Python, which is a multifaceted programming language, provides different ways of representing and utilizing mathematical constants and expressions. This mathematical constant is called either ‘e’ or Euler’s number. This article is focused on Python to walk us through writing ‘e’ in different contexts in a precise manner so that a novice can understand as well.
Understanding ‘e’ in Mathematical Context
It is important to understand mathematical ‘e’ significance before delving into its representation in Python. The base ‘e’ (approximately 2.71828) which is present in all natural logarithms occupies a special place and is used in different areas of mathematics such as calculus, complex analysis, and financial calculations.
In Python, correctly representing ‘e’ is crucial because it supports the precision in calculations. Hence it is imperative to know how to write ‘e’ in Python for different mathematical expressions. We shall get acquainted with the fundamentals of representing ‘e’ in Python.
Basic Representation of ‘e’ in Python
One of the simplest ways to write ‘e’ in Python is to import the math module. In this module for instance, you can access several mathematical functions and constants like ‘e’.Below is a demonstration of how to represent ‘e’ in Python using the math module:
import math
# Accessing 'e' in Python
e_constant = math.e
print(e_constant)
This code snippet is importing the math module and assigning the value of ‘e’ to e_constant. The end it prints the value of ‘e’ to the console, which is very precise.
The import of the math module is the basic step in the sequence of operations of using Python for a series of computations where ‘e’ is involved with great precision.
Benefits of Using ‘e’ in Python
By learning how to use `e` in Python, a way which allows the use of mathematical programming is opened. Here are some benefits:
- Accurate Calculations: The use of ‘e’ provides the correlation for accurate computations especially in situations of exponential behavior;
- Advanced Mathematical Operations: ‘e’ plays an instrumental role in higher level operations, such as differentiation and integration, especially in the scope of calculus which is an advanced math subject;
- Financial Applications: Finance employs ‘e’ as a fundamental tool for continuous compounding and rate computation, implying that it is required in financial programming;
- Statistical Analysis: In statistics as well, letter ‘e’ occurs much in equations of probability and regression analysis enabling a lot for robust statistical programming in Python.
Calculating Exponentials with ‘e’ in Python
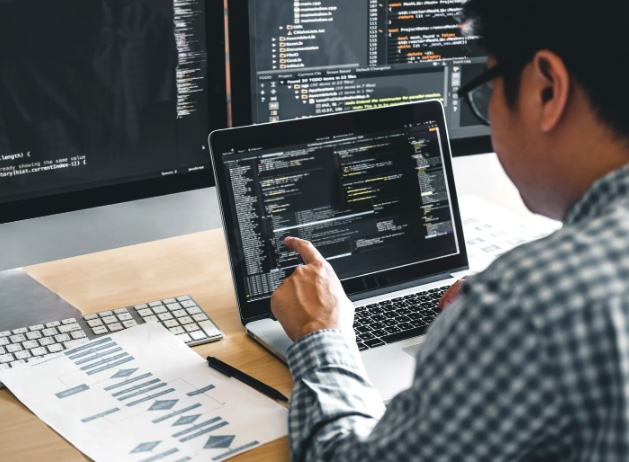
Application of the mathematical constant e as one of the fundamentals in Python is its use in exponential calculations. In fact, math.exp(x) is a very useful tool for calculating e^x where e is the base and x is the exponent. With it we can easily and quickly execute exponential operations. Let’s move on and talk about adding ‘e’ in Python to deal with exponential functions.
Exponential functions are among the most important functions, which are used in a great number of scientific and engineering calculations, describing, for example, exponential growth or decay. The mathematics.exp (x) function in Python is especially effective at handling such computations. This feature calculates e to the power of x simply and efficiently. Here’s an illustrative example demonstrating the utilization of ‘e’ in Python for exponential calculations:
import math
# Calculating e to the power of 2
result = math.exp(2)
print(result)
Here in this code snippet, math.exp(2) function performs the ‘e’ raised to the power of 2 calculation. The result is computed and subsequently stored in the variable result. With the help of the math module, Python guarantees the required level of accuracy and precision for exponential calculations. Lastly, the result is sent to the console from which the application of the ‘e’ constant in exponential operations in Python coding is demonstrated.
The ability to carry out very fast operations with ‘e’ in Python is a godsend in different fields such as physics, finance and statistics. From modeling population growth, to calculating compound interest, compound decay or anything exponential, The ‘e’ support in Python allows programmers to solve such complex problems with ease and accuracy.
Logarithmic Functions Involving ‘e’
Logarithmic functions along with exponential calculations are other important areas in which understanding the ‘e’ representation in Python is crucial. The most common logarithms are ‘e’, the base of natural logarithms, which is why it is crucial to use ‘e’ correctly in logarithmic calculations. The Python math module has the math.log(x) method that makes natural logarithm calculation with the base ‘e’ possible. Let’s discover how to use ” e ” in Python for logarithmic functions.
import math
# Calculating the natural logarithm of 10
log_result = math.log(10)
print(log_result)
The natural logarithm of 10 is being calculated in math.log(10) function with adopted ‘e’. Python includes ‘e’ in the standard library meaning that the logarithmic calculation is done correctly and in time. Later, ‘e’ best fit in the logarithmic functions in Python can be seen in the logarithmic value printed to the console.
Learning how to apply the form ‘e’ in Python for handling exponential and logarithmic operations broadens computational competencies for programmers to address many math tasks. The integration of ‘e’ into the field of application-oriented mathematics is made possible with the help of the math module of Python, therefore providing the capability to apply ‘e’ in many mathematical programming projects, thus enabling more accurate and faster calculations within many domains.
Advanced Usage of ‘e’ in Python
For advanced users seeking to harness the power of ‘e’ in Python, the numpy and scipy libraries provide extensive functionalities for performing complex calculations involving ‘e’. These libraries are widely utilized in scientific computing and offer an array of tools and functions tailored for advanced mathematical operations.
Using Numpy for ”e” in Python
The numpy library is a cornerstone of numerical computing in Python, renowned for its efficiency and versatility. It offers robust support for array operations, mathematical functions, and linear algebra routines, making it an ideal choice for performing advanced calculations involving ‘e’. Below are some key features of numpy related to ‘e’:
- Exponential Function: Numpy provides the numpy.exp() function, which efficiently computes ‘e’ raised to the power of a given array or scalar value. This function is particularly useful for performing element-wise exponential calculations across large datasets;
- Logarithmic Function: The numpy.log() function calculates the natural logarithm of an array or scalar value, with ‘e’ as the base. It offers enhanced flexibility and performance compared to native Python functions, especially when dealing with large datasets;
- Advanced Mathematical Operations: Numpy facilitates a wide range of advanced mathematical operations involving ‘e’, such as matrix exponentiation and eigenvalue calculations. These functionalities are essential for tasks ranging from signal processing to machine learning.
Leveraging scipy for ‘e’ in Python
The scipy library builds upon numpy’s foundation, providing additional capabilities for scientific computing and data analysis. It includes specialized submodules for optimization, interpolation, integration, and more, making it a comprehensive toolkit for tackling complex mathematical problems. Here’s how scipy enhances the utilization of ‘e’ in Python:
- Integration: Scipy’s integration subpackage offers functions for numerical integration, including methods for solving differential equations and computing definite integrals. ‘e’ often appears in the context of integrals, especially in physics and engineering applications;
- Statistical Functions: Scipy provides extensive support for statistical computations, including probability distributions, hypothesis testing, and descriptive statistics. Many statistical formulas and algorithms involve ‘e’, making scipy indispensable for statistical analysis in Python;
- Signal Processing: The signal processing submodule in scipy offers tools for filtering, Fourier analysis, and spectral analysis. ‘e’ frequently appears in the context of Fourier transforms and signal modulation, highlighting scipy’s relevance in digital signal processing tasks.
Conclusion
Understanding how to write ‘e’ in Python is a fundamental skill for anyone delving into mathematical, scientific, or financial programming using Python. From basic representations to complex calculations, Python provides various methods to work with this important mathematical constant. Whether you’re a beginner or an advanced user, mastering how to write ‘e’ in Python is a step forward in your coding journey.
FAQ
Yes, you can approximate ‘e’ manually, but for accuracy and simplicity, using the math module is recommended.
Python’s math.e provides a high degree of accuracy. For even more precision, consider using the decimal module.
Absolutely! Understanding how to write ‘e’ in Python is useful in compound interest calculations and other financial formulas.
Python’s cmath module, designed for complex numbers, can be used to include ‘e’ in such calculations.
Ensure you import the math module before using ‘e’. Also, be mindful of the difference between math.exp(x) and math.pow(math.e, x), as they serve similar but distinct purposes.