String parsing is a fundamental skill in Python programming, essential for data processing, automation, and system integration tasks. In this guide, we will delve into the various methods and best practices on how to parse a string in Python, ensuring you can handle this crucial task with ease.
Before diving into the how-to, let’s clarify what we mean by string parsing in Python. String parsing involves analyzing a string and extracting specific information from it. This can range from simple data extraction to complex text processing.
Basic String Operations
Slicing
Slicing is a common string operation that allows you to extract specific portions of a string by specifying a range of indices. In Python, strings are indexed starting from 0, so the first character has an index of 0, the second has an index of 1, and so on. The syntax for slicing is as follows:
substring = example_string[start:end] |
- example_string: The string you want to slice;
- start: The index at which the slicing begins (inclusive);
- end: The index at which the slicing ends (exclusive).
For example:
text = “Hello, World!” result = text[7:12] # This will extract “World” |
Splitting
Splitting a string involves dividing it into multiple substrings based on a specified delimiter. Python provides a built-in method split() for this purpose. The syntax is as follows:
substring_list = example_string.split(delimiter) |
- example_string: The string you want to split;
- delimiter: The character or string at which the split occurs.
For example:
text = “apple,banana,cherry” fruits = text.split(‘,’) # fruits will be [‘apple’, ‘banana’, ‘cherry’] |
Replacing
Replacing a part of a string involves substituting one substring with another. Python provides the replace() method for this task. The syntax is as follows:
new_string = example_string.replace(old_substring, new_substring) |
- example_string: The string in which you want to perform the replacement;
- old_substring: The substring you want to replace;
- new_substring: The substring to replace old_substring with.
For example:
text = “I love ice cream.” new_text = text.replace(“ice cream”, “chocolate”) # new_text will be “I love chocolate.” |
Advanced String Parsing Techniques
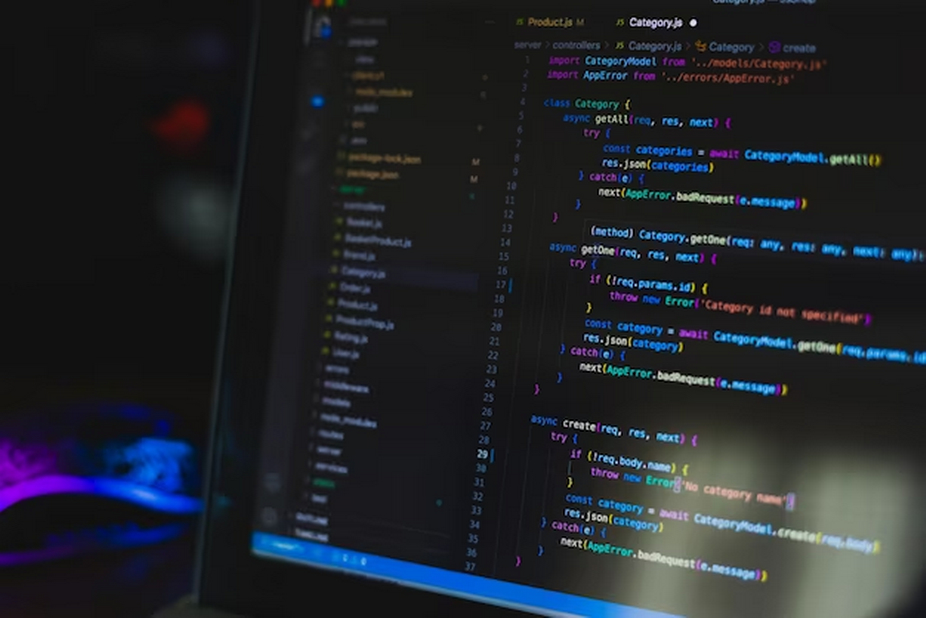
Regular Expressions (Regex)
Regular Expressions, often abbreviated as Regex, are a powerful tool for pattern matching and manipulation of text. Python’s re-module provides extensive support for working with regular expressions. With regular expressions, you can define complex patterns and search for or manipulate text that matches those patterns.
Here is a simple example of using regular expressions to find all email addresses in a text:
import re text = “My email addresses are [email protected] and [email protected]” email_addresses = re.findall(r’\S+@\S+’, text) # email_addresses will be [‘[email protected]’, ‘[email protected]’] |
String Functions
Python offers several built-in string functions that can be handy for various string parsing tasks. Some commonly used functions include:
- str.find(substring): Returns the index of the first occurrence of substring in the string, or -1 if not found;
- str.isdigit(): Checks if all characters in the string are digits and returns True or False accordingly;
- str.startswith(prefix): Checks if the string starts with the specified prefix and returns True or False.
These functions are useful for quickly performing specific checks or searches within a string.
Practical Applications: How to Parse a String in Python
Parsing strings in Python is a fundamental skill for working with textual data. Let’s explore practical applications and methods for parsing strings in Python, including using the split method, implementing regular expressions (regex), and leveraging Python’s built-in string methods.
Using Split Method
The split() method is a straightforward way to parse a string in Python, particularly useful for simple parsing tasks. It splits a string into substrings based on a specified delimiter, such as spaces by default. Let’s take an example of extracting words from a sentence:
python Copy code sentence = “Welcome to string parsing in Python” words = |
In this example, words will be a list containing individual words from the sentence. This method is ideal when the delimiter between elements in the string is consistent, like spaces between words.
Advantages | Limitations |
Easy to use for basic parsing tasks. | May not work well with complex delimiters or patterns. |
Suitable for splitting strings on common delimiters like spaces, commas, or tabs. | Doesn’t provide advanced pattern matching capabilities. |
Implementing Regex
For more complex parsing tasks, regular expressions (regex) are a powerful tool in Python. Regex allows you to define patterns and search for or manipulate text that matches those patterns. Let’s consider an example of extracting email addresses from a text:
import re text = “Contact us at [email protected]” email = re.findall(r’\S+@\S+’, text) |
In this code, we use the re.findall() function to find all email addresses in the text. The r’\S+@\S+’ regular expression pattern matches strings that resemble email addresses.
Advantages | Limitations |
Highly flexible and capable of handling complex patterns. | Regex can be challenging to master due to its complexity. |
Suitable for parsing structured data like emails, URLs. | It may not be the best choice for simple parsing tasks. |
String Methods
Python’s built-in string methods provide various ways to parse a string. These methods offer functionality for specific parsing tasks, making them a convenient choice for certain situations. Let’s look at an example of checking if a string contains a digit:
example_string = “Parse4me” contains_digit = any(char.isdigit() for char in example_string) |
In this code, we use a generator expression with the any() function to check if any character in the string is a digit.
Advantages | Limitations |
Convenient for specific parsing tasks like checking digits or letters. | Limited to specific parsing tasks. |
Typically easy to understand and use. | May require combining multiple methods for complex parsing needs. |
Best Practices in String Parsing
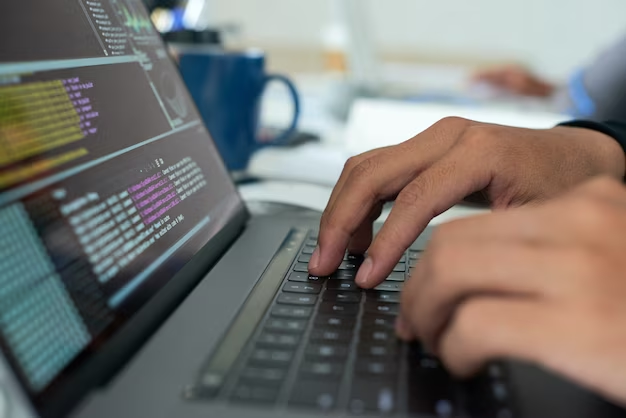
String parsing in Python is a crucial skill for extracting and manipulating information from text data. To ensure efficient and error-free string parsing, it’s essential to follow best practices. Let’s delve into these best practices, emphasizing the importance of understanding your data, leveraging Python libraries, and implementing error handling mechanisms.
Understand Your Data
Before embarking on any string parsing task, it’s imperative to thoroughly understand the structure and characteristics of the string you are working with. Consider the following aspects:
- Delimiters and Patterns: Identify the delimiters or patterns that separate different parts of the string. This step is crucial for choosing the appropriate parsing method. For example, if your data is comma-separated, the split() method might be suitable. For more complex patterns, regular expressions (regex) are a powerful choice;
- Data Types: Determine the types of data within the string. Are you dealing with numbers, dates, email addresses, or plain text? Knowing the data types will help you decide how to extract and handle specific elements correctly;
- Data Integrity: Check the data for consistency and potential errors. Ensure that the string adheres to the expected format. Handling unexpected variations in the data is a key consideration in robust string parsing.
Use Python Libraries
Python provides libraries and modules that simplify string parsing tasks. One of the most powerful tools for complex pattern matching is the re library, which enables you to work with regular expressions.
- Regular Expressions (Regex): Regular expressions are a versatile way to define and search for patterns in text. They allow you to create complex rules for matching and extracting data from strings. For instance, you can use regex to validate email addresses, extract dates, or parse structured data.
Example of using regex to extract email addresses:
import re text = “Contact us at [email protected]” email = re.findall(r’\S+@\S+’, text) |
- String Methods: Python’s built-in string methods, such as split(), find(), and replace(), are handy for straightforward parsing tasks. These methods are intuitive and can be highly effective when you need to perform basic operations on a string.
Error Handling
String parsing is prone to errors, especially when dealing with unstructured or inconsistent data. To ensure the robustness of your code, implement error handling mechanisms using try-except blocks. Common exceptions that can occur during string parsing include IndexError, ValueError, or AttributeError.
Example of error handling when using the split() method:
try: parts = string.split(‘,’) except ValueError as e: print(f”An error occurred: {e}”) # Handle the error gracefully |
Error handling not only prevents your code from crashing but also allows you to provide meaningful feedback or fallback mechanisms when parsing fails.
Conclusion
Mastering how to parse a string in Python is an invaluable skill in your programming toolkit. Whether you’re a beginner or looking to refine your skills, understanding and applying these methods will enhance your ability to handle string data efficiently.
FAQ
Q1: How do I parse a JSON string in Python?
A1: Use the json.loads() method to convert a JSON string into a Python dictionary.
Q2: Can I parse XML and HTML strings in Python?
A2: Yes, libraries like BeautifulSoup and lxml are great for parsing XML and HTML.
Q3: How do I handle Unicode strings in Python parsing?
A3: Python 3 handles Unicode by default. Use .encode() and .decode() methods for specific encodings.
Q4: Is it possible to parse a string into a date in Python?
A4: Yes, use the datetime.strptime() method to convert strings into datetime objects.