Python, a programming language known for its simplicity and readability, provides a seamless experience when it comes to performing operations like addition. In this comprehensive guide, we will dive into the fundamental skill of adding two variables in Python. Whether you are a beginner taking your first steps in Python or an experienced programmer looking for a refresher.
Understanding Variables
Before we delve into the nitty-gritty of adding two variables in Python, let’s establish the basics. Python’s elegance lies in its straightforward approach to programming, making tasks like addition intuitive and efficient.
In Python, a variable is essentially a storage location paired with an associated symbolic name. This name represents a known or unknown quantity of information referred to as a value. Variables serve as a fundamental building block in programming, allowing us to store, manipulate, and work with data efficiently.
The Simple Art of Addition in Python
Adding two variables in Python is a fundamental operation that forms the basis of more complex calculations. In this guide, we will explore the process of adding two variables step by step, emphasizing clarity and simplicity.
Defining the Variables
Firstly, we need to define two variables. Variables in Python are used to store various types of data, such as numbers, strings, lists, and more. For this example, we will work with numerical variables.
number1 = 5 number2 = 10 |
In the code above, we have defined two variables, number1 and number2, and assigned them the values 5 and 10, respectively. These variables can now be used in mathematical operations.
Performing Addition
To add two variables in Python, we use the + operator. This operator is not only used for numerical addition but can also concatenate strings and perform other operations depending on the data types involved. However, in this case, we are focusing on numerical addition.
result = number1 + number2 |
In this code, we have added number1 and number2 together and stored the result in a new variable called result. The + operator takes the values of number1 and number2, adds them together, and assigns the sum to result.
Displaying the Result
Now that we have performed the addition, it’s time to see the outcome. To display the result in Python, we can use the print function.
print(result) # Output: 15 |
The print function is a built-in Python function that allows us to output information to the console. In this case, we are printing the value of the result variable. When you run this code, it will display the sum of number1 and number2, which is 15, in the console.
Delving Deeper: Variable Types and Addition
Depending on the variable types involved, Python exhibits different behaviors when performing addition. In this educational guide, we will delve into three primary variable types: Numeric, String, and List, and explore how Python handles addition operations for each of them.
Numeric Variables
Numeric variables in Python encompass both integers and floating-point numbers. When you add two numeric variables, Python performs arithmetic addition, resulting in a numeric sum.
Consider the following example:
a = 20 b = 30.5 sum = a + b # 50.5 |
In this case, the variables a and b are of numeric types, specifically an integer and a float. When we add them together, the result is 50.5, a numeric sum.
String Variables
When you are dealing with string variables in Python, the addition operation takes on a different meaning. Instead of performing arithmetic addition, Python concatenates (joins together) the strings.
Here’s an example:
first_name = “John” last_name = “Doe” full_name = first_name + ” ” + last_name # John Doe |
In this scenario, first_name and last_name are both string variables. By using the + operator, Python combines these strings, resulting in the full name “John Doe.”
List Variables
List variables in Python contain collections of elements. When you add two list variables together, Python concatenates the lists, creating a single list containing all the elements from both lists.
Consider the following example:
list1 = [1, 2, 3] list2 = [4, 5, 6] combined_list = list1 + list2 # [1, 2, 3, 4, 5, 6] |
In this instance, list1 and list2 are both lists. When you use the + operator between them, Python merges the two lists, resulting in a combined list [1, 2, 3, 4, 5, 6].
To provide a concise overview of how Python handles addition for different variable types, let’s visualize the scenarios in a table format:
Variable Type 1 | Variable Type 2 | Result |
Integer | Integer | Numeric Sum |
Float | Integer | Numeric Sum |
String | String | Concatenation |
List | List | Concatenation |
In summary, it’s essential to remember the following key points when adding variables in Python:
- When adding two numeric variables (integers or floats), the result is a numeric sum;
- When adding two string variables, Python concatenates them, combining the strings;
- When adding two list variables, Python concatenates them, creating a single, concatenated list.
Handling Errors and Exceptions
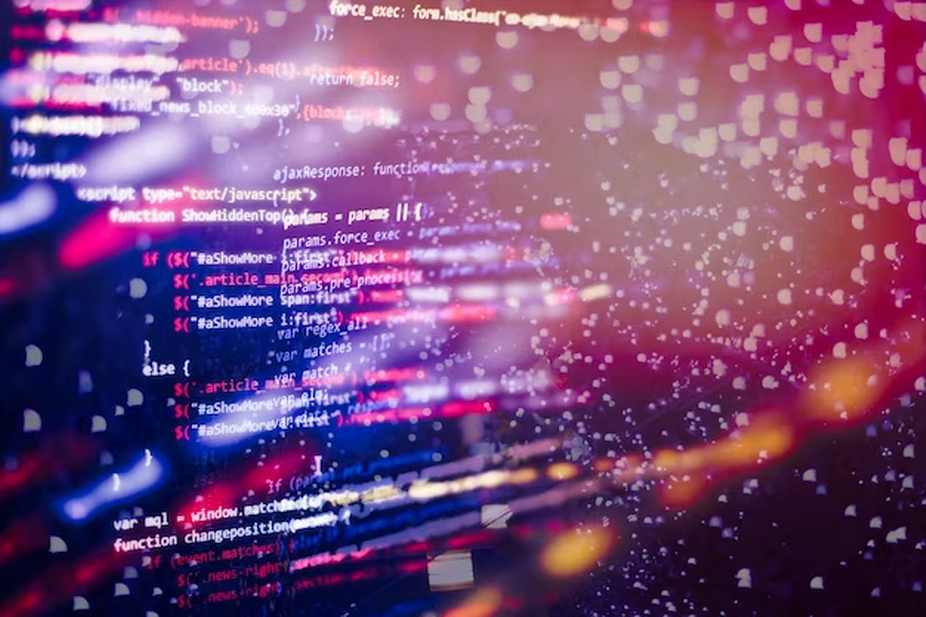
When you are learning how to add two variables in Python, you may encounter errors and exceptions, especially when dealing with variables of incompatible types. Understanding these common pitfalls and how to handle them is essential for writing robust and error-free Python code. Let’s explore common mistakes and how to address them when performing variable addition in Python.
Adding a String and a Number
One of the most frequent errors when adding variables in Python is attempting to add a string and a number. This operation results in a TypeError because Python does not implicitly convert types during addition. This means that you cannot directly combine a numeric variable (integer or float) with a string variable using the + operator.
ython Copy code number = 42 text = “Hello, “ result = text + number # This will r |
To resolve this issue, you need to explicitly convert the numeric variable to a string using functions like str() before concatenating:
number = 42 text = “Hello, “ result = text + str(number) # This works as expected |
Undefined Variables
Another common error to watch out for is referencing a variable that has not been defined yet. This leads to a NameError because Python cannot find the variable you are trying to use.
print(undefined_variable) # This will raise a NameError |
To avoid this error, make sure to define variables before using them:
defined_variable = “I’m defined!” print(defined_variable) # This works as expected |
Handling Exceptions
To handle errors and exceptions gracefully in Python, you can use try and except blocks. This allows you to anticipate potential issues and provide specific instructions for how your program should respond when errors occur.
Here’s an example of using a try and except block to handle a TypeError when adding variables of incompatible types:
try: number = 42 text = “Hello, “ result = text + number # Attempt to add string and number except TypeError as e: print(f”An error occurred: {e}”) # Handle the error gracefully here |
In this code, if a TypeError occurs during the addition, the program will catch the error, print a message, and allow you to implement a suitable response, such as informing the user or taking corrective action.
Similarly, you can use try and except blocks to handle NameError when dealing with undefined variables.
Conclusion
Understanding how to add two variables in Python is a fundamental skill in your programming journey. This operation, though simple, lays the groundwork for more complex operations and logic in Python programming. The elegance of Python lies in its simplicity, and as you’ve seen, adding variables is no exception.
Keep experimenting with different types of variables and operations to deepen your understanding of Python.
FAQ
Q: Can I add more than two variables in Python?
A: Absolutely! Python supports adding multiple variables in a single expression.
Q: What happens if I add two variables of different types?
A: Generally, Python will throw a TypeError, as it does not support implicit type conversion for addition.
Q: Is it possible to add variables of complex data types?
A: Yes, Python can handle complex data types, but the rules for addition depend on how these data types are defined.
Q: Can I use variables to store the result of an addition?
A: Yes, storing the result of an addition in a variable is a common and useful practice in Python.