating these extrinsic components, developers can control scenarios and test the function.
Q: Can Python handle multiple lists with its function-receiving skills?
A: Appending multiple lists to Python’s function invocations is neither difficult nor difficult. Any skilled developer may do this by adding parameters.
Q: Python lambda functions—what are they?
A: Python’s mysterious lambda functions remain anonymous. These tiny constructions, announced with the arcane ‘lambda’ incantation, exude succinctness and excel at simple jobs.