The popular and easy-to-understand programming language Python provides a number of string manipulation capabilities, one of which is the ability to escape commas from a string. Python programmers of all skill levels will find something useful in this article’s exploration of the many approaches of stripping strings of commas.
Understanding String Manipulation in Python
One must be familiar with the fundamentals of string manipulation in Python before delving into the details of string manipulation, such as how to delete a comma. Strings written using Python cannot be modified in any way once created; this is known as their immutability. On the other hand, we can modify existing strings to generate new ones.
Immutable Nature
Once a string is formed in Python, its contents cannot be directly edited since strings are immutable. Data integrity is ensured by the immutability of strings, which is a critical property. Python preserves the integrity of the old string while creating a new one with the updated content. As an illustration:
original_string = “Hello, World!”
modified_string = original_string.replace(“,”, “”)
print(original_string) # Output: “Hello, World!”
print(modified_string) # Output: “Hello World!”
In this example, original_string remains unchanged, and a new string modified_string is created without the comma.
Indexing and Slicing
You need to know how to get to particular characters or parts of strings if you want to modify strings properly. By utilizing indexing, Python enables you to get specific characters within a string. As an example, the first character is at index 0, the second at index 1, and so on in Python’s zero-based indexing. The following is a string indexing example:
my_string = “Python”
first_character = my_string[0] # Access the first character
print(first_character) # Output: “P”
Additionally, Python supports string slicing, which allows you to extract a portion of a string by specifying a start and end index. For instance:
my_string = “Python Programming”
substring = my_string[7:18] # Slice from index 7 to 17 (inclusive)
print(substring) # Output: “Programming”
Slicing is particularly useful when you need to work with substrings within a larger string.
Concatenation
A new string can be created by concatenating two or more strings. You may connect strings in Python by simply using the plus sign (+) or the str.join() method.
When using the plus sign:
string1 = “Hello, “
string2 = “World!”
result = string1 + string2
print(result) # Output: “Hello, World!”
Using the str.join() method:
strings = [“Hello”, “World”]
result = “, “.join(strings)
print(result) # Output: “Hello, World”
Concatenation is a fundamental operation in string manipulation and is often used to build strings dynamically.
Methods to Remove Commas from Strings in Python
Several methods exist in Python to remove commas from strings. We’ll explore these methods with examples to understand how to remove commas effectively.
Method 1: Using replace()
The replace() method is one of the easiest ways to remove commas from Python strings. This Python string class function searches the string for a comma and replaces it with an empty string.
Example:
original_string = “Hello, World!”
modified_string = original_string.replace(“,”, “”)
print(modified_string) # Output: Hello World!
You can see an example of using the replace() method to remove commas from the original_string in the code snippet above. After removing all commas from the original text, the resultant modified_string is identical to the original. For simple string manipulation jobs, this approach is perfect because it is clear and simple to grasp.
Method 2: Using translate()
To remove characters, including commas, from a string in a more sophisticated way, you can use the translate() method. One useful feature is the ability to generate translation tables that outline which characters can be substituted or removed in a single run.
Example:
original_string = “Hello, World!”
translation_table = original_string.maketrans(“”, “”, “,”)
modified_string = original_string.translate(translation_table)
print(modified_string) # Output: Hello World!
Here, we start by making a translation table with the maketrans() function; we tell it to eliminate any and all instances of the comma. The original string is translated into the changed string using this translation table. Similar to the first example, this one also gets rid of commas.
For simultaneous removal of several characters from a string, the translate() function shines. It provides an effective and potent method for dealing with such situations.
Method 3: Using List Comprehensions
You may generate lists with minimal code using list comprehensions, a succinct and expressive feature in Python. By generating a list of characters that do not correspond to the ones that need to be deleted, they can also be used to extract particular characters, like commas, from strings.
Example:
original_string = “Hello, World!”
modified_string = ”.join([char for char in original_string if char != ‘,’])
print(modified_string) # Output: Hello World!
Here, we go through the original string character by character using a list comprehension. Before adding a character to the list, we verify that it is not a comma using the condition char!= ‘,’. Last but not least, we remove all commas from the modified_string by merging the characters from the list using the join() function.
A more compact and Pythonic approach to string removal is possible with list comprehensions, which offer an elegant and legible solution.
Method 4: Using Regular Expressions
One of Python’s most useful features for working with strings is regular expressions, or regex. Their pattern-matching and manipulation capabilities make it possible to design intricate string structures. By defining a pattern that matches the comma character and then replacing it with an empty string, regex makes removing commas a simple process.
Example:
import re
original_string = “Hello, World!”
modified_string = re.sub(“,”, “”, original_string)
print(modified_string) # Output: Hello World!
To begin, we bring up the re module, which is a Python package that helps with regular expressions. Then, for every comma in original_string, we use the re.sub() function to replace it with an empty string. As a result, the modified_string is created without any commas.
When it comes to manipulating strings, regular expressions provide the maximum versatility. Their use becomes crucial in handling intricate situations involving the removal or manipulation of certain patterns inside strings.
Common Pitfalls in String Manipulation
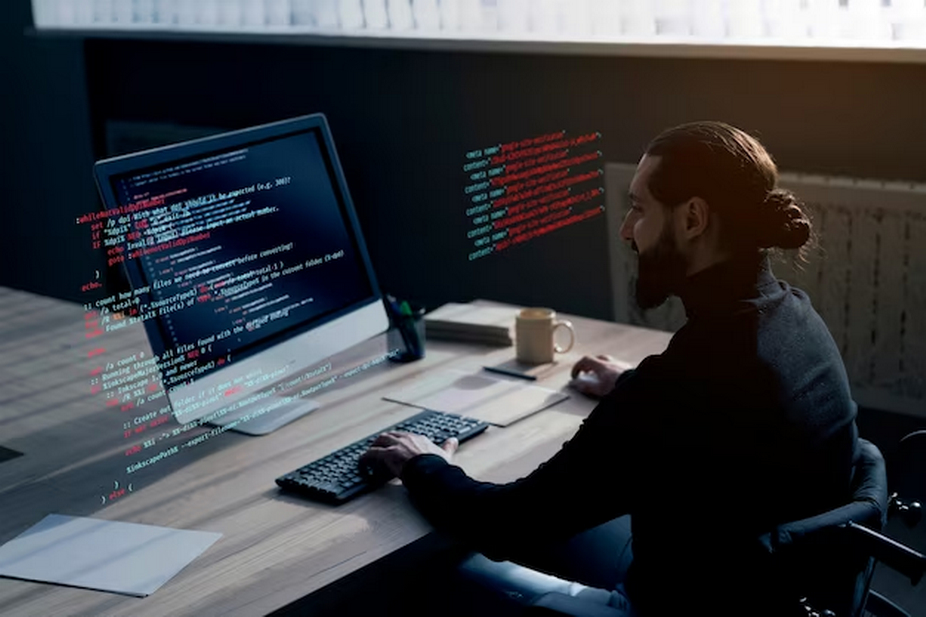
Fundamental to computer programming, string manipulation entails a number of operations on text data, including joining, splitting, searching, and replacing. Despite appearances, programmers frequently run into problems while dealing with strings. In this detailed tutorial, we’ll look at these problems and offer solutions to help you create efficient and error-free code for string manipulation.
Indexing Errors
Indexing issues are common when working with strings and can cause inaccurate results or even runtime faults. When you try to access characters in a string using the wrong indices, several issues happen. Keep in mind that the initial character of a string is at index 0 in many programming languages, which can lead to this trap.
Example:
text = “Hello, World!”
# Incorrect: Accessing the 14th character, which doesn’t exist
character = text[14]
# Correct: Accessing the 13th character
character = text[13]
Forgetting About String Immutability
In many programming languages, strings cannot be altered once formed; this property is known as immutability. A new string object will be created if you try to edit a string directly. Code that isn’t efficient might result from ignoring its immutability.
Example:
text = “Hello, World!”
# Incorrect: Attempting to modify the string
text[0] = ‘h’ # Raises an error
# Correct: Creating a new string with the desired modification
new_text = “hello” + text[1:]
Inefficient Concatenation
When working with long strings, concatenating them in a loop could drastically reduce speed. It could take a while for each concatenation operation to finish because it generates a new string.
Example:
# Incorrect: Inefficient string concatenation in a loop
result = “”
for i in range(1000):
result += str(i)
# Correct: Using a list to collect parts of the string and then joining them
parts = []
for i in range(1000):
parts.append(str(i))
result = ”.join(parts)
Not Handling Encoding and Character Set Issues
Dealing with various character encodings and sets makes string manipulation more complex. Incorrect handling of these concerns can result in corrupted data and weird behavior.
Best Practices for String Manipulation in Programming
Programming relies heavily on string manipulation, which includes tasks like finding, replacing, concatenating, and separating strings. For many tasks, including data processing and text processing, efficient string manipulation is required. Highlighting efficiency, error prevention, and data integrity, this guide will discuss best practices for string manipulation.
Use String Methods
For common string operations, most computer languages have built-in string methods. Using these methods instead of writing your own code is a good idea for several reasons:
- Efficiency: Custom implementations of string methods are not always as fast as the built-in ones;
- Readability: For readability’s sake, string methods are usually preferable to custom code because they are shorter and simpler;
- Error Reduction: The built-in procedures are less likely to make mistakes because they have been tried and proven.
Here’s an example in Python:
text = “Hello, World!”
# Using built-in methods
uppercase_text = text.upper() # Convert to uppercase
words = text.split() # Split into words
To make the text all capital letters, we use the upper() function, and to break it up into words, we use the split() technique. It is a global best practice since similar approaches are available in other programming languages.
Validate Input Data
To avoid making mistakes that you wouldn’t expect, check the input data before manipulating strings. Accidental or erroneous input might cause accidents or produce inaccurate outcomes. Standard validation procedures consist of:
- Checking for null or empty strings.
- Ensuring that the data conforms to your expectations, such as type checking.
Here’s an example in JavaScript:
function manipulateString(input) {
if (typeof input === ‘string’ && input.length > 0) {
// Perform string manipulation
} else {
console.error(‘Invalid input data’);
}
}
In this JavaScript function, before performing any string modification, we verify that the input is not an empty string. Strong programming relies on accurate input validation.
Use StringBuilder or Equivalent
A StringBuilder or its programming language equivalent should be considered whenever string concatenation is required, whether in a loop or for numerous strings. An effective way to concatenate strings is via StringBuilder, a data structure. When compared to the inefficient method of naïve string concatenation, it drastically improves efficiency while decreasing memory overhead.
Here’s an example in Java:
// Efficient string concatenation using StringBuilder
StringBuilder result = new StringBuilder();
for (int i = 0; i < 1000; i++) {
result.append(i);
}
String finalResult = result.toString();
To efficiently concatenate numbers in a loop, we utilize a StringBuilder in this Java example. If you’re working with huge datasets, using StringBuilder or anything similar will keep your code performant.
Handle Encoding and Character Set Properly
Character encoding and character set concerns must be carefully considered before dealing with text data. Data corruption and unexpected behavior might occur from improper handling of encoding and character set compatibility. Some guidelines for efficient encoding management are as follows:
- When working with text files, it is imperative that you always use the correct encoding;
- If you need to convert encodings, you can use libraries or methods that do that;
- Exercise caution and check for correct encoding support while dealing with multilingual text.
Comparison of Methods
Method | Use Case | Complexity |
replace() | Simple replacement of a specific character | Low |
translate() | Removal or replacement of multiple characters | Medium |
List Comprehension | Custom logic for character removal | Medium |
Regular Expressions | Complex pattern matching and replacement | High |
Conclusion
Removing commas from a string in Python can be achieved through various methods, each with its own advantages. Whether you’re a beginner or an experienced coder, understanding how to remove a comma from a string in Python enhances your string manipulation skills and broadens your Python programming proficiency.
FAQ
Q1: Can these methods remove commas from all string formats?
Yes, these methods work on any string format in Python, whether it’s a single word, sentence, or multiline text.
Q2: Is it necessary to import any modules for these methods?
For the replace(), translate(), and list comprehension methods, no additional modules are needed. However, for using regular expressions, the re module must be imported.
Q3: How do I handle removing commas from a large text file?
For large text files, read the file line by line and apply the chosen method to remove commas. This approach is memory efficient.
Q4: Can these methods be used to remove other characters besides commas?
Absolutely! You can modify these methods to remove or replace any character in a string.