Python, as a programming language, is renowned for its simplicity and readability, making it a favorite among beginners and experts alike. A common query among Python enthusiasts and learners is: what does ‘n’ mean in Python? This article aims to provide an insightful and detailed answer to this question, elucidating the different contexts in which ‘n’ is used in Python.
Understanding Variables and ‘n’
Before delving into the specifics of what ‘n’ means in Python, it’s crucial to comprehend the concept of variables. In Python, a variable is akin to a storage box where data values can be stored and manipulated. ‘n’ is a commonly used variable name, but it lacks a predefined meaning. Its significance is derived from how it’s employed within the code. For instance:
n = 5
Here, 'n' serves as a variable storing the integer value 5.
Variables in Python
Variables in Python are identifiers that hold values. They are essentially symbolic names that reference data. When you assign a value to a variable, you’re storing that value in memory, allowing you to retrieve and manipulate it later in the program.
‘n’ as a Variable
As mentioned earlier, ‘n’ is frequently used as a variable name in Python code. Its usage can vary greatly depending on the context of the program. Here are some common scenarios where ‘n’ might be employed:
- Loop Iterations: In loops, ‘n’ is often used as an iterator variable to control the number of iterations. For example, in a ‘for’ loop:
for n in range(5):
print(n)
In this loop, ‘n’ iterates over the range of numbers from 0 to 4.
- Mathematical Operations: ‘n’ can be used in mathematical operations or equations. For instance:
result = n * 2
In this case, ‘n’ is multiplied by 2, and the result is stored in the variable ‘result’.
- Function Arguments: ‘n’ may also appear as a parameter in function definitions or calls. For example:
def square(number):
return number ** 2
result = square(n)
Here, ‘n’ is passed as an argument to the function ‘square()’, which returns the square of ‘n’.
The ‘n’ in Loops
The utilization of ‘n’ in Python is prevalent, especially within loops such as for-loops and while-loops. In these contexts, ‘n’ typically symbolizes the number of iterations or serves as a counter. Consider the following example:
python
Copy code
for n in ran
In this loop, ‘n’ is employed to iterate through a sequence of numbers ranging from 0 to 9.
Understanding Loop Iteration
Loops in programming are constructs that allow a set of instructions to be executed repeatedly until a certain condition is met. They are invaluable for automating repetitive tasks and iterating over collections of data. In Python, loops come in various forms, but for-loops and while-loops are among the most commonly used.
The ‘range()’ Function
In the example provided, the ‘range()’ function is utilized to generate a sequence of numbers. This function generates numbers starting from 0 (by default) up to, but not including, the specified number. The general syntax of the ‘range()’ function is as follows:
range(start, stop, step)
Parameter | Description | Default Value |
---|---|---|
start | The starting value of the sequence (default is 0). | 0 |
stop | The end value of the sequence (not inclusive). | – |
step | The increment between each number in the sequence. | 1 |
Utilizing ‘n’ as an Iterator
Within the context of the loop:
for n in range(10):
print(n)
Variable | Description |
---|---|
n | Serves as the iterator variable that takes on each value in the sequence generated by ‘range(10)’. |
Loop | Iterates through each value of ‘n’ from 0 to 9. |
Action | During each iteration, the value of ‘n’ is printed to the console. |
‘n’ in Functions
‘n’ serves various purposes, commonly acting as a parameter or argument. Its function is to receive and store the value passed to the function for further processing. Let’s examine the role of ‘n’ in functions through the following example:
def square(n):
return n * n
In this function, ‘n’ is designated as a parameter, accepting a value that will subsequently be squared.
Understanding Functions in Python
Functions in Python are reusable blocks of code that perform a specific task. They allow for modularization of code, making it easier to read, write, and maintain. Functions can accept inputs, perform operations on those inputs, and optionally return an output.
‘n’ as a Parameter
In the function definition:
def square(n):
return n * n
- ‘n’ is declared as a parameter inside the parentheses following the function name;
- Parameters act as placeholders for values that will be supplied when the function is called;
- Here, ‘n’ represents the value that will be squared by the function.
Passing Arguments to Functions
When calling the function ‘square()’, you provide an argument that will be assigned to the parameter ‘n’:
result = square(5)
- In this example, the argument 5 is passed to the function ‘square()’;
- The value of ‘n’ within the function will be 5 during this invocation;
- The function will then return the square of 5, which is 25.
Benefits of Parameterization
Using parameters like ‘n’ allows functions to be flexible and reusable. By accepting inputs, functions can perform the same operation on different values, enhancing code modularity and readability.
The Mathematical ‘n’
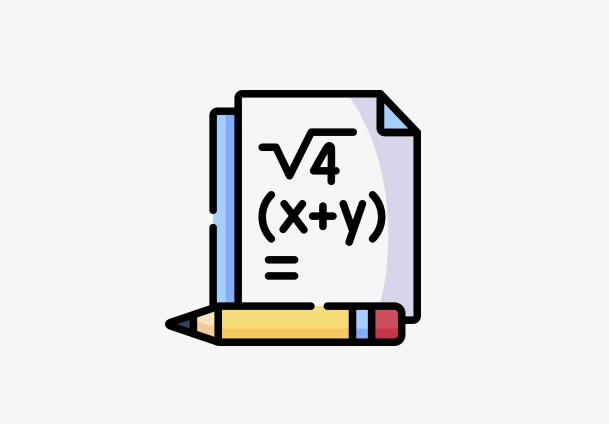
The variable ‘n’ holds significant importance in mathematical contexts, often serving as a placeholder for numerical values, particularly in algorithms, equations, and data structures. Understanding the role of ‘n’ is fundamental for efficiently solving problems and optimizing code. Let’s delve into the various applications and significance of ‘n’ in Python.
Representing Numerical Values
In mathematical algorithms and formulas, ‘n’ commonly represents a numerical value. This value can denote various aspects of the problem being solved. For instance:
- ‘n’ can signify the size of a data set, such as the number of elements in a list, array, or matrix;
- It may represent the number of iterations in a loop or the number of elements to process.
Applications in Algorithms
The variable ‘n’ frequently appears in algorithmic analysis, where it denotes the input size or the number of elements being processed. Understanding how ‘n’ affects algorithmic complexity is crucial for assessing performance and scalability. Common algorithmic complexities include:
- O(n): Linear time complexity, indicating that the runtime grows linearly with the size of ‘n’;
- O(n^2): Quadratic time complexity, where the runtime grows quadratically with ‘n’;
- O(log n): Logarithmic time complexity, characteristic of efficient algorithms like binary search.
Usage in Data Structures
In Python, data structures play a vital role in organizing and manipulating data efficiently. The variable ‘n’ often appears in discussions related to data structure operations and performance. Some examples include:
- Lists: The length of a list is commonly denoted as ‘n’, representing the number of elements;
- Arrays: In numerical computing, ‘n’ can denote the size of an array, determining its dimensions and memory requirements;
- Trees: In tree-based data structures such as binary search trees or heaps, ‘n’ signifies the number of nodes.
Impact on Computational Complexity
Understanding the relationship between ‘n’ and computational complexity is essential for designing efficient algorithms and writing optimized code. As ‘n’ grows, the efficiency of algorithms and data structures becomes increasingly critical. Optimizing algorithms and data structures to minimize the impact of ‘n’ can lead to significant performance improvements, especially for large datasets or computational tasks.
Example: Calculating Factorial
Consider the calculation of factorial as an example:
def factorial(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
n = 5
print("Factorial of", n, "is", factorial(n))
In this example, ‘n’ represents the input value for which the factorial is calculated. The loop iterates ‘n’ times, demonstrating the usage of ‘n’ as a control variable.
The Placeholder ‘n’
The placeholder ‘n’ holds significance as a versatile symbol often utilized during code development. While ‘n’ itself may lack specificity, it serves as a temporary placeholder until a more descriptive variable name is determined. This convention is particularly common in mathematical contexts, where ‘n’ typically represents a numerical value. Let’s explore the multifaceted role of the placeholder ‘n’ in Python coding practices.
Temporary Placeholder
During the initial stages of code development, developers frequently employ ‘n’ as a placeholder to denote numerical values or iteration counts. This temporary usage allows for rapid prototyping and algorithmic design without getting bogged down by naming intricacies. For example:
# Calculate the sum of the first n natural numbers
def sum_of_natural_numbers(n):
total = 0
for i in range(1, n + 1):
total += i
return total
In this snippet, ‘n’ acts as a placeholder for the number of natural numbers to be summed, offering a concise representation of the problem.
Iterative Control
In algorithms involving iteration, ‘n’ often serves as a control variable, determining the number of iterations or the size of the dataset being processed. This usage is particularly prevalent in loops and iterative constructs. For instance:
# Find the maximum value in a list
def find_max_value(lst):
max_val = float('-inf') # Initialize with negative infinity
for num in lst:
if num > max_val:
max_val = num
return max_val
In this example, ‘n’ is not explicitly used, but it indirectly represents the number of elements in the list ‘lst’, thereby influencing the number of iterations required.
Mathematical Representation
Mathematical formulas and equations often employ ‘n’ as a variable representing a numerical quantity. This convention facilitates the concise expression of mathematical concepts and algorithms. Consider the following example of calculating the factorial of ‘n’:
# Calculate the factorial of n
def factorial(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
Here, ‘n’ symbolizes the input value for which the factorial is computed, aligning with its mathematical interpretation.
Flexibility and Readability
While ‘n’ lacks explicit semantic meaning, its usage as a placeholder promotes code flexibility and readability during the initial stages of development. Once the code’s functionality is established, developers often replace ‘n’ with more descriptive variable names, enhancing code comprehension and maintainability.
‘n’ in Data Structures
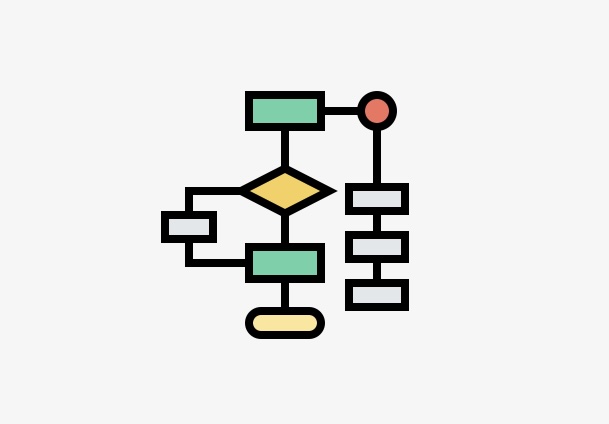
The symbol ‘n’ frequently assumes a crucial role in denoting the length or size of the structure. Understanding how ‘n’ is employed within data structures such as lists, tuples, and arrays is fundamental for effective data manipulation and algorithm design.
Representing Length or Size
In Python, the length or size of a data structure refers to the number of elements it contains. ‘n’ is commonly used as a placeholder to represent this quantity. For instance:
- In a list, ‘n’ signifies the number of elements present in the list;
- In a tuple, ‘n’ denotes the tuple’s size, which remains constant after creation;
- For arrays, ‘n’ indicates the total number of elements or the size of the array.
Accessing Length Information
Accessing the length of a data structure is typically achieved using built-in functions such as len(). For example:
my_list = [1, 2, 3, 4, 5]
n = len(my_list) # 'n' represents the length of the list
Here, the len() function returns the number of elements in the list my_list, which is then stored in the variable 'n'.
Utilizing ‘n’ in Algorithms
The knowledge of ‘n’ as the length or size of a data structure is invaluable in algorithm design and optimization. Algorithms often need to iterate through data structures or perform operations based on their size. ‘n’ provides a concise representation of the input size, facilitating algorithmic analysis and complexity estimation.
Example: Iterating Through a List
Consider an example where ‘n’ is used to iterate through a list and perform some operation on each element:
def process_list(lst):
for i in range(len(lst)):
print("Element at index", i, ":", lst[i])
my_list = [10, 20, 30, 40, 50]
process_list(my_list)
In this example, ‘n’ represents the length of the list my_list, determining the range of indices to iterate through during the processing of each element.
Impact on Computational Complexity
Understanding the relationship between ‘n’ and computational complexity is vital for designing efficient algorithms. Algorithms that exhibit linear time complexity, denoted as O(n), often have runtime proportional to ‘n’ due to the need to process each element individually. As ‘n’ grows, the efficiency of algorithms becomes increasingly critical.
Conclusion
In Python, the meaning of ‘n’ is context-dependent. It’s a simple yet flexible variable name that can represent various concepts, from loop counters to parameters in functions. Understanding what does n mean in Python is a step forward in grasping Python’s straightforward and efficient coding philosophy. Whether you’re a beginner or an experienced programmer, knowing the significance of ‘n’ in different scenarios will enhance your coding skills and deepen your understanding of Python.
Whether used in loops, functions, or as a mere placeholder, ‘n’ exemplifies Python’s user-friendly approach to coding. Remember, in Python, the meaning of ‘n’ is all about the context in which it’s used. Keep exploring Python and its nuances, and you’ll soon appreciate the simplicity and power this language has to offer.
FAQ
No, ‘n’ is not a special keyword in Python. It’s a variable name that can be replaced with any valid identifier.
Yes, ‘n’ can store any numeric value, including negative numbers, when used as a variable.
No, the choice of ‘n’ as a variable name is arbitrary. Any valid identifier can be used.
While ‘n’ is commonly used, especially in examples and tutorials, it’s best practice to use descriptive variable names for clarity.
Yes, ‘n’ can be used as a function name, but it’s not recommended due to its generality.