In the enchanting world of programming, blending different types of ingredients to create something new and useful is a daily adventure. In Python, one of the most common alchemical processes is concatenating strings and integers. This article will serve as your guide through the captivating journey of how to concatenate string and int in Python, explaining the process with clear examples, helpful tables, and answering some of the most frequently asked questions.
The Basics: What Does Concatenation Mean in Python?
Concatenation can be thought of as a digital form of “gluing” or “binding” data together. When you concatenate a string and an integer in Python, you are essentially joining a sequence of characters with a numerical value to create a new string.
Python provides a straightforward and intuitive way to perform concatenation using the + operator. This operator allows you to combine strings and integers to generate the desired output. Let’s explore why you might need to concatenate string and int in Python and how it can be applied in various scenarios.
Why Concatenate String and Int in Python?
Concatenating strings and integers in Python serves various purposes, making it a fundamental operation in many programming tasks. Here are some common reasons for combining these two data types:
Data Presentation
A major purpose for concatenating texts and numbers is data display. Text messages and user interfaces may need numerical numbers. If your gaming application monitors user scores, you may need to display “Your score is 100 points.” To make a user-friendly result, concatenate the integer (score) with the string (message).
Example:
score = 100 message = “Your score is ” + str(score) + ” points.” print(message) |
Output:
Your score is 100 points. |
File and URL Handling
Concatenation is valuable when generating filenames or URLs dynamically. Suppose you are writing a script that saves files with names that include a timestamp or a unique identifier. By combining strings and integers, you can create these filenames programmatically.
Example:
import datetime file_name = “data_” + str(datetime.datetime.now().timestamp()) + “.csv” print(file_name) |
Output (depending on the current timestamp):
data_1642394823.72956.csv |
Data Logging
Concatenating strings and integers is also crucial for creating informative log messages that include numerical data. When you’re developing software, logging is a vital part of debugging and monitoring. You might want to log messages that provide context by including numeric values, such as timestamps, error codes, or counters.
Example:
error_code = 404 log_message = “Error ” + str(error_code) + “: Page not found.” print(log_message) |
Output:
Error 404: Page not found. |
The Concatenation Spell: Simple Methods to Merge String and Int
Let’s explore several methods for merging strings and integers in Python, focusing on the usage of the + operator, f-strings, the format() method, the str.join() method, and the % operator. Each of these methods has its own strengths and use cases, which we will discuss in detail.
Using the + Operator
The simplest way to join two Python objects, a string and an integer, is using the plus operator. The str() function can be used to convert the number to a string, and then the + operator can be used to join them.
Example:
result = ‘Score: ‘ + str(100) |
This method is simple and effective, making it suitable for basic concatenation needs.
Formatted String Literals (f-strings)
Introduced in Python 3.6, f-strings provide a cleaner and more readable way to create strings that incorporate variables. You can directly embed expressions inside string literals using curly braces {} and prefix the string with ‘f’ or ‘F’.
Example:
result = f’Score: {100}’ |
F-strings are a modern and preferred method for string concatenation due to their simplicity and readability.
Using the format() Method
The format() method gives a more versatile technique to concatenate texts and numbers, especially when you need complicated formatting. The format() method can be used to replace placeholders within the string with the desired values.
Example:
result = ‘Score: {}’.format(100) |
This method is flexible and allows for more advanced formatting options, making it suitable for complex use cases.
The str.join() Method
The str.join() method is useful when you need to concatenate multiple strings, including integers. It takes an iterable of strings and combines them into a single string.
Example:
result = ”.join([‘Score: ‘, str(100)]) |
This method is efficient for joining lists of strings, making it handy for situations where you have multiple components to concatenate.
String Interpolation with % Operator
The % operator is an older method but still functional for string interpolation. It allows you to insert variables into a string using placeholders. The %d format code is used for integers.
Example:
result = ‘Score: %d’ % 100 |
While this method is less favored in modern Python due to the advent of f-strings, it is still widely used and supported.
Comparing Methods: A Quick Table
Method | Syntax | Ease of Use | Versatility |
+ Operator | ‘text’ + str(number) | Simple | Basic |
f-strings | f’text {number}’ | Easy | High |
format() Method | ‘text {}’.format(number) | Moderate | High |
str.join() Method | ”.join([‘text’, str(number)]) | Moderate | Moderate |
% Operator | ‘text %d’ % number | Moderate | Moderate |
Advanced Concatenation: Handling Complex Scenarios
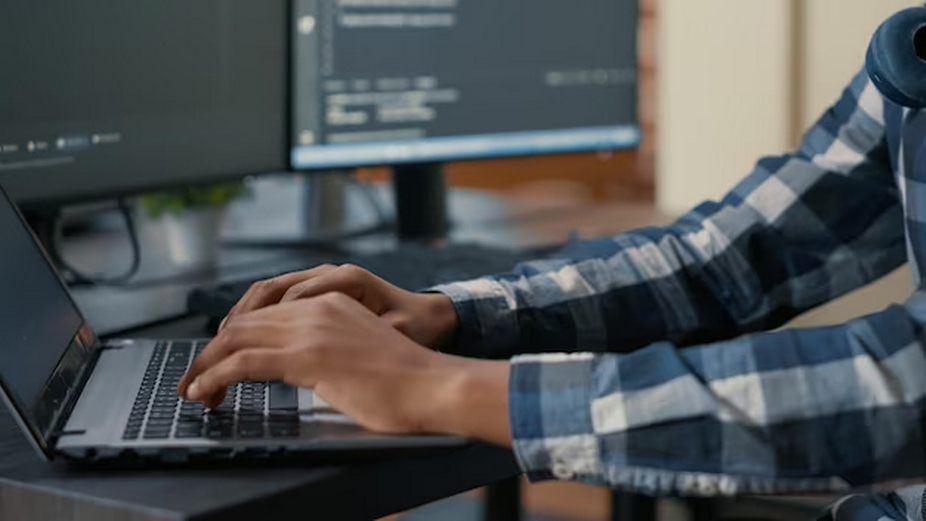
Sometimes, you’ll encounter situations where simple concatenation doesn’t cut it. Here are some tips for handling such cases:
Concatenating Lists and Tuples
When dealing with lists and tuples, it’s crucial to convert each element to a string before concatenation. This process ensures that disparate data types within these structures can be seamlessly combined into a single string. For instance, if you have a list of numbers and strings, converting the numbers to strings ensures they can be concatenated with text. Here’s a Python example:
my_list = [1, “apple”, 3.14, True] concatenated_string = ”.join(str(item) for item in my_list) |
Handling Non-English Text
Concatenating non-English text, especially when it contains special characters or accents, requires careful attention to encoding. Incorrect encoding can lead to character corruption or unreadable output. UTF-8 encoding is commonly used for handling a wide range of character sets. Ensure that your text data is properly encoded before concatenating it with other strings to maintain data integrity.
Dealing with Large Numbers
In scenarios involving large numbers, such as financial data or population statistics, it’s advisable to improve readability by adding commas as thousands separators. This not only enhances the visual clarity of the numbers but also makes them more understandable to users. Many programming languages offer built-in functions or libraries to format large numbers with commas, simplifying the process significantly. For instance, in Python, you can use the locale module to achieve this:
import locale number = 1000000 formatted_number = locale.format_string(“%d”, number, grouping=True) |
Conclusion
Understanding how to concatenate string and int in Python is a fundamental skill that opens up countless possibilities in data handling and presentation. Whether you are a beginner or an experienced coder, mastering this simple yet powerful technique is a step forward in your Python journey. Remember, the right method depends on your specific needs and the context of your project.
FAQ
What happens if I try to concatenate a string and int without converting the int?
Python will throw a TypeError because it cannot directly concatenate different data types.
Can I concatenate other data types like lists or floats with strings?
Yes, but similar to integers, you need to convert them to strings first.
Is there a limit to how many items I can concatenate?
Practically, no. But remember, more items can lead to less readable code.
Are there any performance concerns with string concatenation?
For small to moderate concatenations, no. But for very large scale concatenations, consider using str.join() for better performance.
Can I use these methods in all versions of Python?
Most methods work across all versions, but f-strings are only available from Python 3.6 onwards.